In this PyQt6 Tutorial we want to learn how to Create LineEdit with QLineEdit, so first of all let’s talk about QLineEdit.
What is QLineEdit in PyQt6 ?
QLineEdit is a widget provided by PyQt6 library for creating single line input fields in PyQt6. It allows users to enter and edit a single line of text. QLineEdit is commonly used in different types of applications, such as forms, search bars, login screens and more.
Key features of QLineEdit:
- Input Handling: Users can add input texts using their keyboard, and QLineEdit widget provides functionalities for editing and manipulating the entered text, such as selecting, copying, cutting and pasting.
- Placeholder Text: QLineEdit supports placeholder text, and it is displayed as a hint to users about the expected input format or purpose of the field when it’s empty.
- Validation: Developers can implement input validation to restrict the type or format of the input data, and it ensures that users enter valid information.
- Signals and Slots: QLineEdit emits signals, such as textChanged, editingFinished and returnPressed, which developers can connect to custom slots to perform actions in response to user interactions.
This is the complete code for this article
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17 18 19 |
import sys from PyQt6.QtWidgets import QApplication, QMainWindow, QLineEdit class MainWindow(QMainWindow): def __init__(self): super().__init__() self.setWindowTitle("Codeloop - PyQt6 LineEdit") # Create a QLineEdit widget line_edit = QLineEdit(self) # Set the position and size of the QLineEdit line_edit.setGeometry(100, 50, 200, 30) if __name__ == "__main__": app = QApplication(sys.argv) window = MainWindow() window.show() sys.exit(app.exec()) |
Now let’s describe this code
- We have started by importing the necessary modules
- After that, we have defined a MainWindow class that inherits from QMainWindow. also we have added title in the constructor.
- Inside the MainWindow class, we have created a QLineEdit widget named line_edit and specify its position and size using the setGeometry method.
- We have created a QApplication instance, and then instantiated the MainWindow class, also displayed the window using show(), and start the application event loop with app.exec().
Run the code and this will be the result
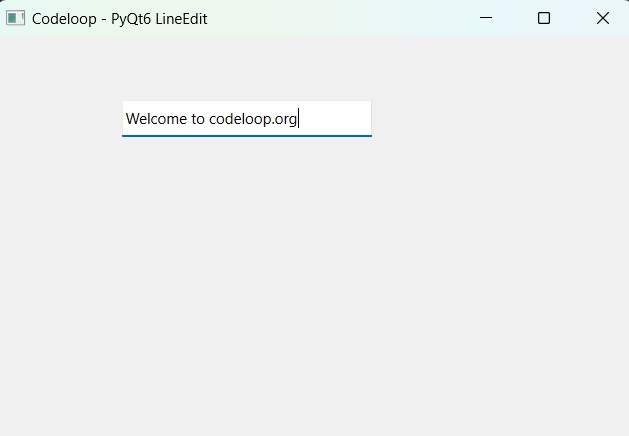
How to Customize QLineEdit in PyQt6
In PyQt6, you can customize the appearance and behavior of a QLineEdit widget using different properties and methods. These are some common customization options:
Text Appearance:
- You can set the font, font size and font style using the setFont() method.
- Change the text color with the setStyleSheet() method by specifying a CSS style.
- Adjust the alignment of the text using setAlignment().
Background and Border:
- You can customize the background color of the QLineEdit with setStyleSheet() by setting the background-color property.
- You can Modify the border style and color using CSS properties like border-width, border-style, and border-color.
Placeholder Text:
- You can set placeholder text to provide hints or instructions to the user before they input any text. Use setPlaceholderText() method.
Input Validation:
- Implement custom input validation using the setValidator() method to restrict input based on certain criteria, such as numeric input only, regular expressions, etc.
Echo Mode:
- You can control how the entered text is displayed using the setEchoMode() method. Options include Normal, No Echo, Password, and Password Echo On Edit.
Clear Button:
- You can enable a clear button on the right side of the QLineEdit to allow users to easily clear the input text with the setClearButtonEnabled() method.
Cursor and Selection:
- Customize the appearance of the cursor and selection highlight using CSS properties like caret-color and selection-color.
This is the complete code
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17 18 19 20 21 22 23 24 25 26 27 28 29 30 31 32 33 34 35 36 37 38 39 |
import sys from PyQt6.QtWidgets import QApplication, QMainWindow, QLineEdit from PyQt6.QtGui import QFont class MainWindow(QMainWindow): def __init__(self): super().__init__() self.setWindowTitle("Customize QLineEdit Example") line_edit = QLineEdit(self) line_edit.setGeometry(100, 50, 200, 30) # Text appearance customization line_edit.setStyleSheet("color: blue; background-color: lightgray; border: 2px solid gray;") # Create a QFont object with the desired font family and size font = QFont() font.setFamily("Arial") font.setPointSize(12) # Set the font for the QLineEdit line_edit.setFont(font) # Placeholder text line_edit.setPlaceholderText("Enter your text here") # Echo mode line_edit.setEchoMode(QLineEdit.EchoMode.Normal) # Enable clear button line_edit.setClearButtonEnabled(True) if __name__ == "__main__": app = QApplication(sys.argv) window = MainWindow() window.show() sys.exit(app.exec()) |
This code creates a customized QLineEdit widget with a blue text color, light gray background, and a gray border. It sets the font size to 12 and aligns the text to the center. Also it displays placeholder text, enables the clear button, and sets the echo mode to Normal.
Run the code and this will be the result
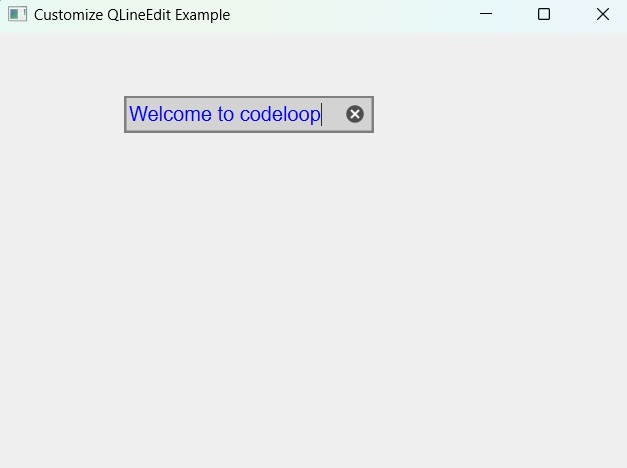
How to Add Signals and Slots to PyQt6 QLineEdit
In PyQt6, signals and slots are used to establish communication between different widgets or components. QLineEdit widgets emit different signals when their state changes, such as when the text is edited, when the return key is pressed, or when the widget gains or loses focus. You can connect these signals to custom slots to perform specific actions in response to these events.
This is an example of PyQt6 Signals and Slots with QLineEdit
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17 18 19 20 21 22 23 24 25 26 27 28 |
import sys from PyQt6.QtWidgets import QApplication, QMainWindow, QLineEdit class MainWindow(QMainWindow): def __init__(self): super().__init__() self.setWindowTitle("Signals and Slots Example") self.setGeometry(100, 100, 400, 200) # Create a QLineEdit widget self.line_edit = QLineEdit(self) self.line_edit.setGeometry(100, 50, 200, 30) # Connect the textChanged signal to the text_changed_slot function self.line_edit.textChanged.connect(self.text_changed_slot) # Custom slot function to handle the textChanged signal def text_changed_slot(self, text): if text: print("Text changed:", text) else: print("Text cleared") if __name__ == "__main__": app = QApplication(sys.argv) window = MainWindow() window.show() sys.exit(app.exec()) |
- We have created a QLineEdit widget and connect its textChanged signal to the text_changed_slot function.
- The text_changed_slot function receives the current text of the QLineEdit as a parameter whenever the text changes.
- Inside the slot function, we print the text when it changes or print a message when the text is cleared.
FAQs
Q: What is a QLineEdit?
A: QLineEdit is a widget in PyQt6 used to input and edit a single line of text. It provides a simple interface for users to enter text data.
Q: How do I set the initial text in a QLineEdit?
A: You can set the initial text of a QLineEdit using the setText() method. For example: line_edit.setText(“Initial text”).
Q: How can I handle actions such as pressing the Enter key in aQLineEdit?
A: You can handle actions such as pressing the Enter key by connecting the appropriate signals, such as returnPressed, to custom slot functions. These signals are emitted when specific user actions occur.
Learn More on PyQt6 Widgets:
Subscribe and Get Free Video Courses & Articles in your Email