In this Python TKinter article i want to show you How to Create ProgressBar in Python TKinter. first of let’s talk about TKinter ProgressBar, but you will have a question in your mind, that Is Tkinter the only GUI for Python? the answer is No, if you are Python developer, for creating a GUI in Python, you have different options, for example PyQt6, wxPython, PySide6 and also Kivy. so now let’s talk about progressbar in Python.
What is TKinter ProgressBar?
How to Create ProgressBar In Python TKinter?
For creating a ProgressBar in Python Tkinter, you can use ttk.Progressbar widget from the ttk module. This is a simple example of how to create and use a ProgressBar:
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17 18 19 20 21 22 23 24 25 26 27 28 29 30 31 32 33 34 35 36 37 38 39 40 41 42 43 44 45 46 47 48 49 50 51 52 53 54 55 56 57 58 59 60 61 62 63 64 |
from tkinter import * from tkinter import ttk import time class Root(Tk): def __init__(self): super(Root, self).__init__() # Set title of the window self.title("Codeloop - Tkinter ProgressBar") # Set window icon self.iconphoto(True, PhotoImage(file='codeloop.png')) # Create label frame to group the progress bar buttons self.buttonFrame = ttk.LabelFrame(self, text="Progress Bars") self.buttonFrame.grid(column=0, row=0) # Call method to create the progress bar and buttons self.progressBar() def progressBar(self): # Create buttons to control the progress bar self.button1 = ttk.Button(self.buttonFrame, text="Run Progress Bar", command=self.run_progressbar) self.button1.grid(column=0, row=0) self.button2 = ttk.Button(self.buttonFrame, text="Start Progress Bar", command=self.start_progressbar) self.button2.grid(column=0, row=1) self.button3 = ttk.Button(self.buttonFrame, text="Stop Immediately", command=self.stop_progressbar) self.button3.grid(column=0, row=2) # Create the progress bar widget self.progress_bar = ttk.Progressbar(self, orient='horizontal', length=286, mode='determinate') self.progress_bar.grid(column=0, row=3, pady=2) def run_progressbar(self): # Configure the progress bar maximum value self.progress_bar["maximum"] = 100 # Update progress bar value in a loop for i in range(101): # Simulate a process delay time.sleep(0.05) self.progress_bar["value"] = i # Update the progress bar appearance self.progress_bar.update() # Reset the progress bar value after completion self.progress_bar["value"] = 0 def start_progressbar(self): # Start the progress bar animation self.progress_bar.start() def stop_progressbar(self): # Stop the progress bar animation immediately self.progress_bar.stop() # Create an instance of the Root class root = Root() root.mainloop() |
These are the imports that we need for this article, basically it is tkinter library, also we are going to import ttk from tkinter with time library from Python.
1 2 3 |
from tkinter import * from tkinter import ttk import time |
So at the top we have created our Root class that extends from TK, and we have added our window requirements like title, size and icon in the constructor of the class.
1 2 3 4 5 6 7 8 9 10 11 |
class Root(Tk): def __init__(self): super(Root, self).__init__() self.title("Tkinter Progress Bar") self.minsize(640, 400) self.iconbitmap('icon.ico') self.buttonFrame = ttk.LabelFrame(self, text="Progress Bars") self.buttonFrame.grid(column=0, row=0) self.progressBar() |
In this method we create three buttons with ProgressBar, and i want to give some functionality to these buttons. i want when a user click on run the progressbar should be run in the stop it should be stopped.
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17 |
def progressBar(self): self.button1 = ttk.Button(self.buttonFrame, text = "Run Progress Bar", command = self.run_progressbar) self.button1.grid(column =0, row = 0) self.button2 = ttk.Button(self.buttonFrame, text = "Start Progress Bar", command = self.start_progressbar) self.button2.grid(column = 0, row = 1) self.button3 = ttk.Button(self.buttonFrame, text="Stop Immediatly", command = self.stop_progressbar) self.button3.grid(column=0, row=2) self.progress_bar = ttk.Progressbar(self, orient = 'horizontal', length = 286, mode = 'determinate') self.progress_bar.grid(column = 0, row = 3, pady =2) |
And these are the methods for the functionality of our progressbar.
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17 18 19 |
def run_progressbar(self): self.progress_bar["maximum"] = 100 for i in range(101): time.sleep(0.05) self.progress_bar["value"] = i self.progress_bar.update() self.progress_bar["value"] = 0 def start_progressbar(self): self.progress_bar.start() def stop_progressbar(self): self.progress_bar.stop() |
Run the complete code and this will be the result
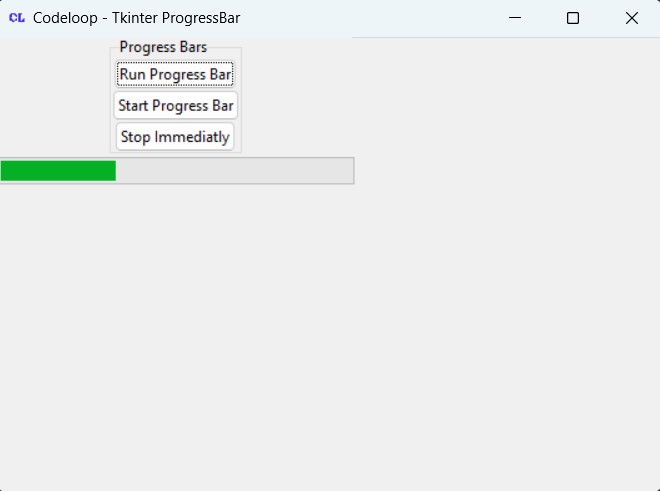
Subscribe and Get Free Video Courses & Articles in your Email
Thanks a lot for your videos. I’ve been finally starting to understand Tkinter better after watching your videos. But there’s something I can’t manage to figure out: how do I make the progress bar stop once it reaches 100? Because in the video, it continues to move until it I press ‘stop’, but what if I wanted it to stop if it also reached the maximum value?
you can check the max size, if it goes to hundred you can make the value to zero or stop progressbar