How to Create Animation in Qt5 with QPropertyAnimation – In this example, we will learn how to animate our Graphical
User Interface (GUI) elements using Qt’s property animation class, part of its powerful animation framework, which allows
us to create fluid looking animation with minimal effort.
So in this example we are going to animate a QPushButton widget, and in the design
we need to add a QPushButton in mainwindow.ui file like this.
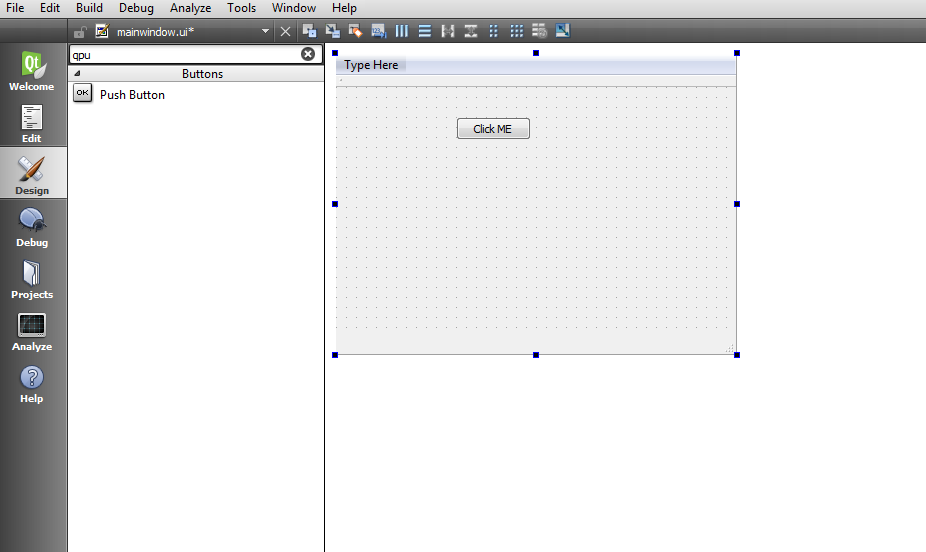
So now open your mainwindow.h and add the QPropertyAnimation header file, also you need to create a pointer to QPropertyAnimation
in the private section of your mainwindow.h like this.
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17 18 19 20 21 22 23 24 25 26 |
#ifndef MAINWINDOW_H #define MAINWINDOW_H #include <QMainWindow> #include<QPropertyAnimation> namespace Ui { class MainWindow; } class MainWindow : public QMainWindow { Q_OBJECT public: explicit MainWindow(QWidget *parent = 0); ~MainWindow(); private: Ui::MainWindow *ui; QPropertyAnimation *animation; }; #endif // MAINWINDOW_H |
After that open your mainwindow.cpp and add these codes in the constructor
1 2 3 4 5 |
animation = new QPropertyAnimation(ui->pushButton, "geometry"); animation->setDuration(10000); animation->setStartValue(ui->pushButton->geometry()); animation->setEndValue(QRect(200,200,100,50)); animation->start(); |
Now your mainwindow.cpp will look like this
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17 18 19 20 21 |
#include "mainwindow.h" #include "ui_mainwindow.h" MainWindow::MainWindow(QWidget *parent) : QMainWindow(parent), ui(new Ui::MainWindow) { ui->setupUi(this); animation = new QPropertyAnimation(ui->pushButton, "geometry"); animation->setDuration(10000); animation->setStartValue(ui->pushButton->geometry()); animation->setEndValue(QRect(200,200,100,50)); animation->start(); } MainWindow::~MainWindow() { delete ui; } |
How it works ?
So one of the more common methods to animate a GUI element is through the property
animation class provided by Qt, known as the QPropertyAnimation class. This class is part of the animation framework and it makes use of the
timer system in Qt to change the properties of a GUI element over a given duration.
What we are trying to accomplish here is to animate the button from one position to
another, while at the same time we also enlarge the button size along the way.
By including the QPropertyAnimation header in our source code in Step 2, we will be able
to access the QPropertyAnimation class provided by Qt and make use of its functionalities.
The code in Step 3 basically creates a new property animation and applies it to the push
button we just created in Qt Designer. We specifically request the property animation class
changes the geometry properties of the push button and sets its duration to 1,0000
milliseconds (10 seconds).
Then, the start value of the animation is set to the initial geometry of the push button,
because obviously we want it to start from where we initially place the button in Qt
Designer. The end value is then set to what we want it to become; in this case we will move
the button to a new position at x: 200, y: 200 while changing its size to width: 100, height: 50
along the way.
After that, call animation->start() to start the animation.
Compile and run the project and now you should see the button start to move slowly across
the main window while expanding in size a bit at a time, until it reaches its destination. You
can change the animation duration and the target position and scale by altering the values in
the preceding code. It’s really that simple to animate a GUI element using Qt’s property
animation system!
After runing the project this will be the result
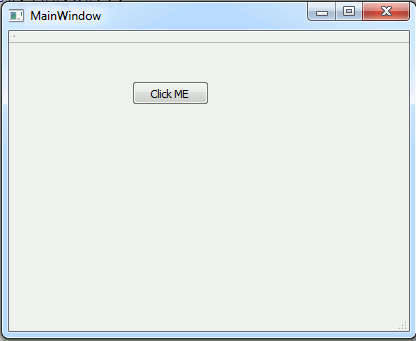
Also check Qt5 C++ GUI Development Articles in the below links
1: Qt5 C++ Introduction And Installation
2: Qt5 C++ First Console Application
3: Qt5 C++ First GUI Application
4: Qt5 C++ Signal And Slots Introduction
5: Qt5 C++ Layout Management
6: Qt5 C++ Creating Qt Style Sheets
7: Qt5 C++ Creating QPushButton
8: How To Create QCheckBox in Qt5
9: Qt5 GUI How To Create QRadioButton
10: Qt5 GUI Development How To Create ComboBox
11: Qt5 C++ GUI Development Creating QListWidget
12: Qt5 C++ GUI Development Creating QMessageBox
13 : Qt5 C++ GUI Creating QMenu And QToolbar
14: Qt5 C++ GUI Development Creating QPrintDialog
15: Qt5 C++ GUI Development Creating QFontDialog
16: Qt5 C++ GUI Development Creating QColorDialog
17: How to Create QFileDialog in Qt5 C++
18: How to Create QProgressbar in Qt5 C++
Also you can watch the complete video for this article
Subscribe and Get Free Video Courses & Articles in your Email