In this article we will learn How To Create QParallelAnimationGroup In Qt5 GUI, also we will learn how to use an animation group to manage the states of the animations contained in the group.
What is QParallelAnimationGroup In Qt5 ?
QParallelAnimationGroup–a container for animations–starts all its animations when it is started itself, i.e., runs all animations in parallel.
The animation group finishes when the longest lasting animation has finished.
You can treat QParallelAnimationGroup as any other QAbstractAnimation, e.g., pause, resume, or add it to other animation groups.
1 2 3 4 5 |
QParallelAnimationGroup *group = new QParallelAnimationGroup; group->addAnimation(anim1); group->addAnimation(anim2); group->start(); |
So first of all you need to create a New Project in Qt5 C++ Framework, after creating New Project you need to open your mainwindow.ui file and add three QPushButton in your design like this.
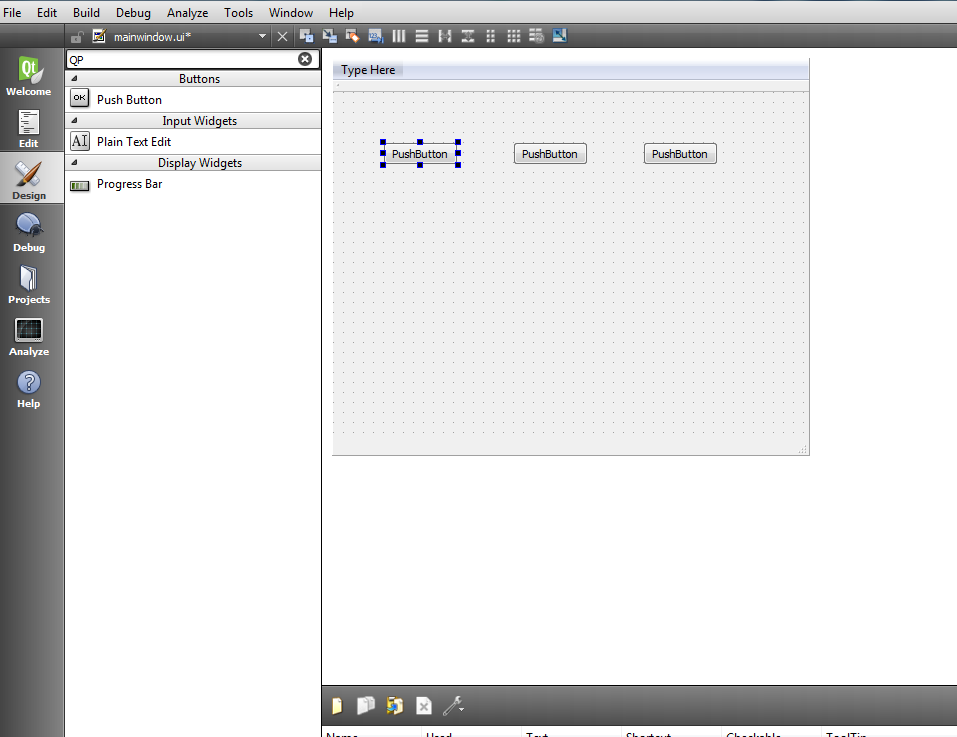
OK now you need to open your mainwindow.h file and add this
1 2 |
#include<QParallelAnimationGroup> #include<QPropertyAnimation> |
Also in the private section of your mainwindow.h we need to add these codes
1 2 3 4 5 6 7 |
QPropertyAnimation *animation1; QPropertyAnimation *animation2; QPropertyAnimation *animation3; QParallelAnimationGroup *animationGroup; |
After adding your mainwindow.h will look like this
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17 18 19 20 21 22 23 24 25 26 27 28 29 30 31 32 33 |
#ifndef MAINWINDOW_H #define MAINWINDOW_H #include <QMainWindow> #include<QParallelAnimationGroup> #include<QPropertyAnimation> namespace Ui { class MainWindow; } class MainWindow : public QMainWindow { Q_OBJECT public: explicit MainWindow(QWidget *parent = 0); ~MainWindow(); private: Ui::MainWindow *ui; QPropertyAnimation *animation1; QPropertyAnimation *animation2; QPropertyAnimation *animation3; QParallelAnimationGroup *animationGroup; }; #endif // MAINWINDOW_H |
So now open your mainwindow.cpp file and define the animation for each of the push buttons in the main window’s constructor.
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 |
animation1 = new QPropertyAnimation(ui->pushButton, "geometry"); animation1->setDuration(3000); animation1->setStartValue(ui->pushButton->geometry()); animation1->setEndValue(QRect(50, 200, 100, 50)); animation2 = new QPropertyAnimation(ui->pushButton_2, "geometry"); animation2->setDuration(3000); animation2->setStartValue(ui->pushButton_2->geometry()); animation2->setEndValue(QRect(150, 200, 100, 50)); animation3 = new QPropertyAnimation(ui->pushButton_3, "geometry"); animation3->setDuration(3000); animation3->setStartValue(ui->pushButton_3->geometry()); animation3->setEndValue(QRect(250, 200, 100, 50)); |
After that, create an easing curve and apply the same curve to all three animations
1 2 3 4 5 6 7 8 |
QEasingCurve curve; curve.setType(QEasingCurve::OutBounce); curve.setAmplitude(1.00); curve.setOvershoot(1.70); curve.setPeriod(0.30); animation1->setEasingCurve(curve); animation2->setEasingCurve(curve); animation3->setEasingCurve(curve); |
Once you have applied the easing curve to all three animations, we will then create an
animation group and add all three animations to the group:
1 2 3 4 5 |
animationGroup = new QParallelAnimationGroup; animationGroup->addAnimation(animation1); animationGroup->addAnimation(animation2); animationGroup->addAnimation(animation3); |
At the end call the start() function from the animation group we just created
1 |
animationGroup->start(); |
Your complete code in mainwindow.cpp constructor will look like this
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17 18 19 20 21 22 23 24 25 26 27 28 29 30 31 32 33 34 35 36 37 38 39 40 41 42 43 44 45 46 47 48 49 50 51 52 53 54 |
#include "mainwindow.h" #include "ui_mainwindow.h" MainWindow::MainWindow(QWidget *parent) : QMainWindow(parent), ui(new Ui::MainWindow) { ui->setupUi(this); animation1 = new QPropertyAnimation(ui->pushButton, "geometry"); animation1->setDuration(3000); animation1->setStartValue(ui->pushButton->geometry()); animation1->setEndValue(QRect(50, 200, 100, 50)); animation2 = new QPropertyAnimation(ui->pushButton_2, "geometry"); animation2->setDuration(3000); animation2->setStartValue(ui->pushButton_2->geometry()); animation2->setEndValue(QRect(150, 200, 100, 50)); animation3 = new QPropertyAnimation(ui->pushButton_3, "geometry"); animation3->setDuration(3000); animation3->setStartValue(ui->pushButton_3->geometry()); animation3->setEndValue(QRect(250, 200, 100, 50)); QEasingCurve curve; curve.setType(QEasingCurve::OutBounce); curve.setAmplitude(1.00); curve.setOvershoot(1.70); curve.setPeriod(0.30); animation1->setEasingCurve(curve); animation2->setEasingCurve(curve); animation3->setEasingCurve(curve); animationGroup = new QParallelAnimationGroup; animationGroup->addAnimation(animation1); animationGroup->addAnimation(animation2); animationGroup->addAnimation(animation3); animationGroup->start(); } MainWindow::~MainWindow() { delete ui; } |
How it Works
Since we are using an animation group now, we no longer call the start() function from the
individual animation, but instead we will be calling the start() function from the animation
group we just created.
If you compile and run the example now, you will see all three buttons being played at the
same time. This is because we are using the parallel animation group.
You can replace it with a sequential animation group and run the example again
1 |
QSequentialAnimationGroup *group = new QSequentialAnimationGroup; |
This time, only a single button will play its animation at a time, while the other buttons will
wait patiently for their turn to come.
The priority is set based on which animation is added to the animation group first. You can
change the animation sequence by simply rearranging the sequence of an animation being
added to the group. For example, if we want button 3 to start the animation first, followed
by button 2, and then button 1, the code will look like this:
1 2 3 |
group->addAnimation(animation3); group->addAnimation(animation2); group->addAnimation(animation1); |
Also check Qt5 C++ GUI Development Articles in the below links
1: Qt5 C++ Introduction And Installation
2: Qt5 C++ First Console Application
3: Qt5 C++ First GUI Application
4: Qt5 C++ Signal And Slots Introduction
5: Qt5 C++ Layout Management
6: Qt5 C++ Creating Qt Style Sheets
7: Qt5 C++ Creating QPushButton
8: How To Create QCheckBox in Qt5
9: Qt5 GUI How To Create QRadioButton
10: Qt5 GUI Development How To Create ComboBox
11: Qt5 C++ GUI Development Creating QListWidget
12: Qt5 C++ GUI Development Creating QMessageBox
13 : Qt5 C++ GUI Creating QMenu And QToolbar
14: Qt5 C++ GUI Development Creating QPrintDialog
15: Qt5 C++ GUI Development Creating QFontDialog
16: Qt5 C++ GUI Development Creating QColorDialog
17: How to Create QFileDialog in Qt5 C++
18: How to Create QProgressbar in Qt5 C++
19: How to Create QPropertyAnimation in Qt5
20: How To Control QPropertyAnimation in Qt5
So now run the complete project and you will see that three buttons are in one animation group
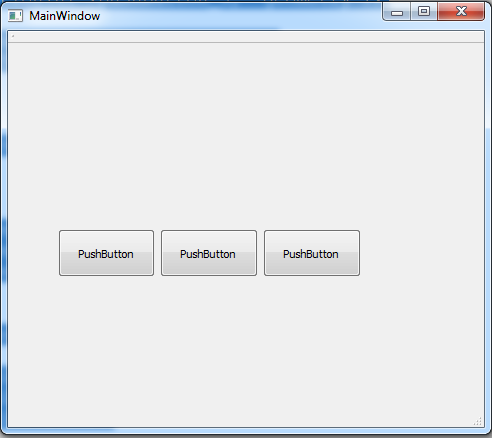
You can watch the complete video for QParallelAnimationGroup and QSequentialAnimationGroup
Subscribe and Get Free Video Courses & Articles in your Email