In this PyQt5 article i want to show you How To Draw Polygon In Python With PyQt5 (QPainter Class). QPainter class is is used for drawing different shapes in PyQt5. before this we had a complete introduction to QPainter class so you can check my previous articles in the below links.
This is the complete source code for How To Draw Polygon In Python With PyQt5 (QPainter Class)
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17 18 19 20 21 22 23 24 25 26 27 28 29 30 31 32 33 34 35 36 37 38 39 40 41 42 43 44 45 46 47 48 49 50 51 52 53 54 55 56 57 |
from PyQt5 import QtGui from PyQt5.QtWidgets import QApplication, QMainWindow import sys from PyQt5.QtGui import QBrush, QPen, QPainter, QPolygon from PyQt5.QtCore import QPoint, Qt class Window(QMainWindow): def __init__(self): super().__init__() # Window properties self.title = "Codeloop - PyQt5 Drawing Polygon" self.top = 200 self.left = 500 self.width = 400 self.height = 300 # Initialize window self.InitWindow() def InitWindow(self): # Set window icon self.setWindowIcon(QtGui.QIcon("codeloop.png")) # Set window title and geometry self.setWindowTitle(self.title) self.setGeometry(self.left, self.top, self.width, self.height) # Show window self.show() def paintEvent(self, event): # Define a QPainter object for painting painter = QPainter(self) # Set pen properties (color, width, style) painter.setPen(QPen(Qt.black, 5, Qt.SolidLine)) # Set brush properties (color, pattern) painter.setBrush(QBrush(Qt.red, Qt.VerPattern)) # Define polygon points points = QPolygon([ QPoint(10,10), QPoint(10,100), QPoint(100,10), QPoint(100,100) ]) # Draw polygon using the defined points painter.drawPolygon(points) # Create the application instance App = QApplication(sys.argv) # Create the main window window = Window() # Execute the application sys.exit(App.exec()) |
These are the imported classes that we are using in this article.
1 2 3 4 5 |
from PyQt5 import QtGui from PyQt5.QtWidgets import QApplication, QMainWindow import sys from PyQt5.QtGui import QBrush, QPen,QPainter, QPolygon from PyQt5.QtCore import QPoint, Qt |
So now this is our main Window class that extends from QMainWindow. and in the constructor of the class we need to initialize some window requirements.
1 2 3 4 5 6 7 8 9 10 11 12 |
class Window(QMainWindow): def __init__(self): super().__init__() self.title = "Codeloop - PyQt5 Drawing Polygon" self.top = 200 self.left = 500 self.width = 400 self.height = 300 self.InitWindow() |
In here we are going to set our window title, window icon and window geometry, also we need to show our window in here.
1 2 3 4 5 |
def InitWindow(self): self.setWindowIcon(QtGui.QIcon("icon.png")) self.setWindowTitle(self.title) self.setGeometry(self.left, self.top, self.width, self.height) self.show() |
So in QPainter class when we are going to do drawing we need to use paintEvent() method. in this method first we have created the QPainter class object and we have set the QBrush and QPen for our QPainter class and for drawing of QPolygon we need some QPoints and at the end we draw our polygon
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17 18 |
def paintEvent(self, event): painter = QPainter(self) painter.setPen(QPen(Qt.black, 5, Qt.SolidLine)) #painter.setBrush(QBrush(Qt.red, Qt.SolidPattern)) painter.setBrush(QBrush(Qt.red, Qt.VerPattern)) points = QPolygon([ QPoint(10,10), QPoint(10,100), QPoint(100,10), QPoint(100,100) ]) painter.drawPolygon(points) |
Run the complete code and this will be the result
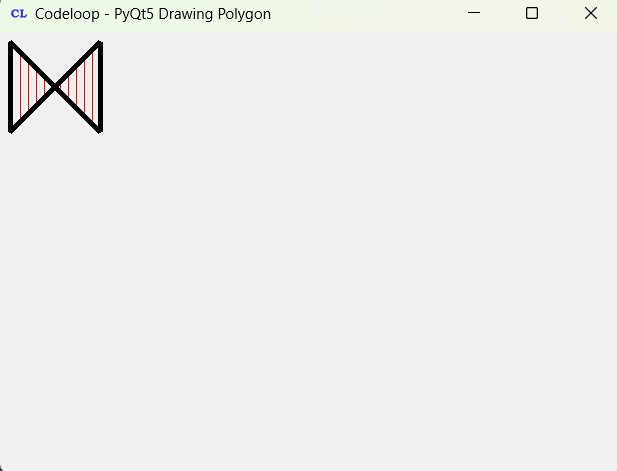
FAQs:
How do you draw a polygon in Python?
In Python, you can draw a polygon using different libraries such as PyQt5 or matplotlib. In PyQt5, you can use QPainter class to draw polygons.
How to add shape in PyQt5?
For adding shapes in PyQt5, you typically need a subclass of QWidget or QMainWindow and override paintEvent method. Inside the paintEvent method, you can use QPainter to draw different shapes such as rectangles, ellipses, polygons, lines, etc.
Subscribe and Get Free Video Courses & Articles in your Email
Hey Parwiz, please help me out. its been only one month that I have started learning Qt. Now i need to draw an arrow on an image using mouse click events. I know how to draw rectangle, ellipse, line using the available libraries, but my requirement is to start drawing the arrow once the mouse is pressed and end the drawing when the mouse is released. I hope my querry is clear. please please please help me out. I am stuck at this and tried several ways, but failed. Hope you l reply.