In this Qt5 GUI i want to show you How To Create QFontDialog.
The QFontDialog class provides a dialog widget for selecting a font. A font dialog is created through one of the static getFont() functions.
Example:
1 2 3 4 5 6 7 8 9 |
bool ok; QFont font = QFontDialog::getFont( &ok, QFont("Helvetica [Cronyx]", 10), this); if (ok) { // the user clicked OK and font is set to the font the user selected } else { // the user canceled the dialog; font is set to the initial // value, in this case Helvetica [Cronyx], 10 } |
Also the dialog can also be used to set a widget’s font directly:
1 |
myWidget.setFont(QFontDialog::getFont(0, myWidget.font())); |
Check Qt5 C++ GUI Development Articles in the below links
1: Qt5 C++ Introduction And Installation
2: Qt5 C++ First Console Application
3: Qt5 C++ First GUI Application
4: Qt5 C++ Signal And Slots Introduction
6: Qt5 C++ Creating Qt Style Sheets
7: Qt5 C++ Creating QPushButton
8: How To Create QCheckBox in Qt5
9: Qt5 GUI How To Create QRadioButton
10: Qt5 GUI Development How To Create ComboBox
11: Qt5 C++ GUI Development Creating QListWidget
12: Qt5 C++ GUI Development Creating QMessageBox
13 : Qt5 C++ GUI Creating QMenu And QToolbar
14: Qt5 C++ GUI Development Creating QPrintDialog
OK now iam going to use my previous article example on How To Create QMenu, also iam going to add a new Menu with a new Menu Item like this.
make sure that you have an icon for the menu item in your resource file
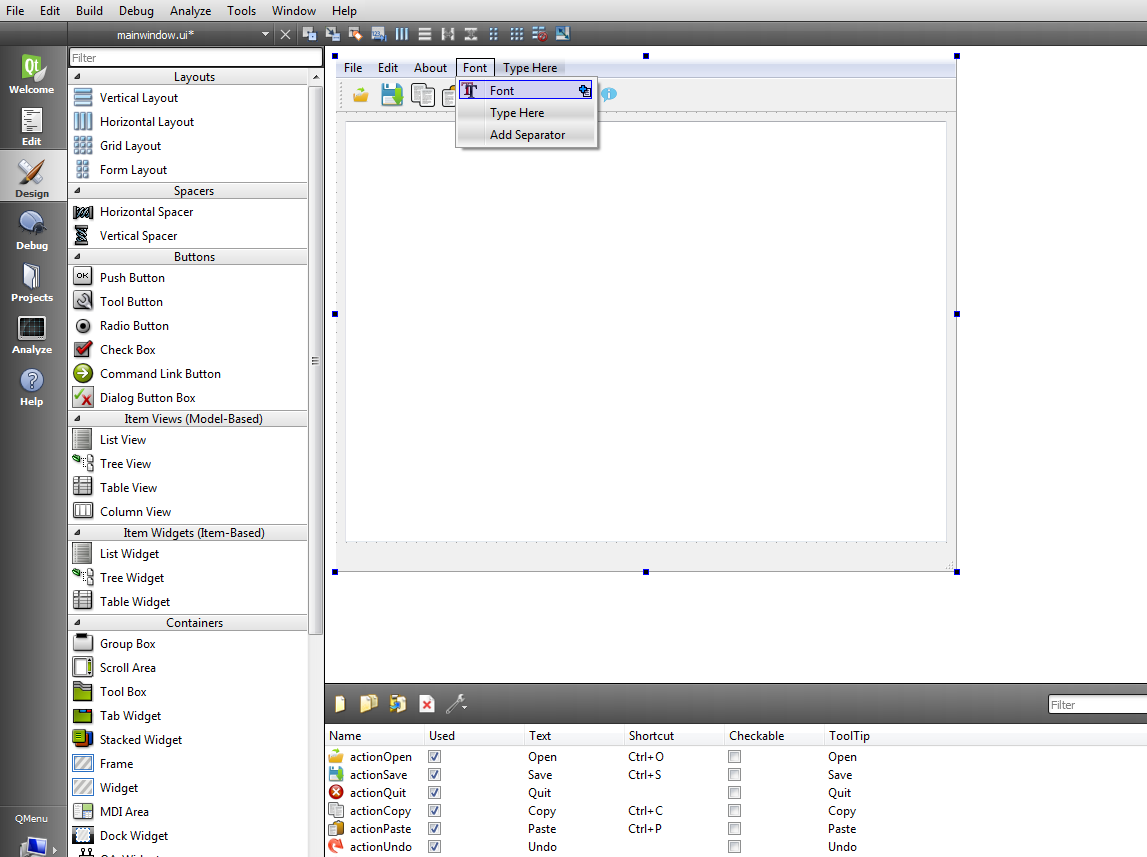
OK now right click on your menu item after that choose Go To Slot and from the dialog choose triggered() like this.
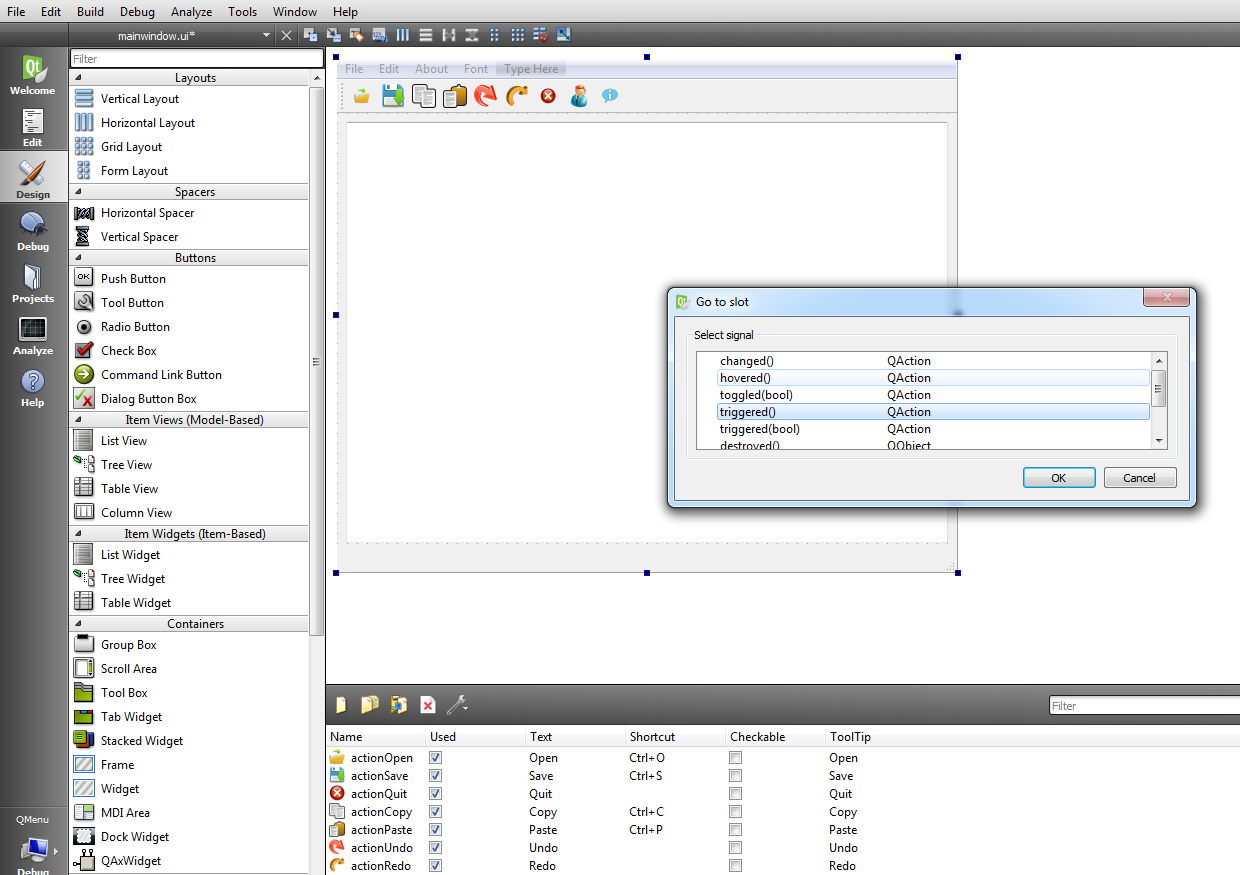
So now open your mainwindow.h file and in the header add QFontDialog class like this
1 |
#include<QFontDialog> |
After adding your mainwindow.h file will look like this
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17 18 19 20 21 22 23 24 25 26 27 28 29 |
#ifndef MAINWINDOW_H #define MAINWINDOW_H #include <QMainWindow> #include<QFontDialog> namespace Ui { class MainWindow; } class MainWindow : public QMainWindow { Q_OBJECT public: explicit MainWindow(QWidget *parent = 0); ~MainWindow(); private slots: void on_actionPrint_triggered(); void on_actionFont_triggered(); private: Ui::MainWindow *ui; }; #endif // MAINWINDOW_H |
Now open your mainwindow.cpp and these codes in the slot
1 2 3 4 5 6 7 8 9 10 |
bool ok; QFont font = QFontDialog::getFont(&ok, QFont("Helvetical[Cronyx]", 12), this); if(ok) { ui->textEdit->setFont(font); } |
After adding your mainwindow.cpp will look like this
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17 18 19 20 21 22 23 24 25 26 27 28 29 30 31 32 33 34 35 36 37 38 39 40 41 42 43 44 45 46 47 48 49 50 51 52 53 54 55 56 57 58 |
#include "mainwindow.h" #include "ui_mainwindow.h" #include<QPrinter> #include<QPrintDialog> MainWindow::MainWindow(QWidget *parent) : QMainWindow(parent), ui(new Ui::MainWindow) { ui->setupUi(this); } MainWindow::~MainWindow() { delete ui; } void MainWindow::on_actionPrint_triggered() { QPrinter printer; QPrintDialog dialog(&printer, this); dialog.setWindowTitle("Print Document"); if(ui->textEdit->textCursor().hasSelection()) dialog.addEnabledOption(QAbstractPrintDialog::PrintSelection); if(dialog.exec() != QDialog::Accepted) return; } void MainWindow::on_actionFont_triggered() { bool ok; QFont font = QFontDialog::getFont(&ok, QFont("Helvetical[Cronyx]", 12), this); if(ok) { ui->textEdit->setFont(font); } } |
And at the end this will be the result
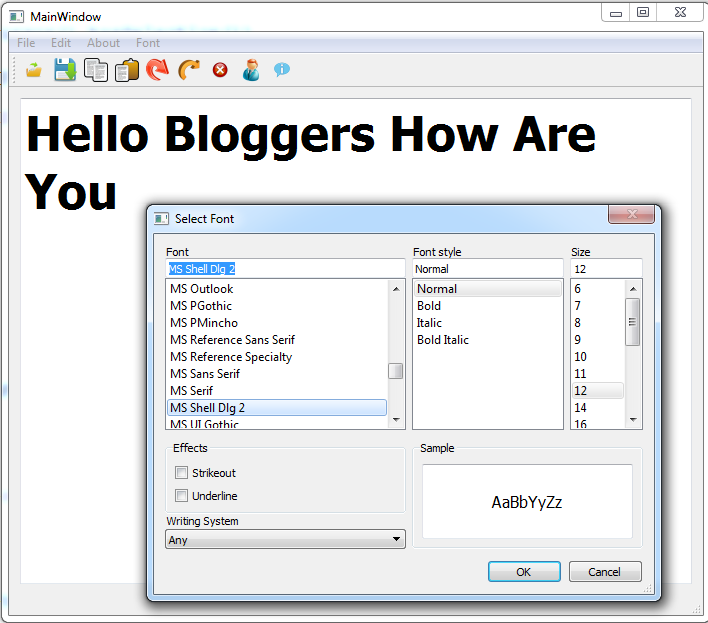
Also you can watch the complete video for this article
Subscribe and Get Free Video Courses & Articles in your Email