This is our sixteenth article in Django, in this article we are going to learn Django Creating Forms with Django Model Form, as i have before said that there are two ways that you can create Forms in Django, the first way is that you can use Form class, we have already talked about this you can read this article for that Django Creating Forms with Form Class. and the second way is by using Model Form class, particularly in this article we are going to use Model Form Class.
Flask Web Development Tutorials
1: Flask CRUD Application with SQLAlchemy
2: Flask Creating News Web Application
3: Flask Creating REST API with Marshmallow
Python GUI Development Tutorials
1: PyQt5 GUI Development Tutorials
2: Pyside2 GUI Development Tutorials
3: wxPython GUI Development Tutorials
4: Kivy GUI Development Tutorials
5: TKinter GUI Development Tutorials
What is Model Form
If you’re building a database-driven app, chances are you’ll have forms that map closely to Django models. For instance, you might have a RegistrationData model, and you want to create a form that lets people register their information . In this case, it would be redundant to define the field types in your form, because you’ve already defined the fields in your model. now in the previous article when we have created our form using Form class, you have saw that we have created separate fields for our form.
For this reason, Django provides a helper class that lets you create a Form
class from a Django model.
So now we need to create a New Project in Django, also for django installation and creating New Project you can read this article Django Introduction & Installation. but you can use this command for creating of the New Project.
1 |
django-admin startproject DjangoProject |
After creating of the Django Project, you need to migrate your project. make sure that you have changed the directory to the created project.
1 |
python manage.py migrate |
So after doing this, let’s create our Django App, i have already talked about Django App in one of my articles, you can check this Django Apps & URL Routing.
1 |
python manage.py startapp MyApp |
After creation of the App, you need to add the created App in your settings.py file INSTALLED_APP section .
1 2 3 4 5 6 7 8 9 |
INSTALLED_APPS = [ 'django.contrib.admin', 'django.contrib.auth', 'django.contrib.contenttypes', 'django.contrib.sessions', 'django.contrib.messages', 'django.contrib.staticfiles', 'MyApp', ] |
Now open your models.py in your MyApp App, and add your model, this is a basic model with four fields, you can read my article on django models Django Models Introduction.
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 |
from django.db import models # Create your models here. class RegistrationData(models.Model): username = models.CharField(max_length=100) password = models.CharField(max_length=100) email = models.CharField(max_length=100) phone = models.CharField(max_length=100) def __str__(self): return self.username |
After adding a new model in Django, first you need to create migrations file and after that you need to add your model to the database, by default Django uses Sqlite database, for mysql database connection you can read this article Django Mysql Database Connection. make sure that you have changed directory to your project.
1 |
python manage.py makemigrations |
It is time to add our this model to the database, so for this you need to do migrate.
1 |
python manage.py migrate |
After this our model data has been successfully added to the Sqlite database.
OK now let’s just add our model to the Django admin panel, you can read this article for django super user Django Creating Super User. open your admin.py file and add this code.
1 2 3 4 5 6 |
from django.contrib import admin from .models import RegistrationData # Register your models here. admin.site.register(RegistrationData) |
It’s time to create our form, now you need to create a new Python file in your app, i have named forms.py, but you can name it what ever you want. and we are going to use Model Form in this article.
1 2 3 4 5 6 7 8 9 10 11 12 13 14 |
from django import forms from .models import RegistrationData class RegistrationModel(forms.ModelForm): class Meta: model = RegistrationData fields = [ 'username', 'password', 'email', 'phone', ] |
So you can see that in the code, we have extended our class from ModelForm, and after that in the inner Meta class we are going to tell django that according to our model create our form, for example in here we have RegisterationData model, so we are going to tell django that create our Form based on this model, also you need to specify the fields that you want form for that.
Before adding our html files, we need to create our views, so open your views.py file and add this code for creating a simple view. and you can see in the view we have passed the form as context variable to the django template. the register() view is for rendering our form, and the addUser() view is for adding data from our form to the database.
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17 18 19 20 21 22 23 |
from django.shortcuts import render, redirect from .forms import RegistrationModel # Create your views here. def register(request): context = {"form":RegistrationModel} return render(request, "index.html", context) def addUser(request): modelform = RegistrationModel(request.POST) if modelform.is_valid(): modelform.save() return redirect('register') |
Now we are going to render this form in our html file, so you need to create a new directory in your Django Project called templates, and in the templates folder add two html files, the first one is index.html and the second one is base.html. for django templates you can read this article Django Templates Introduction. also make sure that you have added your templates name in the templates section of settings.py file like this.
1 2 3 4 |
TEMPLATES = [ { 'BACKEND': 'django.template.backends.django.DjangoTemplates', 'DIRS': ["templates"], # templates name added here |
This is our base.html file, also you can see that in the base.html we have added the Bootstrap CDN link, because in this article we are going to use from Bootstrap styles. also you can check this article for adding styles to your Django Project Django Adding Bootstrap & CSS.
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17 18 19 |
<!DOCTYPE html> <html lang="en"> <head> <meta charset="UTF-8"> <!-- CSS only --> <link rel="stylesheet" href="https://stackpath.bootstrapcdn.com/bootstrap/4.5.0/css/bootstrap.min.css" integrity="sha384-9aIt2nRpC12Uk9gS9baDl411NQApFmC26EwAOH8WgZl5MYYxFfc+NcPb1dKGj7Sk" crossorigin="anonymous"> <title>{% block title %}{% endblock %}</title> </head> <body> {% block body %} {% endblock %} </body> </html> |
This is our index.html file and we are going to render our simple form in here. also we have added our the name of our addUser url in our form action.
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17 18 19 20 21 22 23 24 25 26 27 28 29 |
{% extends 'base.html' %} {% block title %} Registration {% endblock %} {% block body %} <div class="container"> <h1>Registration Model Form</h1> <h3>Welcome to codeloop.org</h3> <h5>Django Tutorial - Tutorial Number 16 Django Model Form</h5> <hr/> <form action="{% url 'addUser' %}" method="post"> {% csrf_token %} {{form.as_p}} <input type="submit" value="Submit" class="btn btn-primary"/> </form> </div> {% endblock %} |
Also you need to create your urls, you can read this article for url routing Django Apps & URL Routing . but just create a new python file in your MyApp app at name of urls.py and add these codes.
1 2 3 4 5 6 7 8 |
from django.urls import path from .views import register, addUser urlpatterns = [ path('', register, name = "register"), path('add/', addUser, name = "addUser"), ] |
And also you need to include your app urls.py in your project urls.py file.
1 2 3 4 5 6 7 8 |
from django.contrib import admin from django.urls import path, include urlpatterns = [ path('admin/', admin.site.urls), path('', include('MyApp.urls')) ] |
Now you can run your project and we have our simple form. also you can add data from this form to your database. so add some data and check your admin panel, you will have the added data.
1 |
python manage.py runserver |
http://localhost:8000/
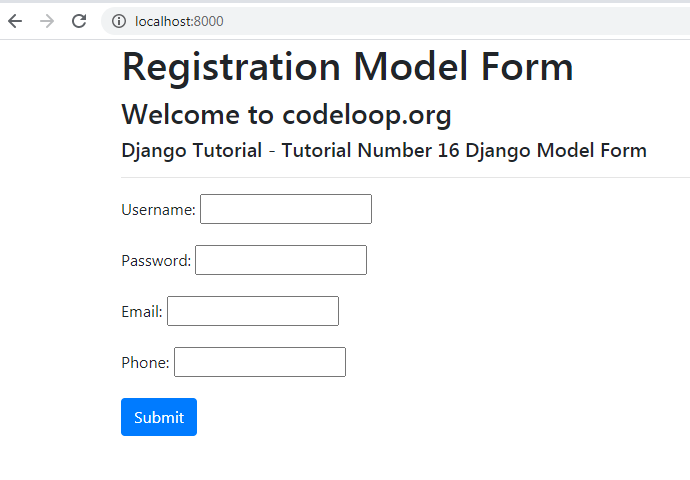
Adding Widgets To Model Form
Using widgets you can give design and style to your forms, so open your forms.py file and we need to bring some changes to our form, we are going to add a widgets dictionary to our inner class Meta.
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17 18 19 20 21 22 23 24 |
from django import forms from .models import RegistrationData class RegistrationModel(forms.ModelForm): class Meta: model = RegistrationData fields = [ 'username', 'password', 'email', 'phone', ] widgets = { 'username':forms.TextInput(attrs={'class':'form-control', 'placeholder':'Please Enter Username'}), 'password':forms.PasswordInput(attrs={'class':'form-control', 'placeholder':'Please Enter Password'}), 'email':forms.EmailInput(attrs={'class':'form-control', 'placeholder':'Please Enter Email'}), 'phone':forms.NumberInput(attrs={'class':'form-control', 'placeholder':'Please Enter Phone'}), } |
So now if you run your project and go to http://localhost:8000/, this will be the result a nice form with Django Model Form. it has the same functionality of our previous form, i mean that you can add data through this form to your database but just the design is changed.
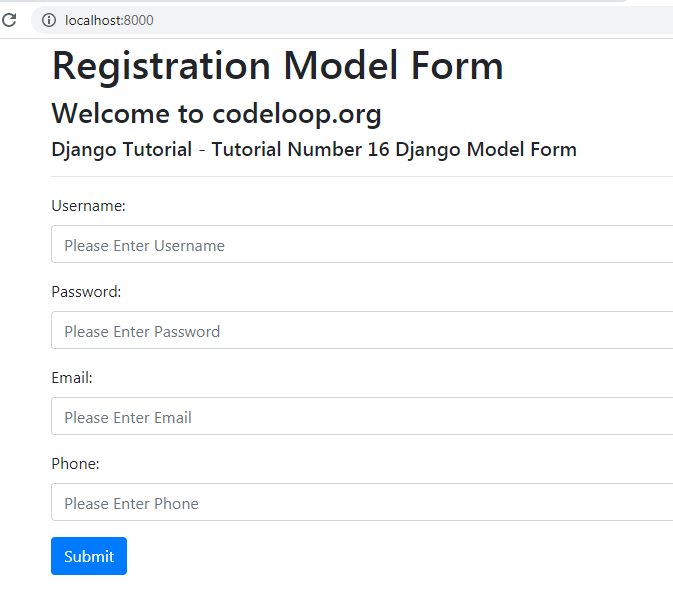
Subscribe and Get Free Video Courses & Articles in your Email