In this Python GUI article i want to show you How to Make Employee Application in PyQt5,
basically we are using QListWidget in this article. before starting our main topic if your
interested in Python GUI Development with PyQt5, you can check the below link.
Also you can check more Python GUI articles in the below links
1: Kivy GUI Development Tutorials
2: Python TKinter GUI Development
5: PyQt5 GUI Development Course
What is QListWidget ?
QListWidget is a convenience class that provides a list view similar to the one supplied by QListView, but with a classic item-based interface for adding and removing items. QListWidget uses an internal model to manage each QListWidgetItem in the list. For a more flexible list view widget, use the QListView class with a standard model. List widgets are constructed in the same way as other widgets:
Also in this video we have designed our application using Qt Designer, you can watch
the complete process for this article in the below video .
So now this is the complete source code for this article.
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17 18 19 20 21 22 23 24 25 26 27 28 29 30 31 32 33 34 35 36 37 38 39 40 41 42 43 44 45 46 47 48 49 50 51 52 53 54 55 56 57 58 59 60 61 62 63 64 65 66 67 68 69 70 71 72 73 74 75 76 77 78 79 80 81 82 83 84 85 86 87 88 89 90 91 92 93 94 95 96 97 98 99 100 101 102 103 104 105 106 107 108 109 110 111 112 113 114 115 116 117 118 119 120 121 122 123 124 125 126 127 128 129 130 131 132 133 134 135 136 137 138 139 140 141 142 143 144 145 146 147 148 149 150 151 152 153 154 155 156 157 158 159 160 161 |
from PyQt5 import QtCore, QtGui, QtWidgets from PyQt5.QtWidgets import QInputDialog, QDialog, QLineEdit, QMessageBox from PyQt5.QtGui import QIcon class Window(QDialog): def setupUi(self, Dialog): Dialog.setObjectName("Dialog") Dialog.resize(574, 470) self.verticalLayout_2 = QtWidgets.QVBoxLayout(Dialog) self.verticalLayout_2.setContentsMargins(11, 11, 11, 11) self.verticalLayout_2.setSpacing(6) self.verticalLayout_2.setObjectName("verticalLayout_2") self.horizontalLayout = QtWidgets.QHBoxLayout() self.horizontalLayout.setContentsMargins(11, 11, 11, 11) self.horizontalLayout.setSpacing(6) self.horizontalLayout.setObjectName("horizontalLayout") self.listWidget = QtWidgets.QListWidget(Dialog) self.listWidget.setObjectName("listWidget") self.horizontalLayout.addWidget(self.listWidget) self.verticalLayout = QtWidgets.QVBoxLayout() self.verticalLayout.setContentsMargins(11, 11, 11, 11) self.verticalLayout.setSpacing(6) self.verticalLayout.setObjectName("verticalLayout") self.pushButton_add = QtWidgets.QPushButton(Dialog) self.pushButton_add.setObjectName("pushButton_add") self.verticalLayout.addWidget(self.pushButton_add) self.pushButton_edit = QtWidgets.QPushButton(Dialog) self.pushButton_edit.setObjectName("pushButton_edit") self.verticalLayout.addWidget(self.pushButton_edit) self.pushButton_remove = QtWidgets.QPushButton(Dialog) self.pushButton_remove.setObjectName("pushButton_remove") self.verticalLayout.addWidget(self.pushButton_remove) self.pushButton_up = QtWidgets.QPushButton(Dialog) self.pushButton_up.setObjectName("pushButton_up") self.verticalLayout.addWidget(self.pushButton_up) self.pushButton_down = QtWidgets.QPushButton(Dialog) self.pushButton_down.setObjectName("pushButton_down") self.verticalLayout.addWidget(self.pushButton_down) self.pushButton_sort = QtWidgets.QPushButton(Dialog) self.pushButton_sort.setObjectName("pushButton_sort") self.verticalLayout.addWidget(self.pushButton_sort) spacerItem = QtWidgets.QSpacerItem(20, 40, QtWidgets.QSizePolicy.Minimum, QtWidgets.QSizePolicy.Expanding) self.verticalLayout.addItem(spacerItem) self.pushButton_close = QtWidgets.QPushButton(Dialog) self.pushButton_close.setObjectName("pushButton_close") self.verticalLayout.addWidget(self.pushButton_close) self.horizontalLayout.addLayout(self.verticalLayout) self.verticalLayout_2.addLayout(self.horizontalLayout) self.retranslateUi(Dialog) QtCore.QMetaObject.connectSlotsByName(Dialog) self.pushButton_add.clicked.connect(self.add) self.pushButton_edit.clicked.connect(self.edit) self.pushButton_remove.clicked.connect(self.remove) self.pushButton_up.clicked.connect(self.up) self.pushButton_down.clicked.connect(self.down) self.pushButton_sort.clicked.connect(self.sort) self.pushButton_close.clicked.connect(self.close) self.Employee() def retranslateUi(self, Dialog): _translate = QtCore.QCoreApplication.translate Dialog.setWindowTitle(_translate("Dialog", "Employee")) Dialog.setWindowIcon(QIcon("icon.png")) self.pushButton_add.setText(_translate("Dialog", "Add")) self.pushButton_edit.setText(_translate("Dialog", "Edit")) self.pushButton_remove.setText(_translate("Dialog", "Remove")) self.pushButton_up.setText(_translate("Dialog", "Up")) self.pushButton_down.setText(_translate("Dialog", "Down")) self.pushButton_sort.setText(_translate("Dialog", "Sort")) self.pushButton_close.setText(_translate("Dialog", "Close")) def Employee(self): self.employess = ["John", "Doe", "Tom", "Karim", "Ahmad"] self.listWidget.addItems(self.employess) self.listWidget.setCurrentRow(0) def add(self): row = self.listWidget.currentRow() text, ok = QInputDialog.getText(self, "Employee Dialog", "Enter Employee Name") if ok and text is not None: self.listWidget.insertItem(row, text) def edit(self): row = self.listWidget.currentRow() item = self.listWidget.item(row) if item is not None: string, ok = QInputDialog.getText(self, "Employee Dialog", "Edit Employee Name", QLineEdit.Normal, item.text()) if ok and string is not None: item.setText(string) def remove(self): row = self.listWidget.currentRow() item = self.listWidget.item(row) if item is None: return reply = QMessageBox.question(self, "Remove Employee", "Do You Want To Remove Employee " + str(item.text()), QMessageBox.Yes|QMessageBox.No) if reply == QMessageBox.Yes: item = self.listWidget.takeItem(row) del item def up(self): row = self.listWidget.currentRow() if row >= 1: item = self.listWidget.takeItem(row) self.listWidget.insertItem(row - 1, item) self.listWidget.setCurrentItem(item) def down(self): row = self.listWidget.currentRow() if row < self.listWidget.count() - 1: item = self.listWidget.takeItem(row) self.listWidget.insertItem(row + 1, item) self.listWidget.setCurrentItem(item) def sort(self): self.listWidget.sortItems() def close(self): quit() if __name__ == "__main__": import sys app = QtWidgets.QApplication(sys.argv) Dialog = QtWidgets.QDialog() ui = Window() ui.setupUi(Dialog) Dialog.show() sys.exit(app.exec_()) |
Run the complete code and this will be the result.
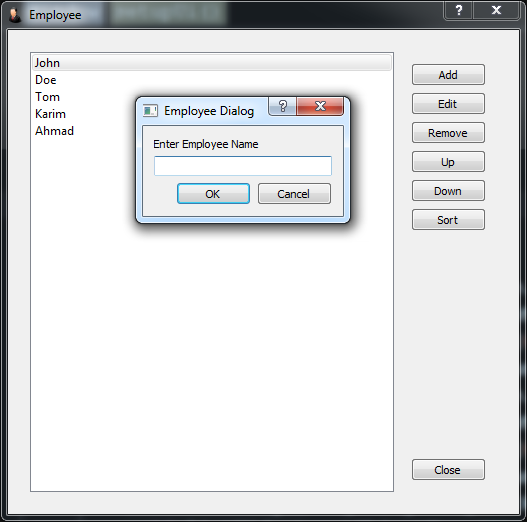
Subscribe and Get Free Video Courses & Articles in your Email