In this PyQt5 article iam going to show you Creating QCheckBox With Signals, also iam going to show you how you can create signals for your QCheckBox. so a QCheckBox is an option button that can be switched on (checked) or off (unchecked). Checkboxes are typically used to represent features in an application that can be enabled or disabled without affecting others. Different types of behavior can be implemented.
Also you can read more Python GUI articles in the below links
- Kivy GUI Development Tutorials
- TKinter GUI Development Tutorials
- Pyside2 GUI Development
- wxPython GUI Development Tutorials
- PyQt5 GUI Development Tutorials
First we need some imports
1 2 3 4 5 |
from PyQt5.QtWidgets import QApplication, QDialog, QCheckBox, QHBoxLayout, QGroupBox, QVBoxLayout, QLabel import sys from PyQt5 import QtGui from PyQt5.QtCore import QRect from PyQt5 import QtCore |
After that we are going to create our main Window class that extends from QDialog . and in the constructor of the class we need to initialize some requirements of the window . also we have called our initWindow() method in here.
1 2 3 4 5 6 7 8 9 10 |
class Window(QDialog): def __init__(self): super().__init__() self.title = "PyQt5 CheckBox" self.top = 200 self.left = 400 self.width = 400 self.height = 100 self.iconName = "icon.png" self.InitWindow() |
After that we are going to create our InitWindow() method. we set our window title, window icon and window geometry. also we have created the QVBoxLayout and QLable objects in here. we have also called our CreateLayout() method in here.
1 2 3 4 5 6 7 8 9 10 11 12 |
def InitWindow(self): self.setWindowIcon(QtGui.QIcon(self.iconName)) self.setWindowTitle(self.title) self.setGeometry(self.left, self.top, self.width, self.height) self.CreateLayout() vbox = QVBoxLayout() vbox.addWidget(self.groupBox) self.label = QLabel(self) self.label.setFont(QtGui.QFont("Sanserif",15)) vbox.addWidget(self.label) self.setLayout(vbox) self.show() |
In here we need to create our CheckBox, also we have created a group box, because we want to add the check boxes in the group box.
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17 18 19 20 21 22 23 |
def CreateLayout(self): self.groupBox = QGroupBox("What Is Your Favorite Programming Language ?") self.groupBox.setFont(QtGui.QFont("Sanserif",13)) hboxLayout = QHBoxLayout() self.check1 = QCheckBox("Python") self.check1.setIcon(QtGui.QIcon("pythonicon.png")) self.check1.setIconSize(QtCore.QSize(40,40)) self.check1.setFont(QtGui.QFont("Sanserif", 13)) self.check1.toggled.connect(self.onCheckbox_toggled) hboxLayout.addWidget(self.check1) self.check2 = QCheckBox("Java") self.check2.setIcon(QtGui.QIcon("java.png")) self.check2.setIconSize(QtCore.QSize(40, 40)) self.check2.setFont(QtGui.QFont("Sanserif", 13)) self.check2.toggled.connect(self.onCheckbox_toggled) hboxLayout.addWidget(self.check2) self.check3 = QCheckBox("C++") self.check3.setIcon(QtGui.QIcon("cpp.png")) self.check3.setIconSize(QtCore.QSize(40, 40)) self.check3.setFont(QtGui.QFont("Sanserif", 13)) self.check3.toggled.connect(self.onCheckbox_toggled) hboxLayout.addWidget(self.check3) self.groupBox.setLayout(hboxLayout) |
This is our method that we have already connected with the toggled signal of the QCheckBox.
1 2 3 4 5 6 7 |
def onCheckbox_toggled(self): if self.check1.isChecked(): self.label.setText(" You Have Selected: " + self.check1.text()) if self.check2.isChecked(): self.label.setText("You Have Selected : " + self.check2.text()) if self.check3.isChecked(): self.label.setText("You Have Selected : " + self.check3.text()) |
Also every PyQt5 application must create an application object.
1 |
app = QApplication(sys.argv) |
Finally, we enter the mainloop of the application. The event handling starts from this point.
1 2 |
window = Window() sys.exit(app.exec()) |
Complete source code for PyQt5 Creating QCheckBox With Signals
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17 18 19 20 21 22 23 24 25 26 27 28 29 30 31 32 33 34 35 36 37 38 39 40 41 42 43 44 45 46 47 48 49 50 51 52 53 54 55 56 57 58 59 60 61 62 63 64 65 66 67 68 69 70 71 72 73 74 75 76 77 78 79 80 81 82 83 84 85 86 |
from PyQt5.QtWidgets import QApplication, QDialog, QCheckBox, QHBoxLayout, QGroupBox, QVBoxLayout, QLabel import sys from PyQt5 import QtGui from PyQt5.QtCore import QRect from PyQt5 import QtCore class Window(QDialog): def __init__(self): super().__init__() # Window properties self.title = "Codeloop - PyQt5 CheckBox" self.top = 200 self.left = 400 self.width = 400 self.height = 100 self.iconName = "icon.png" # Initialize window self.InitWindow() def InitWindow(self): # Set window properties self.setWindowIcon(QtGui.QIcon(self.iconName)) self.setWindowTitle(self.title) self.setGeometry(self.left, self.top, self.width, self.height) # Create layout self.CreateLayout() # Create label for displaying selection vbox = QVBoxLayout() vbox.addWidget(self.groupBox) self.label = QLabel(self) self.label.setFont(QtGui.QFont("Sanserif",15)) vbox.addWidget(self.label) self.setLayout(vbox) self.show() def CreateLayout(self): # Create group box for checkboxes self.groupBox = QGroupBox("What Is Your Favorite Programming Language ?") self.groupBox.setFont(QtGui.QFont("Sanserif",13)) hboxLayout = QHBoxLayout() # Create checkboxes self.check1 = QCheckBox("Python") self.check1.setIcon(QtGui.QIcon("python.png")) self.check1.setIconSize(QtCore.QSize(40,40)) self.check1.setFont(QtGui.QFont("Sanserif", 13)) self.check1.toggled.connect(self.onCheckbox_toggled) hboxLayout.addWidget(self.check1) self.check2 = QCheckBox("Java") self.check2.setIcon(QtGui.QIcon("java.png")) self.check2.setIconSize(QtCore.QSize(40, 40)) self.check2.setFont(QtGui.QFont("Sanserif", 13)) self.check2.toggled.connect(self.onCheckbox_toggled) hboxLayout.addWidget(self.check2) self.check3 = QCheckBox("C++") self.check3.setIcon(QtGui.QIcon("cpp.png")) self.check3.setIconSize(QtCore.QSize(40, 40)) self.check3.setFont(QtGui.QFont("Sanserif", 13)) self.check3.toggled.connect(self.onCheckbox_toggled) hboxLayout.addWidget(self.check3) # Set layout for group box self.groupBox.setLayout(hboxLayout) def onCheckbox_toggled(self): # Update label based on checkbox selection if self.check1.isChecked(): self.label.setText(" You Have Selected: " + self.check1.text()) if self.check2.isChecked(): self.label.setText("You Have Selected : " + self.check2.text()) if self.check3.isChecked(): self.label.setText("You Have Selected : " + self.check3.text()) if __name__ == "__main__": app = QApplication(sys.argv) window = Window() sys.exit(app.exec()) |
This code creates a simple PyQt5 application with a dialog window that contains a group box with three checkboxes. Each checkbox represents a programming language (Python, Java, and C++). When a checkbox is checked, a label below the checkboxes updates to display the selected programming language. The application’s window has a title, icon, and fixed size, and it closes when the user exits the application.
Run the complete code and this will be the result
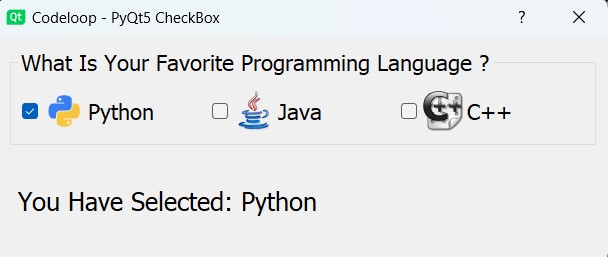
FAQs:
What is a QCheckBox in PyQt5?
A QCheckBox is a widget in PyQt5 that allows users to toggle between two states: checked and unchecked. It is commonly used in GUI applications to represent binary choices or options that can be enabled or disabled.
How do I create a QCheckBox in PyQt5?
For creating a QCheckBox in PyQt5, you can use QCheckBox class and optionally set properties such as text, icons and initial checked state. After that you can add the checkbox to a layout or container widget to display it inside your application.
How do I handle signals emitted by QCheckBox widgets in PyQt5?
Signals emitted by QCheckBox widgets, such as the toggled signal for state changes, can be connected to custom slots or functions using the connect() method. This allows you to execute specific actions in response to checkbox state changes.
What are signals and slots in PyQt5?
Signals and slots are a mechanism for communication between objects in PyQt5. Signals are emitted by objects to indicate that a particular event has occurred, such as a state change in a QCheckBox. Slots are functions or methods that can be connected to signals and executed in response to those events.
Subscribe and Get Free Video Courses & Articles in your Email