In this PyQtChart article iam going to show you How to Create DonutChart in PyQt5.
we are going to use PyQtChart library for this article, so PyQtChart is a set of Python bindings
for The Qt Company’s Qt Charts library. The bindings sit on top of PyQt5 and are implemented
as a single module, The commercial version of PyQtChart is bundled with the commercial
version of PyQt. also you can check my older articles in PyQtChart in the below links.
Read PyQtChart More Articles
1: PyQtChart How to Create BarChart in PyQt5
2: PyQtChart How to Create LineChart in PyQt5
3: PyQtChart How to Create PieChart in PyQt5
Also you can check more Python GUI articles in the below links.
1: Kivy GUI Development Tutorials
2: Python TKinter GUI Development
5: PyQt5 GUI Development Course
What is DonutChart ?
A donut chart is a Pie Chart with an area of the center cut out.
Pie Charts are sometimes criticized for focusing readers on the proportional areas of
the slices to one another and to the chart as a whole. This makes it tricky to see the
differences between slices, especially when you try to compare multiple Pie Charts together.
A Donut Chart somewhat remedies this problem by de-emphasizing the use of the area.
Instead, readers focus more on reading the length of the arcs, rather than comparing
the proportions between slices.
So now this is the complete code for PyQtChart How to Create DonutChart in PyQt5.
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17 18 19 20 21 22 23 24 25 26 27 28 29 30 31 32 33 34 35 36 37 38 39 40 41 42 43 44 45 46 47 48 49 50 51 52 53 54 55 56 57 58 59 60 61 62 63 |
from PyQt5.QtWidgets import QApplication, QMainWindow import sys from PyQt5.QtChart import QChart, QChartView, QPieSeries, QPieSlice from PyQt5.QtGui import QPainter, QPen, QFont from PyQt5.QtCore import Qt class Window(QMainWindow): def __init__(self): super().__init__() self.setWindowTitle("DonutChart Example") self.setGeometry(100,100, 1280,600) self.show() self.create_donutchart() def create_donutchart(self): series = QPieSeries() series.setHoleSize(0.35) series.append("Protein 4.2%", 4.2) slice = QPieSlice() slice = series.append("Fat 15.6%", 15.6) slice.setExploded() slice.setLabelVisible() series.append("Other 23.8%", 23.8); series.append("Carbs 56.4%", 56.4); chart = QChart() chart.legend().hide() chart.addSeries(series) chart.setAnimationOptions(QChart.SeriesAnimations) chart.setTitle("DonutChart Example") chart.setTheme(QChart.ChartThemeBlueCerulean) chartview = QChartView(chart) chartview.setRenderHint(QPainter.Antialiasing) self.setCentralWidget(chartview) App = QApplication(sys.argv) window = Window() sys.exit(App.exec_()) |
So in the above code first of all we have imported our required classes form QtChart
and PyQt5, QChart and QChartView are both essential for working of Charts in PyQt5,
also we have imported QPieSeries and QPieSlice. after that we have created our Window
class that extends from QMainWindow, and we have added our window title and window
geometry in this class also we have called our create_donutchart() method.
1 2 3 4 5 6 7 8 9 10 |
class Window(QMainWindow): def __init__(self): super().__init__() self.setWindowTitle("DonutChart Example") self.setGeometry(100,100, 1280,600) self.show() self.create_donutchart() |
This is the code for creating donutchart.
1 2 3 4 5 6 7 8 9 |
series = QPieSeries() series.setHoleSize(0.35) series.append("Protein 4.2%", 4.2) slice = QPieSlice() slice = series.append("Fat 15.6%", 15.6) slice.setExploded() slice.setLabelVisible() series.append("Other 23.8%", 23.8) series.append("Carbs 56.4%", 56.4) |
OK, in the above code first of all we have created the object for QPieSeries, in the introduction
of donutchart we have said, that it is a type of PieChart. and we are adding some data to
the QPieSeries with some functionality of DonutChart.
OK now i have said that when you want to create some kind of charts in PyQtChart,
creating of QChart and QChartView are important for every kind of charts.
1 2 3 4 5 6 |
chart = QChart() chart.legend().hide() chart.addSeries(series) chart.setAnimationOptions(QChart.SeriesAnimations) chart.setTitle("DonutChart Example") |
This is for creating of theme for our donutchart.
1 |
chart.setTheme(QChart.ChartThemeBlueCerulean) |
And at the end we create our QChartView and we add our QChart object in our ChartView.
1 |
chartview = QChartView(chart) |
Also we need to make chartview as central widget of our window, if we don’t do this, there
will not be any chart in the window.
1 |
self.setCentralWidget(chartview) |
Also every PyQt5 application must create an application object.
1 |
App = QApplication(sys.argv) |
Finally, we enter the mainloop of the application. The event handling starts from this point.
1 |
sys.exit(App.exec_()) |
Run the complete code and this will be the result.
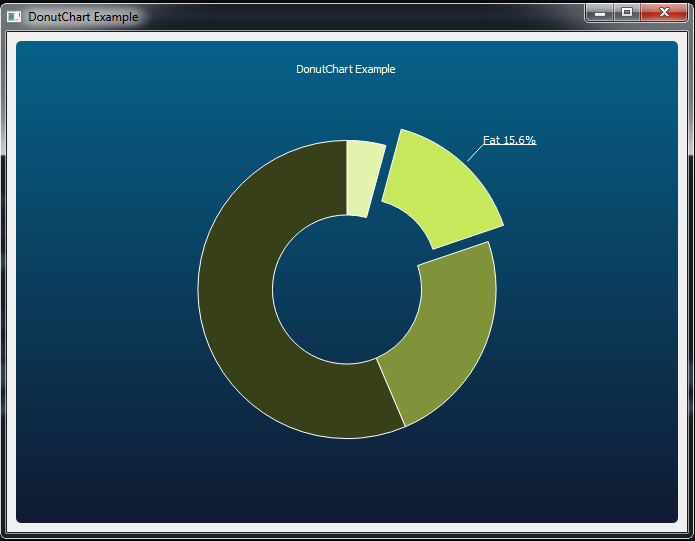
Also you can watch the complete video for this article
Subscribe and Get Free Video Courses & Articles in your Email
Thanks Parwiz