In this Python article i want to show you How to Make Browser in PyQt5 with PyQtWebEngine.
Also you can check more Python GUI articles in the below links
1: Kivy GUI Development Tutorials
2: Python TKinter GUI Development
5: PyQt5 GUI Development Course
What is PyQtWebEngine?
PyQtWebEngine is a set of Python bindings for The Qt Company’s Qt WebEngine framework.
The framework provides the ability to embed web content in applications and is based on
the Chrome browser. The bindings sit on top of PyQt5 and are implemented as three
separate modules corresponding to the different libraries that make up the framework.
License
PyQtWebEngine is released under the GPL v3 license and under a commercial license
that allows for the development of proprietary applications.
Installation
PyQtWebEngine source packages for the GPL version can be dowloaded from River Bank Computing Website
Wheels for the GPL version for 32 and 64-bit Windows, 64-bit OS X and 64-bit Linux can be installed from PyPI:
pip install PyQtWebEngine
pip3 install PyQtWebEngine
OK now this is the complete code for Python How to Make Browser in PyQt5 with PyQtWebEngine
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17 18 |
import sys from PyQt5.Qt import * from PyQt5.QtWebEngineWidgets import * from PyQt5.QtWidgets import QApplication app = QApplication(sys.argv) web = QWebEngineView() web.load(QUrl("https://www.codeloop.org")) web.show() sys.exit(app.exec_()) |
So in the above code these are the imports that we need.
1 2 3 4 |
import sys from PyQt5.Qt import * from PyQt5.QtWebEngineWidgets import * from PyQt5.QtWidgets import QApplication |
And this is the object of QApplication and QWebEngineView.
1 2 3 |
app = QApplication(sys.argv) web = QWebEngineView() |
OK now in here we are going to load our url also we need to show that.
1 2 3 |
web.load(QUrl("https://www.codeloop.org")) web.show() |
So run the complete code and this will be the result
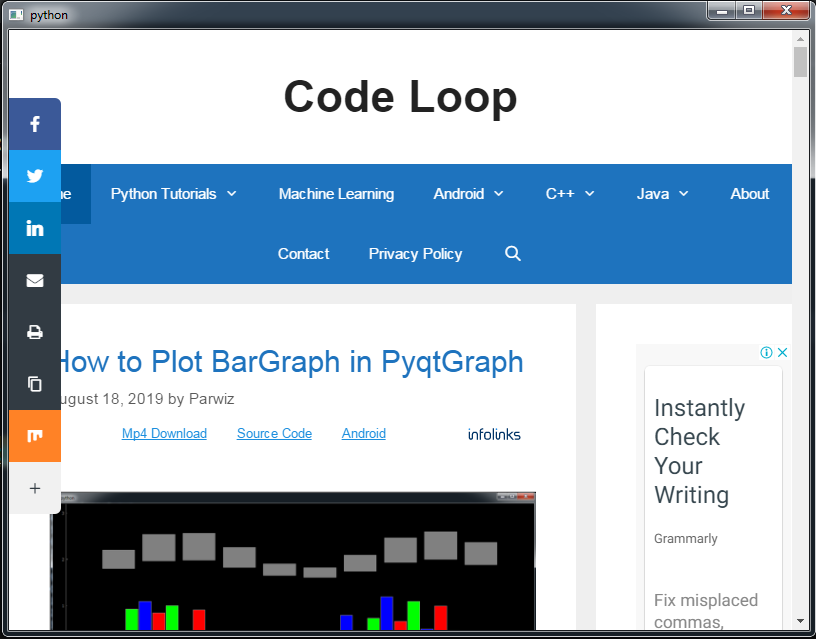
Also you can watch the complete video for this article
Subscribe and Get Free Video Courses & Articles in your Email
Hello & Thanks :
If you entered a search arg in the Google site , would it have navigated to search results ,
and if you clicked on one of those links , would it have navigated to that site ?
I have written a kidSafeBrowser in vb.net , but its IE11 , so much good .
So I a looking alternatives .
In your Browser can I check clicked-on-link and compare it against a SafeSites.txt file .
If there’s a hit , go there , else no go ?
Thanks for your Help…
Vernon Marsden
PyQtWebEngine class is a big class and you can do everything you want, just check the documentation for more information.
Hello,
For some reason this doesn’t work. It runs through the code and exits without showing the browser. Others on your youtube video are posting the same issue. Can you please help with this? Thank you.
can you run the code via terminal and see what is the error, using terminal you can track the error, get the error and comment here i will check that