In this tutorial we want to learn about PyQt5 Input Widgets, we are going to talk about PyQt5 TextBox, SpinBox and Slider, we already know that PyQt5 is one of the most popular GUI framework for Python programming language, so now let’s start from PyQt5 TextBox.
PyQt5 TextBox
Using PyQt5 TextBoxes you can enter and edit text data. They are commonly used for inputting names, addresses or any textual information. PyQt5 offers the QLineEdit widget, which is perfect for handling text input.
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17 18 19 20 21 22 23 24 25 26 27 28 29 30 31 32 33 34 35 36 37 38 39 40 41 42 43 44 45 46 47 |
from PyQt5.QtWidgets import QApplication, QWidget, QVBoxLayout, QLabel, QLineEdit import sys class RegistrationForm(QWidget): def __init__(self): super().__init__() self.initUI() def initUI(self): # Set window title self.setWindowTitle('Registration Form') # Create a vertical layout vbox = QVBoxLayout() # Create a label for the name field nameLabel = QLabel('Name:', self) # Create a text input field for the name nameTextBox = QLineEdit(self) # Connect the textChanged signal of the text input field to the onNameChanged method nameTextBox.textChanged.connect(self.onNameChanged) # Create a label for displaying the result self.result = QLabel() # Add widgets to the vertical layout vbox.addWidget(nameLabel) vbox.addWidget(nameTextBox) vbox.addWidget(self.result) # Set the layout of the widget to the vertical layout self.setLayout(vbox) # Method called when the text in the name text input field changes def onNameChanged(self, text): # Set the text of the result label to the entered name self.result.setText(text) if __name__ == '__main__': # Create the application instance app = QApplication(sys.argv) # Create an instance of the RegistrationForm widget form = RegistrationForm() # Show the RegistrationForm widget form.show() # Start the application event loop sys.exit(app.exec_()) |
In the above example, we have created a QLineEdit widget and connect its textChanged signal to a custom slot onNameChanged. Whenever the user enters or modifies text in the Text Box, the slot is triggered, and we the label text to the TextBox text.
Run the code and this will be the result
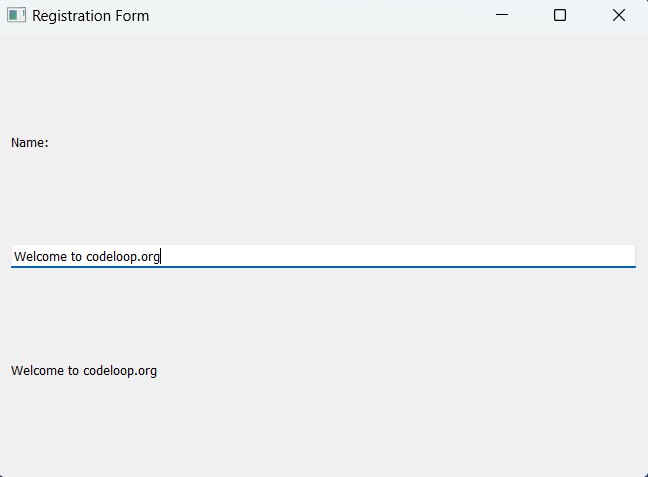
PyQt5 SpinBox
SpinBoxes are useful when you want users to input numeric values within a specified range. They provide an easy way to increase or decrease the value by using up and down arrow buttons or by directly typing in the value. you can use PyQt5 QSpinBox widget for this purpose.
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17 18 19 20 21 22 23 24 25 26 27 28 29 30 31 32 33 34 35 36 37 38 39 40 41 42 43 44 45 46 47 48 49 50 51 |
from PyQt5.QtWidgets import QApplication, QWidget, QVBoxLayout, QLabel, QSpinBox import sys class AgeForm(QWidget): def __init__(self): super().__init__() self.initUI() def initUI(self): # Set window title self.setWindowTitle('Age Form') # Create a vertical layout vbox = QVBoxLayout() # Create a label for the age field ageLabel = QLabel('Age:', self) # Create a spin box for selecting age ageSpinBox = QSpinBox(self) # Create a label for displaying the result self.result = QLabel() # Connect the valueChanged signal of the spin box to the onAgeChanged method ageSpinBox.valueChanged.connect(self.onAgeChanged) # Set minimum and maximum allowed values for the spin box ageSpinBox.setMinimum(0) ageSpinBox.setMaximum(120) # Add widgets to the vertical layout vbox.addWidget(ageLabel) vbox.addWidget(ageSpinBox) vbox.addWidget(self.result) # Set the layout of the widget to the vertical layout self.setLayout(vbox) # Method called when the value of the age spin box changes def onAgeChanged(self, value): # Set the text of the result label to the selected age self.result.setText(str(value)) if __name__ == '__main__': # Create the application instance app = QApplication(sys.argv) # Create an instance of the AgeForm widget form = AgeForm() # Show the AgeForm widget form.show() # Start the application event loop sys.exit(app.exec_()) |
In the above example, we have created a QSpinBox widget, we set its minimum and maximum values, and connect its valueChanged signal to the onAgeChanged slot. Whenever the user modifies the value in the Spin Box, the slot is invoked, and we show the value of spinbox in the label.
This will be the result
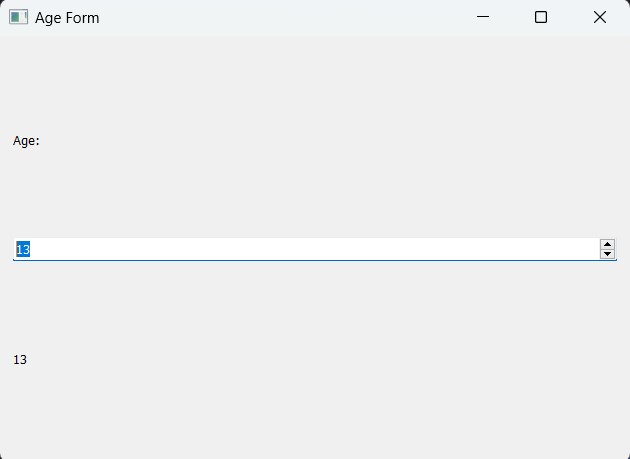
PyQt5 Slider
Sliders provide a visually appealing way to select values within a specified range. They are commonly used for volume control, brightness adjustments, or any other continuous value selection. you can use PyQt5 QSlider widget for this purpose.
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17 18 19 20 21 22 23 24 25 26 27 28 29 30 31 32 33 34 35 36 37 38 39 40 41 42 43 44 45 46 47 48 49 |
from PyQt5.QtWidgets import QApplication, QWidget, QLabel, QSlider, QVBoxLayout from PyQt5.QtCore import Qt import sys class VolumeControlForm(QWidget): def __init__(self): super().__init__() self.initUI() def initUI(self): # Set window title self.setWindowTitle('Volume Control') # Create label for volume control volumeLabel = QLabel('Volume:', self) volumeLabel.setAlignment(Qt.AlignCenter) # Create horizontal slider for adjusting volume volumeSlider = QSlider(Qt.Horizontal, self) volumeSlider.setRange(0, 100) # Connect slider's valueChanged signal to onVolumeChanged method volumeSlider.valueChanged.connect(self.onVolumeChanged) # Create label to display volume changes self.result = QLabel() # Create vertical layout and add widgets layout = QVBoxLayout() layout.addWidget(volumeLabel) layout.addWidget(volumeSlider) layout.addWidget(self.result) # Set layout for the widget self.setLayout(layout) # Method called when volume slider value changes def onVolumeChanged(self, value): # Display volume changes in the result label self.result.setText('Volume changed:' + str(value)) if __name__ == '__main__': # Create application instance app = QApplication(sys.argv) # Create instance of the VolumeControlForm widget form = VolumeControlForm() # Show the VolumeControlForm widget form.show() # Start the application event loop sys.exit(app.exec_()) |
In the above example, we have created a horizontal QSlider widget, set its range from 0 to 100, and connect its valueChanged signal to the onVolumeChanged slot. As the user changes the slider, the slot is triggered, and we add the value to the label.
This will be the result
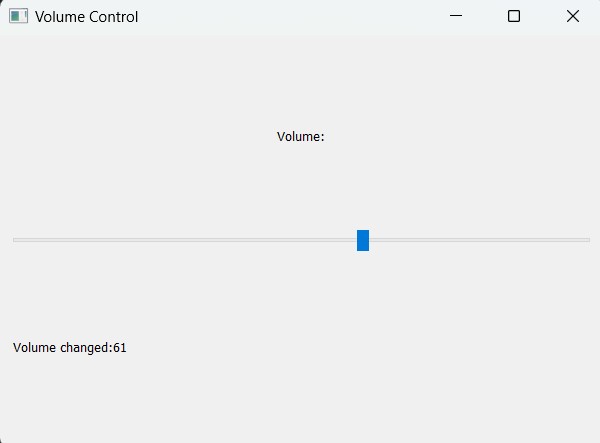
Subscribe and Get Free Video Courses & Articles in your Email