In this Python Object Oriented Programming article we are going to learn about Mastering Object-Oriented Programming in Python, Python is an Object Oriented Programming (OOP) . what it means that we can solve a problem in Python language by creating objects in our programming. in this article we are going to discuss some OOPs concepts like classes, objects and also some main principles in OOP like Inheritance, Polymorphism, Encapsulation and Abstraction. this is a little advance tutorial for Python basics tutorial you can click on the below link.
What is Python Object Oriented Programming ?
Python Object-Oriented Programming (OOP) is programming paradigm that focuses on the use of objects and classes to organize and structure code. It is programming approach that enables programmers to create reusable and modular code that is easier to maintain, modify and test.
In Python objects are instances of classes, which are user defined data types that encapsulate data and behavior. each object has its own set of attributes (data) and methods (behavior), which can be accessed and manipulated through the object’s interface.
Python’s OOP features include encapsulation, inheritance, and polymorphism. Encapsulation allows objects to hide their implementation details and expose only the necessary information through public interface. Inheritance allows classes to inherit attributes and methods from parent class and it allows programmers to reuse code and create hierarchies of related classes. Polymorphism allows objects to take on multiple forms and it allows them to respond differently to the same message based on their type.
Python OOP features makes it powerful and flexible programming language that is suitable for different types of applications, from web development to data science and machine learning. by using OOP principles, programmers can create code that is easier to maintain, extend, and debug, leading to more efficient and effective software development, in this Mastering Object-Oriented Programming in Python we will cover different practical examples on this concept.
Also Read:
1: What Are Python Objects
Object In Real World:
Objects are something that we can sense, feel and manipulate, you can look around you and see many examples of real world objects like your dog, your cat, or human being these are the real world examples for Objects. all Objects shares two characteristics, they all have attributes and they all have behaviors. for example dogs have attributes like (name, color) and behaviors like (barking, fetching).
Objects In Software Development:
Objects in software development are models of somethings that can do certain things and have certain things done to them. formally objects are collection of data and associated behaviors.
2: What is Python class
in Object Oriented Programming (OOP) a class is a blueprint for the objects, also a class is a way of organizing information about type of data so a programmer can reuse elements when making multiple instances of that data type for example, if a programmer wanted to make three instances of car,maybe a BMW, Ferrari, and a Ford instance.the car class would allow the programmer to store similar information that is unique to each car (they are different models, and maybe different colors.) and associate the appropriate information with each car.
Let’s say an example for Python OOP
An example of a class is the class Persons. In general Persons usually have a name and age; these are instance attributes. Person can also can walk and eat; this is a method.
When you talk about a specific person, you would have an object in programming: an object is an instantiation of a class. This is the basic principle on which object-oriented programming is based.
Creating class in Python
1 2 3 |
class Person: def __init__(self): pass |
Note: self in Python is equivalent to this in C++ or Java.
Instantiating Python Objects:
To instantiate an object, type the class name, followed by two brackets. You can assign this to a variable to keep track of the object. In this case, you have a (mostly empty) Person class, but no object yet. Let’s create one!
1 |
parwiz = Person() |
Adding Attributes to Python Class:
We can add attributes to our class, let’s create our class again
1 2 3 4 5 6 7 8 9 |
class Person: def __init__(self, name, age): self.name = name self.age = age person = Person("Parwiz",25) print(person.name) print(person.age) |
Run the code you will receive this output
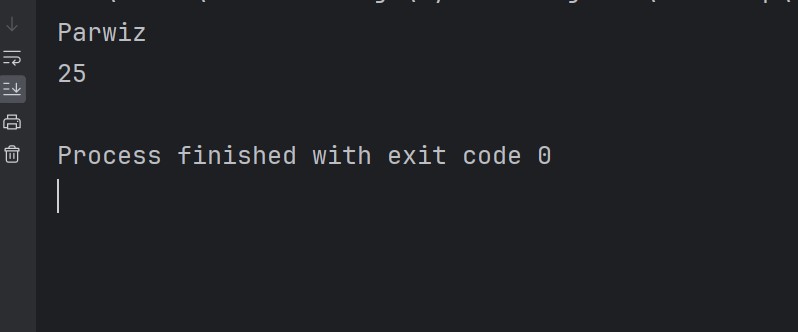
Defining methods in Python class
Now that you have a Person class, it does have a name and age which you can keep track of, but it doesn’t actually do anything. This is where instance methods come in. You can rewrite the class to now include a printDetails() method. Notice how the def keyword is used again, as well as the self argument.
1 2 3 4 5 6 7 8 9 10 11 12 |
class Person: def __init__(self, name, age): self.name = name self.age = age def printDetails(self): print("This is details") person = Person("Parwiz", 25) print(person.name) print(person.age) person.printDetails() |
Your will receive this output in IDE
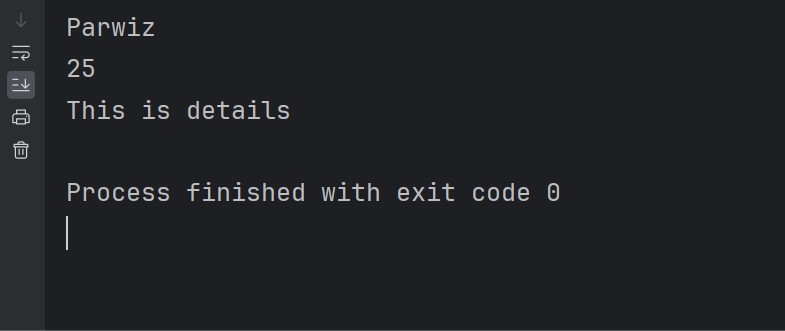
Principles of Python Object Oriented Programming
There are some main principles for Object Oriented Programming let’s talk one by one about these principles.
- Python Encapsulation:
In python we can restrict the access to methods and variables of a class, so this preventing data from direct modification is called encapsulation, and in python we denote the private attributes by using single _ or double __
Note: Python does not enforce you for the encapsulation.
Let’s give a practical example in Python
1 2 3 4 5 6 7 8 9 10 11 12 13 |
class Vehicle: def __init__(self): pass def Driving(self): print("Driving The Car") def __PrintDetails(self): print("Print Details") v = Vehicle() v.Driving() # can access to this method v.PrintDetails() # can not access to this method |
So in the above example we have implemented encapsulation we have make private PrintDetails() method Now our class object just can access to Driving, not PrintDetails()
- Python Polymorphism:
We are going to start with a real world example, as you know we use milk for drinking but milk can also be used to make curd, butter and etc so the term polymorphism means to use something in different forms, in software development to maintain the code and to maintain the simplicity of the code we use the concept of polymorphism the very popular examples are method overloading and method overriding.
method overloading is used in a single class where you have the same function name but different set of arguments for each function.
And method overriding is a concept one comes across while making subclasses, for example we know that a child class can inherits all the methods and attributes from the base class, however In some situations the inherited method from the base class does not fit in to child class, on that situation we need to remplement the method in the child class Now for this we can method overriding
Let’s take an example in python
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17 18 19 20 21 22 23 24 |
class A: def __init__(self, name, lastname): self.name = name self.lastname = lastname def PrintDetails(self): print(" My name is " + self.name + " and my lastname is " + self.lastname) class B(A): def __init__(self, name, lastname, email): self.name = name self.lastname = lastname self.email = email def PrintDetails(self): print(" My name is " + self.name + " and my lastname is " + self.lastname + " and my email is " + self.email) a = A("John", "Doe") a.PrintDetails() b = B("Parwiz", "Forogh", "par@gmail.com") b.PrintDetails() # super for invoking the intializer of parent class in to child class |
This is the output
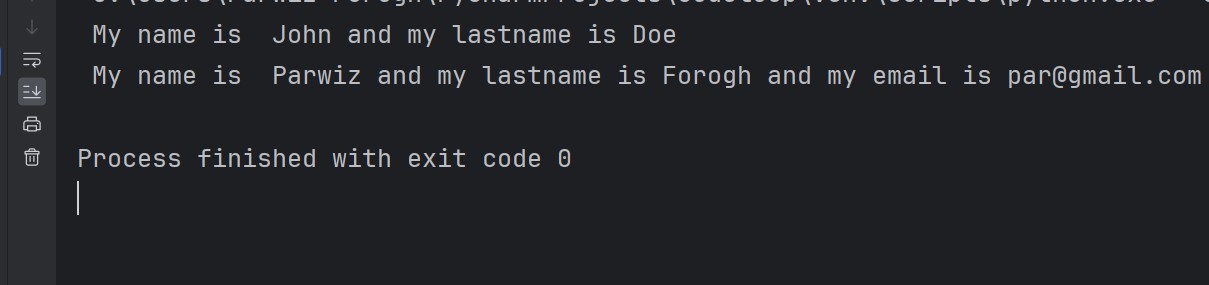
- Python Abstraction:
In object oriented design abstraction means that the details are hidden from the outside world. abstraction mechanism hide internal implementation details, and it should only reveal operations relevant for other objects for example suppose we are driving a car so in driving we only need to know about the basic functionality of the car like how steering works but we don’t need to know about the internal working of the car so in software development there are some information which is not important for users. So that kind of information is made private by the programmers. now we can tell abstraction is a mechanism with the help of that programmers hide the unnecessary details from the users.
- Python Inheritance:
Inheritance is the most famous, relationship in object-oriented programming. Inheritance is sort of like a family tree. For example my father inherited some characteristics from my grandfather and i inherited some characteristic from my father. In object-oriented programming one class can inherit attributes and methods from another class. So in inheritance we have the concept of base class and derived class
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17 18 19 |
class Person: def __init__(self, name, age): self.name = name self.age = age def PrintDetails(self): print("My Name Is " + self.name, "And My Age Is " + str(self.age)) class Student(Person): pass st1 = Student("Parwiz", 24) # st1.FullName() st1.PrintDetails() p1 = Person("John", "Doe") p1.PrintDetails() |
In the above example we have created two classes Person class is our Base class and Student class is our derived class. Now this Student class can access to all attributes and methods of our base class
Multiple Inheritances in Python:
If a class inherits more than one class we can call it multiple inheritances.
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17 |
class A: def move(self): print(" Moving….. ") class B(A): def eat(self): print(" Eating….. ") class C(A, B): pass c = C() c.move() c.eat() |
In the above class we have two base classes, and our C class inherits from A and B this is called multiple inheritances. C class can access to all methods and attributes of the class A and class B.
Multi Level Inheritances in Python:
In Python when you inherit a derived class from another derived class, this is called multi level inheritance, in python multi level Inheritance can be done at any depth
Hierarchical Inheritances in Python:
In python object oriented programming when a base class derived from more than one class than that is called hierarchical inheritance.
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17 18 19 20 21 22 |
class Animal: def run(self, name): self.name = name print(self.name + " is running ") class Dog(Animal) def walk(self, name): self.name = name print(" Dog is walking ") class Cat(Animal) pass dog = Dog() dog.run("Dog") dog.walk() cat = Cat() cat.run("Cat") |
FAQs:
What is Python object-oriented programming?
Object-oriented programming (OOP) in Python is a programming paradigm that cover the concept of objects, objects are instances of classes. OOP focuses on organizing code into reusable and modular components called classes, which encapsulate data (attributes) and behavior (methods). In Python, OOP facilitates code reusability, modularity and abstraction, also it allows developers to efficiently manage complex software systems.
What is object-oriented programming with example?
Object-oriented programming (OOP) is a programming paradigm based on the concept of objects, which are instances of classes. In Python, you can define classes to represent real-world entities or abstract concepts, you can encapsulate attributes and methods that define their behavior. This is a simple example of OOP in Python:
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 |
class Car: def __init__(self, make, model): self.make = make self.model = model def display_info(self): return f"This car is a {self.make} {self.model}" # Creating instances of the Car class car1 = Car("Toyota", "Camry") car2 = Car("Honda", "Civic") # Calling the display_info() method on each instance print(car1.display_info()) # Output: This car is a Toyota Camry print(car2.display_info()) # Output: This car is a Honda Civic |
Can I learn OOP in Python?
Yes, Python is an excellent language for learning object-oriented programming (OOP) concepts, because it is simple and has readable syntax. There are alot of resources available, including tutorials, books and online course, to help you learn OOP in Python easily.
What are the 4 basics of OOP? The four basics of object-oriented programming (OOP) are:
- Encapsulation: The bundling of data (attributes) and methods (functions) that operate on the data into a single unit called a class.
- Inheritance: The mechanism by which a class can inherit attributes and methods from another class, and it makes code reusability and creates hierarchical relationships.
- Polymorphism: The ability of objects to take on different forms or behaviors depending on their context. Polymorphism allows objects of different classes to be treated as objects of a common superclass.
- Abstraction: The process of hiding the implementation details of a class and exposing only the essential features to the outside world. Abstraction simplifies complex systems by focusing on essential characteristics and ignoring irrelevant details.
Subscribe and Get Free Video Courses & Articles in your Email