In this Qt5 article iam going to show How to Create QFileDialog in Qt5 GUI.
Check Qt5 C++ GUI Development Articles in the below links
1: Qt5 C++ Introduction And Installation
2: Qt5 C++ First Console Application
3: Qt5 C++ First GUI Application
4: Qt5 C++ Signal And Slots Introduction
5: Qt5 C++ Layout Management
6: Qt5 C++ Creating Qt Style Sheets
7: Qt5 C++ Creating QPushButton
8: How To Create QCheckBox in Qt5
9: Qt5 GUI How To Create QRadioButton
10: Qt5 GUI Development How To Create ComboBox
11: Qt5 C++ GUI Development Creating QListWidget
12: Qt5 C++ GUI Development Creating QMessageBox
13 : Qt5 C++ GUI Creating QMenu And QToolbar
14: Qt5 C++ GUI Development Creating QPrintDialog
15: Qt5 C++ GUI Development Creating QFontDialog
16: Qt5 C++ GUI Development Creating QColorDialog
so the QFileDialog class enables a user to traverse the file system in order to select one or many files or a directory.
the easiest way to create a QFileDialog is to use the static functions.
1 2 |
fileName = QFileDialog::getOpenFileName(this, tr("Open Image"), "/home/jana", tr("Image Files (*.png *.jpg *.bmp)")); |
In the above example, a modal QFileDialog is created using a static function. The dialog initially displays the contents of the “/home/jana” directory,
and displays files matching the patterns given in the string “Image Files (*.png *.jpg *.bmp)”. The parent of the file dialog is set to this, and
the window title is set to “Open Image”.
If you want to use multiple filters, separate each one with two semicolons. For example:
1 |
"Images (*.png *.xpm *.jpg);;Text files (*.txt);;XML files (*.xml)" |
Also yo can create your own QFileDialog without using the static functions. By calling setFileMode(), you can specify what the user must select in the dialog:
1 2 |
QFileDialog dialog(this); dialog.setFileMode(QFileDialog::AnyFile); |
OK now right click on your Open menu item in Signals And Slot Editor, after that choose Go To Slot and from the dialog choose triggered() like this.
iam using my previous article example How To Create QMenu.
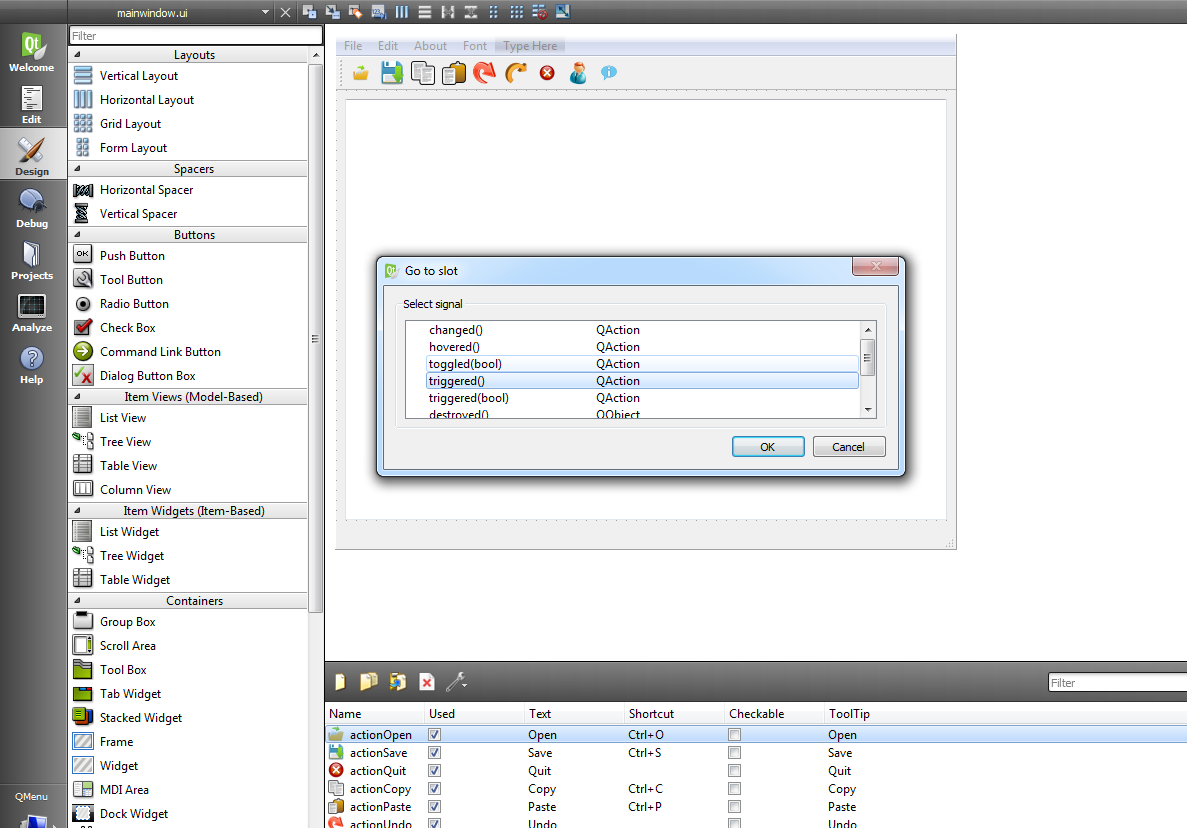
Also open your mainwindow.h and add QPrintDilaog header like this
1 |
#include<QFileDialog> |
After adding your mainwindow.h file will look like this
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17 18 19 20 21 22 23 24 25 26 27 28 29 30 31 32 33 34 35 |
#ifndef MAINWINDOW_H #define MAINWINDOW_H #include <QMainWindow> #include<QFontDialog> #include<QColorDialog> #include<QFileDialog> namespace Ui { class MainWindow; } class MainWindow : public QMainWindow { Q_OBJECT public: explicit MainWindow(QWidget *parent = 0); ~MainWindow(); private slots: void on_actionPrint_triggered(); void on_actionFont_triggered(); void on_actionColor_triggered(); void on_actionOpen_triggered(); private: Ui::MainWindow *ui; }; #endif // MAINWINDOW_H |
Now open your mainwindow.cpp and these codes in the slot
1 2 |
QString file = QFileDialog::getOpenFileName(this, "Open A File", "C://"); ui->textEdit->setText(file); |
After adding your mainwindow.cpp will look like this
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17 18 19 20 21 22 23 24 25 26 27 28 29 30 31 32 33 34 35 36 37 38 39 40 41 42 43 44 45 46 47 48 49 50 51 52 53 54 55 56 57 58 59 60 61 62 63 64 65 66 67 68 69 70 71 72 73 74 75 76 77 78 79 80 81 82 83 84 |
#include "mainwindow.h" #include "ui_mainwindow.h" #include<QPrinter> #include<QPrintDialog> MainWindow::MainWindow(QWidget *parent) : QMainWindow(parent), ui(new Ui::MainWindow) { ui->setupUi(this); } MainWindow::~MainWindow() { delete ui; } void MainWindow::on_actionPrint_triggered() { QPrinter printer; QPrintDialog dialog(&printer, this); dialog.setWindowTitle("Print Document"); if(ui->textEdit->textCursor().hasSelection()) dialog.addEnabledOption(QAbstractPrintDialog::PrintSelection); if(dialog.exec() != QDialog::Accepted) return; } void MainWindow::on_actionFont_triggered() { bool ok; QFont font = QFontDialog::getFont(&ok, QFont("Helvetical[Cronyx]", 12), this); if(ok) { ui->textEdit->setFont(font); } } void MainWindow::on_actionColor_triggered() { bool ok; QColor color = QColorDialog::getColor(Qt::yellow, this); if(ok) { ui->textEdit->setTextColor(color); } } void MainWindow::on_actionOpen_triggered() { QString file = QFileDialog::getOpenFileName(this, "Open A File", "C://"); ui->textEdit->setText(file); } |
Run the complete project and this will be the result
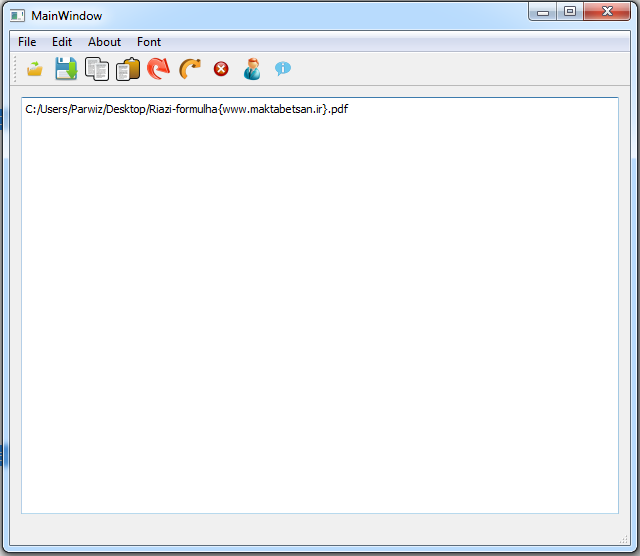
Also you can watch the complete video
Subscribe and Get Free Video Courses & Articles in your Email