In this Qt5 GUI article iam going to show you How To Create QPrintDialog
The QPrintDialog class provides a dialog for specifying the printer’s configuration.
The dialog allows users to change document-related settings, such as the paper size and orientation, type of print (color or grayscale),
range of pages, and number of copies to print.
Controls are also provided to enable users to choose from the printers available, including any configured network printers.
Typically, QPrintDialog objects are constructed with a QPrinter object, and executed using the exec() function.
Check Qt5 C++ GUI Development Articles with videos training and source codes.
1: Qt5 C++ Introduction And Installation
2: Qt5 C++ First Console Application
3: Qt5 C++ First GUI Application
4: Qt5 C++ Signal And Slots Introduction
6: Qt5 C++ Creating Qt Style Sheets
7: Qt5 C++ Creating QPushButton
8: How To Create QCheckBox in Qt5
9: Qt5 GUI How To Create QRadioButton
10: Qt5 GUI Development How To Create ComboBox
11: Qt5 C++ GUI Development Creating QListWidget
12: Qt5 C++ GUI Development Creating QMessageBox
13 : Qt5 C++ GUI Creating QMenu And QToolbar
OK now iam going to use my previous article example on How To Create QMenu, and iam going to add a new menu item at name of print like this.
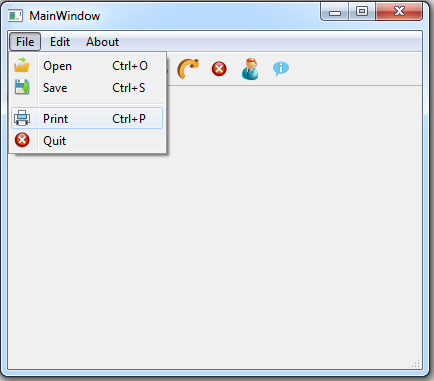
So after that you need to open your .pro file and add print support like this
1 |
QT += core printsupport |
After adding your .pro file will look like this
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17 18 19 20 21 22 23 24 |
QT += core gui QT += core printsupport greaterThan(QT_MAJOR_VERSION, 4): QT += widgets TARGET = QMenu TEMPLATE = app SOURCES += main.cpp\ mainwindow.cpp HEADERS += mainwindow.h FORMS += mainwindow.ui RESOURCES += \ res.qrc DISTFILES += \ print.png |
OK now right click on your menu item after that choose Go To Slot and from the dialog choose triggered() like this.
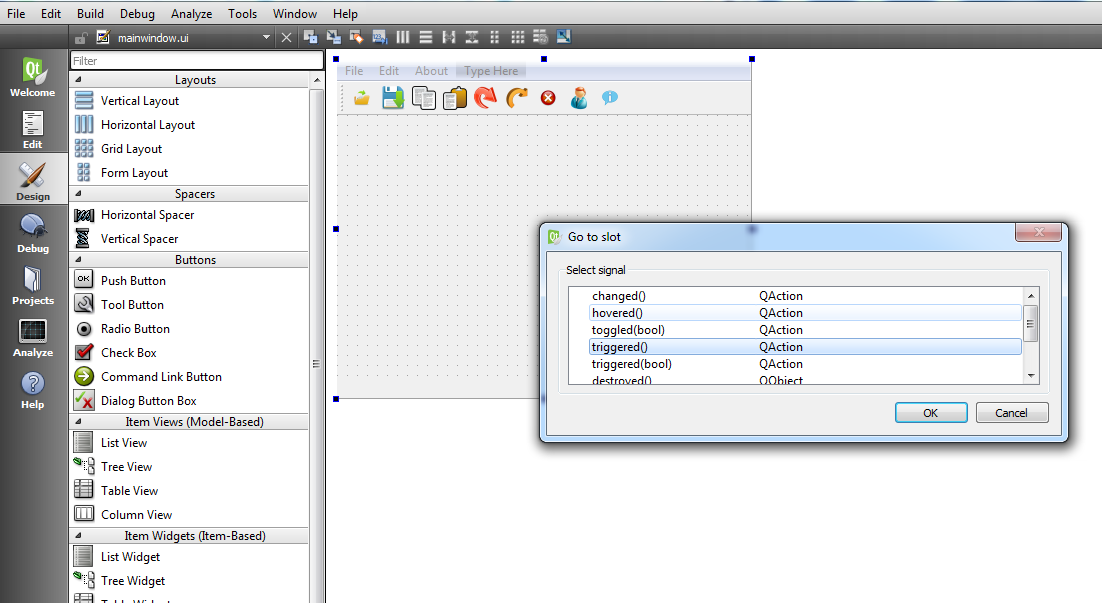
Also in your mainwindow.ui we need to add a QTextEdit like this
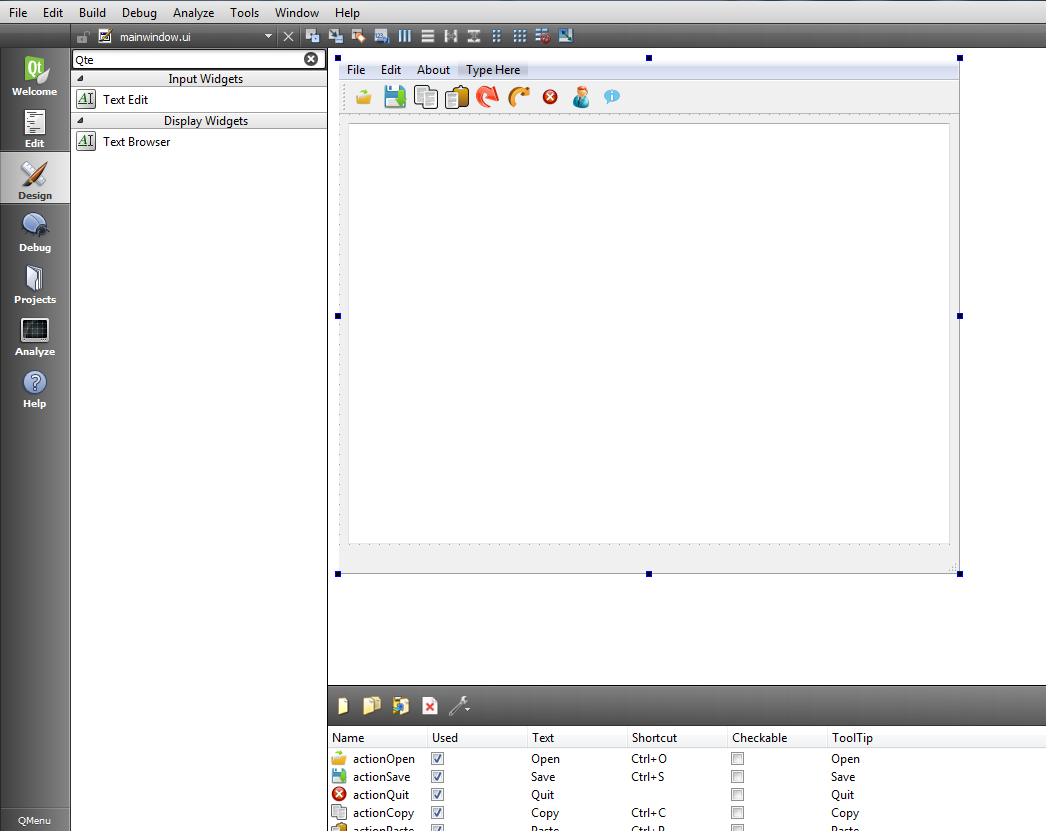
After that open mainwindow.cpp and in the slot section you need to add this line of code for QPrintDialog.
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17 18 19 |
void MainWindow::on_actionPrint_triggered() { QPrinter printer; QPrintDialog dialog(&printer, this); dialog.setWindowTitle("Print Document"); if(ui->textEdit->textCursor().hasSelection()) dialog.addEnabledOption(QAbstractPrintDialog::PrintSelection); if(dialog.exec() != QDialog::Accepted) return; } |
So your mainwindow.cpp look like this
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17 18 19 20 21 22 23 24 25 26 27 28 29 30 31 32 33 34 35 36 |
#include "mainwindow.h" #include "ui_mainwindow.h" #include<QPrinter> #include<QPrintDialog> MainWindow::MainWindow(QWidget *parent) : QMainWindow(parent), ui(new Ui::MainWindow) { ui->setupUi(this); } MainWindow::~MainWindow() { delete ui; } void MainWindow::on_actionPrint_triggered() { QPrinter printer; QPrintDialog dialog(&printer, this); dialog.setWindowTitle("Print Document"); if(ui->textEdit->textCursor().hasSelection()) dialog.addEnabledOption(QAbstractPrintDialog::PrintSelection); if(dialog.exec() != QDialog::Accepted) return; } |
This is the result
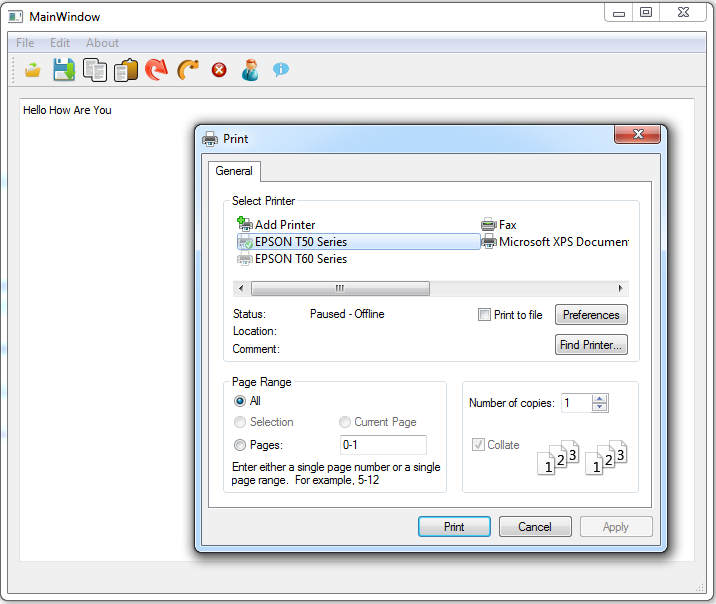
Also you can watch the complete video for this article
Subscribe and Get Free Video Courses & Articles in your Email