In this Android Studio article i want to show you creating ListView with SearchFilters. so now let’s start step by step tutorial on creating search filters in android list view.
1: First of all create a new project in your Android Studio, and choose Empty Activity also iam using API 20 for this project
2: After that open your build.gradle (Module:app) and in the dependencies section you need to add this library, after adding sync your project.
1 |
implementation 'com.android.support:cardview-v7:28.0.0' |
So after adding that your gradle file will look like this.
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17 18 19 20 21 22 23 24 25 26 27 28 29 |
apply plugin: 'com.android.application' android { compileSdkVersion 28 defaultConfig { applicationId "com.forogh.parwiz.listviewbaseadapter" minSdkVersion 21 targetSdkVersion 28 versionCode 1 versionName "1.0" testInstrumentationRunner "android.support.test.runner.AndroidJUnitRunner" } buildTypes { release { minifyEnabled false proguardFiles getDefaultProguardFile('proguard-android.txt'), 'proguard-rules.pro' } } } dependencies { implementation fileTree(dir: 'libs', include: ['*.jar']) implementation 'com.android.support:appcompat-v7:28.0.0' implementation 'com.android.support:cardview-v7:28.0.0' implementation 'com.android.support.constraint:constraint-layout:1.1.0' testImplementation 'junit:junit:4.12' androidTestImplementation 'com.android.support.test:runner:1.0.2' androidTestImplementation 'com.android.support.test.espresso:espresso-core:3.0.2' } |
3: After add some picture to your drawable folder.
4: OK now we need some classes for this project, these are the classes that you need to create
- Data.java class it is a POJO class
- MyAdapter.java class
- MyViewHolder.java class
- SearchFilters.java class
- ItemClickListener.java this is an interface
- Also in layout folder you need to create a model.xml file
Also you need to create a second activity for the project
So now this is our Data.java class, it is a POJO class that holds our data
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17 18 19 20 |
package com.forogh.parwiz.listviewbaseadapter; public class Data { private int Image; private String name; public Data(int image, String name) { Image = image; this.name = name; } public int getImage() { return Image; } public String getName() { return name; } } |
And this is our MyViewHolder class, this class holds our views
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17 18 19 20 21 22 23 24 25 26 27 28 29 30 31 32 33 34 35 36 37 38 39 40 41 |
package com.forogh.parwiz.listviewbaseadapter; import android.support.v7.view.menu.MenuView; import android.view.View; import android.widget.ImageView; import android.widget.TextView; import java.util.ArrayList; import java.util.Date; public class MyViewHolder implements View.OnClickListener { ImageView img; TextView nameTxt; ItemClickListener itemClickListener; public MyViewHolder(View view) { img = view.findViewById(R.id.img); nameTxt = view.findViewById(R.id.movie_title); view.setOnClickListener(this); } @Override public void onClick(View v) { this.itemClickListener.OnItemClicked(v); } public void SetClickListenet(ItemClickListener ic) { this.itemClickListener = ic; } } |
OK now we are going to create our MyAdapter class
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17 18 19 20 21 22 23 24 25 26 27 28 29 30 31 32 33 34 35 36 37 38 39 40 41 42 43 44 45 46 47 48 49 50 51 52 53 54 55 56 57 58 59 60 61 62 63 64 65 66 67 68 69 70 71 72 73 74 75 76 77 78 79 80 81 82 83 84 85 86 87 88 89 90 91 92 93 94 95 96 97 98 99 100 101 102 103 |
package com.forogh.parwiz.listviewbaseadapter; import android.content.Context; import android.content.Intent; import android.view.LayoutInflater; import android.view.View; import android.view.ViewGroup; import android.widget.AdapterView; import android.widget.BaseAdapter; import android.widget.Filter; import android.widget.Filterable; import java.util.ArrayList; import java.util.Date; import java.util.List; public class MyAdapter extends BaseAdapter implements Filterable { Context context; ArrayList<Data> data; LayoutInflater inflater; SearchFilters filter; ArrayList<Data> filteredList; public MyAdapter(Context context, ArrayList<Data> data) { this.context = context; this.data = data; this.filteredList = data; } @Override public int getCount() { return data.size(); } @Override public Object getItem(int position) { return data.get(position); } @Override public long getItemId(int position) { return position; } @Override public View getView(final int position, View convertView, ViewGroup parent) { if(inflater == null) { inflater =(LayoutInflater) context.getSystemService(Context.LAYOUT_INFLATER_SERVICE); } if(convertView == null) { convertView = inflater.inflate(R.layout.model, null); } // Binding Data final MyViewHolder myViewHolder = new MyViewHolder(convertView ); myViewHolder.img.setImageResource(data.get(position).getImage()); myViewHolder.nameTxt.setText(data.get(position).getName()); myViewHolder.SetClickListenet(new ItemClickListener() { @Override public void OnItemClicked(View view) { Intent intent = new Intent(context, SecondActivity.class); intent.putExtra("Images", data.get(position).getImage()); intent.putExtra("Name", data.get(position).getName()); context.startActivity(intent); } }); return convertView; } @Override public Filter getFilter() { if(filter == null) { filter = new SearchFilters(filteredList, this); } return filter; } } |
This is our Interface for handling click events
1 2 3 4 5 6 7 8 9 |
package com.forogh.parwiz.listviewbaseadapter; import android.view.View; public interface ItemClickListener { void OnItemClicked(View view); } |
OK now we are going to create our SearchFilter.java class
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17 18 19 20 21 22 23 24 25 26 27 28 29 30 31 32 33 34 35 36 37 38 39 40 41 42 43 44 45 46 47 48 49 50 51 52 53 54 55 56 57 58 59 60 61 62 63 64 65 66 67 |
package com.forogh.parwiz.listviewbaseadapter; import android.widget.Filter; import java.util.ArrayList; public class SearchFilters extends Filter { ArrayList<Data> filteredList; MyAdapter adapter; public SearchFilters(ArrayList<Data> filteredList, MyAdapter adapter) { this.filteredList = filteredList; this.adapter = adapter; } @Override protected FilterResults performFiltering(CharSequence constraint) { FilterResults filterResults = new FilterResults(); // doing some validation if(constraint != null && constraint.length() >0) { // Change To Upper Case constraint = constraint.toString().toUpperCase(); // Holding the data that is filtered ArrayList<Data> filteredMovie = new ArrayList<>(); for (int i = 0; i <filteredList.size(); i++ ) { if(filteredList.get(i).getName().toUpperCase().contains(constraint)) { filteredMovie.add(filteredList.get(i)); } } filterResults.count = filteredMovie.size(); filterResults.values = filteredMovie; }else { filterResults.count = filteredList.size(); filterResults.values = filteredList; } return filterResults; } @Override protected void publishResults(CharSequence constraint, FilterResults results) { adapter.data = (ArrayList<Data>) results.values; adapter.notifyDataSetChanged(); } } |
Now this is our model.xml file
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17 18 19 20 21 22 23 24 25 26 27 28 29 30 31 32 33 34 35 36 37 38 39 40 41 42 43 44 45 46 47 48 49 50 51 52 53 54 55 56 57 58 59 60 61 62 63 |
<?xml version="1.0" encoding="utf-8"?> <android.support.v7.widget.CardView xmlns:android="http://schemas.android.com/apk/res/android" xmlns:app="http://schemas.android.com/apk/res-auto" android:orientation="horizontal" android:layout_width="match_parent" android:layout_height="match_parent" app:cardCornerRadius="10dp" app:cardElevation="10dp" android:layout_margin="10dp" > <LinearLayout android:layout_width="match_parent" android:layout_height="match_parent" android:orientation="vertical" > <ImageView android:layout_width="match_parent" android:layout_height="200dp" android:src="@drawable/pic1" android:scaleType="fitXY" android:id="@+id/img" android:layout_margin="5dp" android:layout_gravity="center" /> <TextView android:layout_width="wrap_content" android:layout_height="wrap_content" android:text="Breaking Bad" android:textColor="@android:color/holo_red_dark" android:textSize="20sp" android:textStyle="bold" android:layout_margin="5dp" android:id="@+id/movie_title" android:layout_gravity="center" android:textAlignment="center" /> </LinearLayout> </android.support.v7.widget.CardView> |
Also this is our main_activity.xml, we have a ListView with SearchView in here
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17 18 19 20 21 22 23 24 25 26 |
<?xml version="1.0" encoding="utf-8"?> <LinearLayout xmlns:android="http://schemas.android.com/apk/res/android" xmlns:app="http://schemas.android.com/apk/res-auto" xmlns:tools="http://schemas.android.com/tools" android:layout_width="match_parent" android:layout_height="match_parent" android:orientation="vertical" tools:context=".MainActivity"> <android.support.v7.widget.SearchView android:layout_width="match_parent" android:layout_height="50dp" app:defaultQueryHint="Search Data" android:id="@+id/search" ></android.support.v7.widget.SearchView> <ListView android:layout_width="match_parent" android:layout_height="match_parent" android:id="@+id/listView" android:layout_margin="10dp" ></ListView> </LinearLayout> |
This is our activity_second.xml, because we are going to send the data form our list to second activity.
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17 18 19 20 21 22 23 24 25 26 27 28 29 30 31 32 |
<?xml version="1.0" encoding="utf-8"?> <LinearLayout xmlns:android="http://schemas.android.com/apk/res/android" xmlns:app="http://schemas.android.com/apk/res-auto" xmlns:tools="http://schemas.android.com/tools" android:layout_width="match_parent" android:layout_height="match_parent" android:orientation="vertical" tools:context=".SecondActivity"> <ImageView android:layout_width="match_parent" android:layout_height="400dp" android:layout_margin="10dp" android:src="@drawable/pic1" android:scaleType="fitXY" android:id="@+id/img_flower" /> <TextView android:layout_width="wrap_content" android:layout_height="wrap_content" android:text="Flower Data" android:textColor="@android:color/holo_red_dark" android:textAppearance="?android:textAppearanceLarge" android:layout_gravity="center" android:id="@+id/txt_flower" /> </LinearLayout> |
So now this is the coding for our MainActivity.java
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17 18 19 20 21 22 23 24 25 26 27 28 29 30 31 32 33 34 35 36 37 38 39 40 41 42 43 44 45 46 47 48 49 50 51 52 53 54 55 56 57 58 59 60 61 62 63 64 65 66 67 68 69 70 71 72 |
package com.forogh.parwiz.listviewbaseadapter; import android.support.v7.app.AppCompatActivity; import android.os.Bundle; import android.widget.ListView; import android.support.v7.widget.SearchView; import java.util.ArrayList; public class MainActivity extends AppCompatActivity { ListView listView; SearchView searchView; @Override protected void onCreate(Bundle savedInstanceState) { super.onCreate(savedInstanceState); setContentView(R.layout.activity_main); listView = findViewById(R.id.listView); searchView = findViewById(R.id.search); final MyAdapter adapter = new MyAdapter(this, getData()); listView.setAdapter(adapter); searchView.setOnQueryTextListener(new SearchView.OnQueryTextListener() { @Override public boolean onQueryTextSubmit(String query) { return false; } @Override public boolean onQueryTextChange(String newText) { adapter.getFilter().filter(newText); return false; } }); } private ArrayList<Data> getData() { ArrayList<Data> data = new ArrayList<>(); Data m = new Data(R.drawable.pic1, "This is data one"); data.add(m); m = new Data(R.drawable.pic2, "This is data two"); data.add(m); m = new Data(R.drawable.pic3, "This is data three"); data.add(m); m = new Data(R.drawable.pic4, "This is data four"); data.add(m); m = new Data(R.drawable.pic5, "This is data five"); data.add(m); m = new Data(R.drawable.pic6, "This is data six"); data.add(m); return data; } } |
And now this is our SecondActivity.java
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17 18 19 20 21 22 23 24 25 26 27 28 29 |
package com.forogh.parwiz.listviewbaseadapter; import android.support.v7.app.AppCompatActivity; import android.os.Bundle; import android.widget.ImageView; import android.widget.TextView; public class SecondActivity extends AppCompatActivity { ImageView imageView; TextView textView; @Override protected void onCreate(Bundle savedInstanceState) { super.onCreate(savedInstanceState); setContentView(R.layout.activity_second); imageView = findViewById(R.id.img_flower); textView = findViewById(R.id.txt_flower); final int images = getIntent().getIntExtra("Images", 0); final String name = getIntent().getStringExtra("Name"); imageView.setImageResource(images); textView.setText(name); } } |
The result of the project will be like this
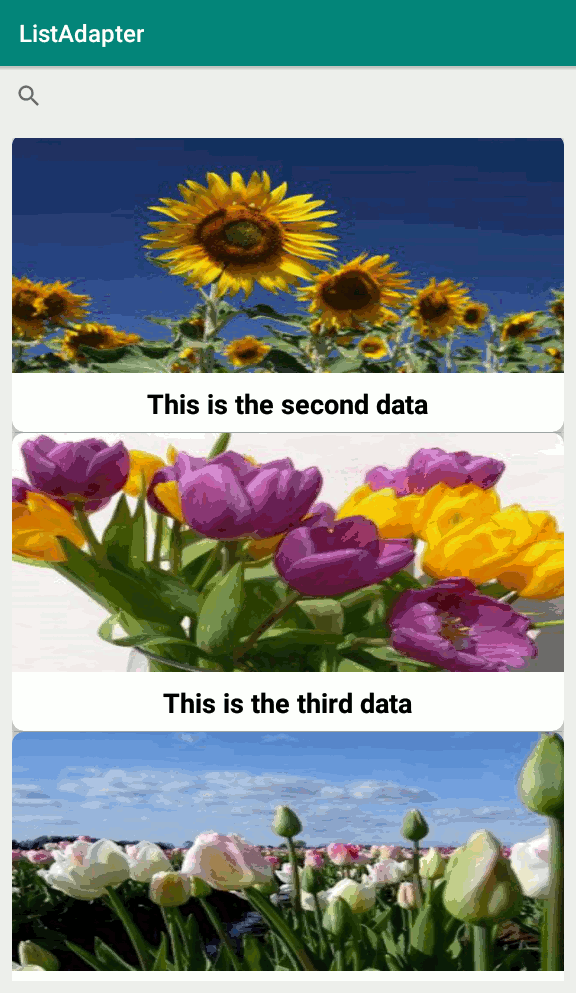
Subscribe and Get Free Video Courses & Articles in your Email