In this lesson we want to learn How to Create ComboBox in Python Tkinter, Python Tkinter Combobox is a drop-down list that allows users to select a value from a list. The list of values is defined by the “values” option, and the currently selected value is stored in the “textvariable” option. The Combobox widget is a combination of an Entry widget and a Listbox widget.
This is an example of how to create a Tkinter Combobox:
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17 18 19 20 |
import tkinter as tk import tkinter.ttk as ttk root = tk.Tk() root.geometry("200x200") # create a variable to store the selected value selected = tk.StringVar() # create the combobox combobox = tk.ttk.Combobox(root, textvariable=selected) combobox.grid(row=0, column=0) # set the values for the combobox combobox["values"] = ["Option 1", "Option 2", "Option 3"] # set a default value for the combobox combobox.set("Option 1") root.mainloop() |
Run the complete code and this will be the result
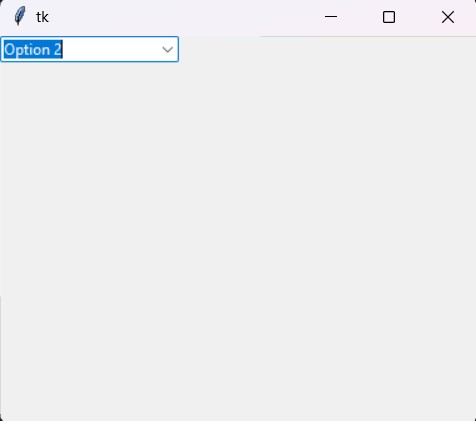
This is an example of how you can add a label that displays the selected item from the Combobox:
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17 18 19 20 21 22 23 24 |
import tkinter as tk import tkinter.ttk as ttk root = tk.Tk() root.geometry("200x200") # create a variable to store the selected value selected = tk.StringVar() # create the combobox combobox = tk.ttk.Combobox(root, textvariable=selected) combobox.grid(row=0, column=0) # set the values for the combobox combobox["values"] = ["Option 1", "Option 2", "Option 3"] # set a default value for the combobox combobox.set("Option 1") # create a label to display the selected item label = tk.Label(root, textvariable=selected) label.grid(row=1, column=0) root.mainloop() |
In this code, I’ve added a label after the Combobox, and set its textvariable to be the same as the Combobox’s textvariable. This means that whenever the user selects a new item from the Combobox, the label will automatically update to display the selected item.
Run the code and this will be the result
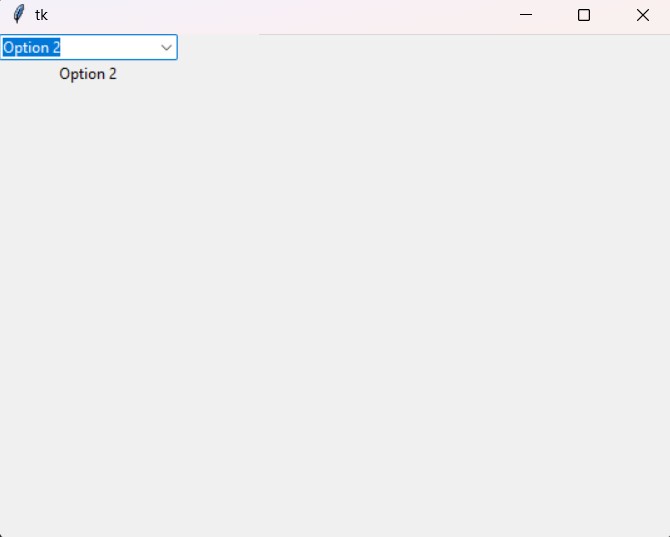
You can also add a function to update the label when you change the combobox selection using the command attribute of the combobox, like this:
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17 18 19 20 21 22 23 24 25 26 27 28 29 |
import tkinter as tk import tkinter.ttk as ttk root = tk.Tk() root.geometry("200x200") # create a variable to store the selected value selected = tk.StringVar() # create a label to display the selected item label = tk.Label(root, textvariable=selected) label.grid(row=1, column=0) def on_select(selected): label.configure(text=selected.get()) # create the combobox combobox = tk.ttk.Combobox(root, textvariable=selected, command=on_select(selected)) combobox.grid(row=0, column=0) # set the values for the combobox combobox["values"] = ["Option 1", "Option 2", "Option 3"] # set a default value for the combobox combobox.set("Option 1") root.mainloop() |
Subscribe and Get Free Video Courses & Articles in your Email