In this Python TKinter article i want to show you Creating MenuItem Events and Commands in TKinter. first of all let’s talk about MenuItems in TKinter.
What is MenuItem in TKinter?
In Tkinter, MenuItem is a class that represents an item in a menu. Menus are a common graphical user interface element used to provide users with a set of options or commands that they can select. and we can say that MenuItem typically appears as a labeled option inside a menu.
These some key points about MenuItem in Tkinter:
- Menu Structure: MenuItem is usually part of a hierarchical structure inside a menu. It can be a top level item or a submenu item.
- Labeling: Each MenuItem typically has a label associated with it, and it is the text of that, and it is displayed to the user in the menu.
- Event Handling: You can bind events to MenuItem objects to perform actions when the user selects them. This allows you to define callbacks that execute specific functions or commands when a menu item is clicked.
- Cascading Menus: Tkinter supports cascading menus, where a MenuItem can open a submenu containing additional options. This allows you to organize related options into hierarchical structures.
This is the complete code for this article
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17 18 19 20 21 22 23 24 25 26 27 28 29 30 31 32 33 34 35 36 37 38 39 40 41 42 43 44 45 46 |
import tkinter as tk from tkinter import Menu class App(tk.Tk): def __init__(self): super(App, self).__init__() # Set title of the window self.title("Codeloop.org - Menus") # Set window icon self.iconphoto(True, tk.PhotoImage(file='codeloop.png')) # Call method to create the menu self.createMenu() def closeWindow(self): # Quit application and destroy the window self.quit() self.destroy() # Exit Python interpreter exit() def createMenu(self): # Create menu bar menuBar = Menu(self) self.config(menu=menuBar) # Create File menu file_menu = Menu(menuBar, tearoff=0) file_menu.add_command(label='New') file_menu.add_command(label='Exit', command=self.closeWindow) file_menu.add_separator() file_menu.add_command(label="Open") menuBar.add_cascade(label="File", menu=file_menu) # Create Help menu help_menu = Menu(menuBar, tearoff=0) menuBar.add_cascade(label='Help', menu=help_menu) help_menu.add_command(label='About') # Create an instance of the App class app = App() app.mainloop() |
Now let’s describe above code:
- Imports: In the above we import the necessary modules from the Tkinter library, including tkinter itself and the Menu class.
- App Class: After that we define a class at name of App, and it inherits from tk.Tk class, it represents the main window of the application.
- Initialization: __init__ method of the App class initializes the application. It sets the title of the window and sets an icon for the window using iconphoto() method.
- Menu Creation: createMenu method inside the App class is responsible for creating the menu bar and its associated menus. It first creates a Menu object (menuBar) for the main menu bar of the application. After that it creates Menu Items.
- Event Handling: closeWindow method is called when the Exit option in the File menu is selected. It quits the application, destroys the window and exits the Python interpreter.
- Instance Creation: An instance of the App class is created (app = App()) and the Tkinter event loop is started (app.mainloop()), and it listens for events such as button clicks and updates the GUI accordingly.
Run the complete code and this will be the result
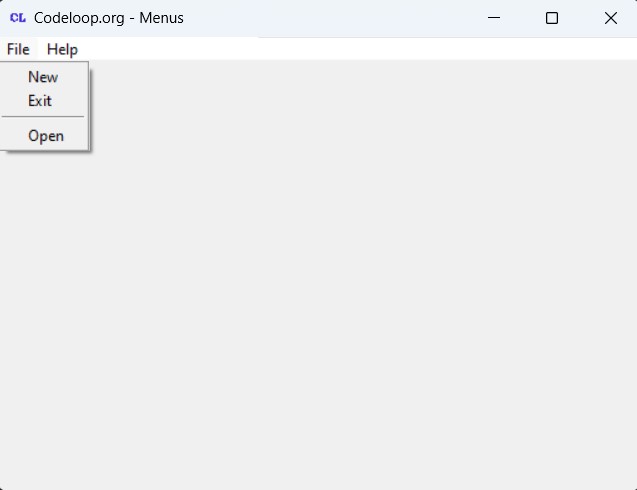
Subscribe and Get Free Video Courses & Articles in your Email