In this PyQtChart article iam going to show you How to Create BarChart in PyQt5.
we are going to use PyQtChart library for this article, so PyQtChart is a set of Python bindings
for The Qt Company’s Qt Charts library. The bindings sit on top of PyQt5 and are implemented
as a single module. The commercial version of PyQtChart is bundled with the commercial
version of PyQt.
Read PyQtChart More Articles
1: PyQtChart How to Create LineChart in PyQt5
2: PyQtChart How to Create PieChart in PyQt5
3: PyQt5 How to Create DonutChart
Also you can check more Python GUI articles in the below links.
1: Kivy GUI Development Tutorials
2: Python TKinter GUI Development
5: PyQt5 GUI Development Course
Types of charts and graphs in Qt
Qt supports most commonly used diagrams, and even allows the developer to customize
the look and feel of them so that they can be used for many different purposes. The Qt
Charts module provides the following chart types:
- Line and spline charts
- Bar charts
- Pie charts
- Polar charts
- Area and scatter charts
- Box-and-whiskers charts
- Candlestick charts
Whats are BarCharts ?
Bar charts are one of the most commonly used diagrams beside line charts and pie charts. A
bar chart is quite similar to a line chart, except it doesn’t connect the data along an axis.
Instead, a bar chart displays its data using individual rectangular shapes, where its height is
determined by the value of the data.
Installation
The GPL version of PyQtChart can be installed from PyPI:
1 |
pip install PyQtChart |
The wheels include a copy of the required parts of the LGPL version of Qt.
pip will also build and install the bindings from the sdist package but Qt’s qmake tool must be on PATH.
The sip-install tool will also install the bindings from the sdist package but will allow you to configure many aspects of the installation.
So now this is the complete code for PyQtChart How to Create BarChart in PyQt5
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17 18 19 20 21 22 23 24 25 26 27 28 29 30 31 32 33 34 35 36 37 38 39 40 41 42 43 44 45 46 47 48 49 50 51 52 53 54 55 56 57 58 59 60 61 62 63 64 65 66 67 68 69 70 |
from PyQt5.QtWidgets import QApplication, QMainWindow import sys from PyQt5.QtChart import QChart, QChartView, QBarSet, QPercentBarSeries, QBarCategoryAxis from PyQt5.QtGui import QPainter from PyQt5.QtCore import Qt class Window(QMainWindow): def __init__(self): super().__init__() self.setWindowTitle("PyQt BarChart") self.setGeometry(100,100, 680,500) self.show() self.create_bar() def create_bar(self): #The QBarSet class represents a set of bars in the bar chart. # It groups several bars into a bar set set0 = QBarSet("Parwiz") set1 = QBarSet("Bob") set2 = QBarSet("Tom") set3 = QBarSet("Logan") set4 = QBarSet("Karim") set0 << 1 << 2 << 3 << 4 << 5 << 6 set1 << 5 << 0 << 0 << 4 << 0 << 7 set2 << 3 << 5 << 8 << 13 << 8 << 5 set3 << 5 << 6 << 7 << 3 << 4 << 5 set4 << 9 << 7 << 5 << 3 << 1 << 2 series = QPercentBarSeries() series.append(set0) series.append(set1) series.append(set2) series.append(set3) series.append(set4) chart = QChart() chart.addSeries(series) chart.setTitle("Percent Example") chart.setAnimationOptions(QChart.SeriesAnimations) categories = ["Jan", "Feb", "Mar", "Apr", "May", "Jun"] axis = QBarCategoryAxis() axis.append(categories) chart.createDefaultAxes() chart.setAxisX(axis, series) chart.legend().setVisible(True) chart.legend().setAlignment(Qt.AlignBottom) chartView = QChartView(chart) chartView.setRenderHint(QPainter.Antialiasing) self.setCentralWidget(chartView) App = QApplication(sys.argv) window = Window() sys.exit(App.exec_()) |
So in the above code first of all we have imported our required classes form QtChart and PyQt5, QChart and QChartView are both essential for working of Charts in PyQt5, after that we have created our Window class that inherits from QMainWindow, and we have added our window title and window geometry in this class also we have called our create_bar() method.
1 2 3 4 5 6 7 8 |
class Window(QMainWindow): def __init__(self): super().__init__() self.setWindowTitle("PyQt BarChart") self.setGeometry(100,100, 1280,720) self.show() self.create_bar() |
And this is the method for creating of our BarChart, basically it is called QBarSeries in QtChart. first of all we have created some barsets using QBarSet(), and we have added some names in our QBarSet(). after that we have created some set of values, because we are using percent bar set, we need to create QPercentBarSeries. also we have appended our sets in our QPercentBarSet.
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17 18 19 20 21 22 |
def create_bar(self): #The QBarSet class represents a set of bars in the bar chart. # It groups several bars into a bar set set0 = QBarSet("Parwiz") set1 = QBarSet("Bob") set2 = QBarSet("Tom") set3 = QBarSet("Logan") set4 = QBarSet("Karim") set0 << 1 << 2 << 3 << 4 << 5 << 6 set1 << 5 << 0 << 0 << 4 << 0 << 7 set2 << 3 << 5 << 8 << 13 << 8 << 5 set3 << 5 << 6 << 7 << 3 << 4 << 5 set4 << 9 << 7 << 5 << 3 << 1 << 2 series = QPercentBarSeries() series.append(set0) series.append(set1) series.append(set2) series.append(set3) series.append(set4) |
OK now i have said that when you want to create some kind of charts in PyQtChart, creating of QChart and QChartView are important for every kind of charts. and now first we create our QChart object and we add our series to QChart object and we set a title with animation for our QChart.
1 2 3 4 |
chart = QChart() chart.addSeries(series) chart.setTitle("Percent Example") chart.setAnimationOptions(QChart.SeriesAnimations) |
In here we create some categories for our QChart.
1 2 3 4 5 6 7 8 |
categories = ["Jan", "Feb", "Mar", "Apr", "May", "Jun"] axis = QBarCategoryAxis() axis.append(categories) chart.createDefaultAxes() chart.setAxisX(axis, series) chart.legend().setVisible(True) chart.legend().setAlignment(Qt.AlignBottom) |
And at the end we create our QChartView and we add our QChart object in our ChartView.
1 2 3 |
chartView = QChartView(chart) chartView.setRenderHint(QPainter.Antialiasing) self.setCentralWidget(chartView) |
Also every PyQt5 application must create an application object. The sys.argv
parameter is a
list of arguments from a command line.
1 |
App = QApplication(sys.argv) |
Finally, we enter the mainloop of the application. The event handling starts from this point.
1 2 |
window = Window() sys.exit(App.exec_()) |
So now run the complete code and this will be the result
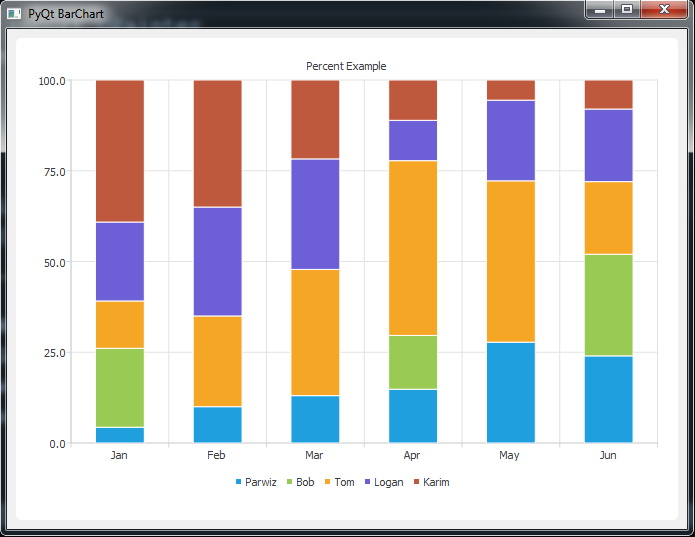
Also you can watch the complete video for this article
Subscribe and Get Free Video Courses & Articles in your Email
Comments are closed.