This is our nineteenth article in Django, in this article we are going to talk about Django Models Many-to-One Relationship . A many to one relationship implies that one model record can have many other model records associated with itself. To define a one to many relationship in Django models you use the ForeignKey data type on the model that has the many records .
Flask Web Development Tutorials
1: Flask CRUD Application with SQLAlchemy
2: Flask Creating News Web Application
3: Flask Creating REST API with Marshmallow
Python GUI Development Tutorials
1: PyQt5 GUI Development Tutorials
2: Pyside2 GUI Development Tutorials
3: wxPython GUI Development Tutorials
4: Kivy GUI Development Tutorials
5: TKinter GUI Development Tutorials
So now we need to create a New Project in Django, also for django installation and creating New Project you can read this article Django Introduction & Installation. but you can use this command for creating of the New Project.
1 |
django-admin startproject ModelRelationship |
After creating of the Django Project, you need to migrate your project. make sure that you have changed the directory to the created project.
1 |
python manage.py migrate |
So after doing this, let’s create our Django App, i have already talked about Django App in one of my articles, you can check this Django Apps & URL Routing.
1 |
python manage.py startapp MyApp |
After creation of the App, you need to add the created App in your settings.py file INSTALLED_APP section .
1 2 3 4 5 6 7 8 9 |
INSTALLED_APPS = [ 'django.contrib.admin', 'django.contrib.auth', 'django.contrib.contenttypes', 'django.contrib.sessions', 'django.contrib.messages', 'django.contrib.staticfiles', 'MyApp', ] |
Also we need to create a super user, because we will use the django admin panel in this article, you can use this command for creating of the super user.
1 |
python manage.py createsuperuser |
Now open your models.py in your MyApp App, and add your model, basically we are going to create two models, one for Category and the second one is for Article . and we want to use Django Models Many-to-One Relationship with our these two models.
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17 18 |
from django.db import models class Category(models.Model): name = models.CharField(max_length=30) def __str__(self): return self.name class Article(models.Model): title = models.CharField(max_length=30) description = models.CharField(max_length=100) category = models.ForeignKey(Category, on_delete=models.CASCADE) def __str__(self): return self.title |
The first Django model has the name field (e.g., Category instances can be Python, Django, Java, etc.). Next, the Article Django model, which has a category field, that itself has the models.ForeignKey(Category) definition. The models.ForeignKey() definition creates the one to many relationship, where the first argument Category indicates the relationship model.
After creating of the models, we need to migrate our project, first you need to do migrations and after that migrate.
1 |
python manage.py makemigrations |
After doing that, you will see this output in the terminal.
1 2 3 4 |
Migrations for 'MyApp': MyApp\migrations\0003_article_category.py - Create model Category - Create model Article |
Now you need to migrate.
1 |
python manage.py migrate |
And this will be the output in the terminal.
1 2 3 4 |
Operations to perform: Apply all migrations: MyApp, admin, auth, contenttypes, sessions Running migrations: Applying MyApp.0003_article_category... OK |
Because we are working with Django Admin Panel, so we need to add our these two models in the admin.py file, i have already talked about django admin panel in one of my articles, you can read this article Django Creating Super User.
1 2 3 4 5 6 7 |
from django.contrib import admin from.models import Category, Article # Register your models here. admin.site.register(Category) admin.site.register(Article) |
OK now if you run your Project, and check admin panel we have our two models.
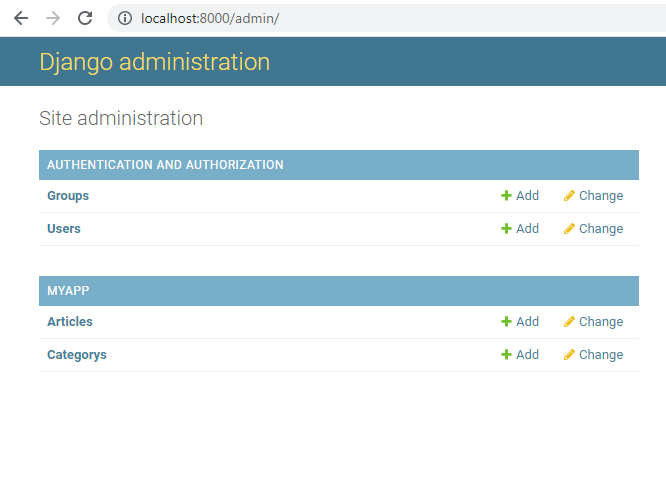
So now let’s just add some data, iam going to use Django Shell, you can use this command for opening Django Shell. make sure that you have changed your directory to your Django Project.
1 |
python manage.py shell |
We are going to add some categories like this, first we need to import the models, and after that we create the categories.
1 2 3 4 5 6 7 8 9 |
In [2]: from MyApp.models import Category, Article In [3]: c = Category(name = 'Python') In [4]: c.save() In [5]: c2 = Category(name = 'Django') In [6]: c2.save() |
Now we are going to create an Article.
1 2 3 4 |
In [7]: a = Article(title = 'Python OOP Tutorial', description = 'Python is an oop p ...: rogramming language', category = c) In [8]: a.save() |
Note that you must save an object before it can be assigned to a foreign key relationship.
OK now if you open your Django Admin Panel , and check your Article , you can see that for the first article we have the first Category.
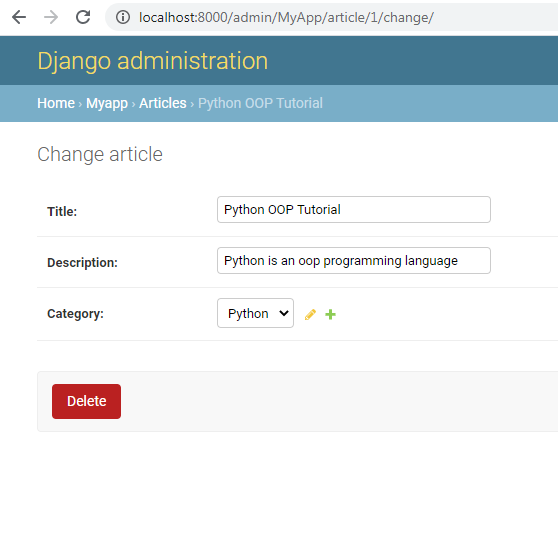
Subscribe and Get Free Video Courses & Articles in your Email