In this Python Tutorial we are going to learn How to Create Classes in Python, in this article we want to cover different concepts like creating classes, instantiating classes, adding methods and attributes to python class and working with class and instance attributes in Python.
Introduction to Python Classes
First of all let’s have a few words about Python Classes, so basically we can say that a class is a blueprint for the objects, or by using a class we can organize information about a type of data. by creating a class we can reuse elements when making multiple instances of that data type for example we create a class Animal, now if we want to create the instances of this class, it may be a Cat or Dog or another type of Animal. the Animal class would allow you to store similar information that is unique to each animal(they are different colors and etc.) and associate the appropriate information with each animal.
How to Create Classes in Python?
OK let’s create a simple class, you can use class keyword followed by the name of the class in Python. in the example we want to creat a simple class at name of MyClass, right now we are not adding anything the class body.
1 2 |
class MyClass: pass |
Now If you want to use this class you need to instantiate the objects from this class. creating of instance class is simple, you can just give the name of the class followed by pair of parenthesis and after that our instances will be created.
1 2 3 4 5 6 |
class MyClass: pass a = MyClass() b = MyClass() |
Read More
Adding Attributes in Python Classes
So now we want to add some attributes to our Python Classes, we can say that attributes are Python variables, right now we don’t want to add anything in the body of class, but we want to add some attributes to a created object of the class. we have created a simple class, and after that we have instantiated two objects from the class, after that we have assigned some attributes to the class using dot notation.
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 |
class Employee: pass emp1 = Employee() emp2= Employee() emp1.name = "John" emp1.age = 30 emp2.name = "Codeloop" emp2.age = 25 print(emp1.name, emp1.age) print(emp2.name, emp2.age) |
Run the code and this is the result.
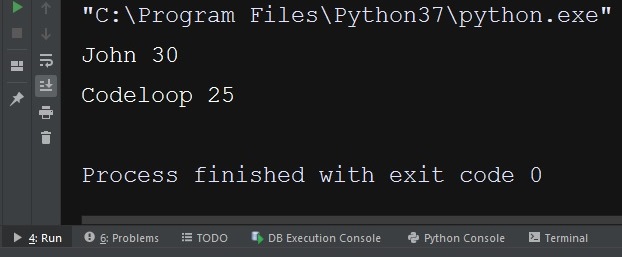
Python Initializer Method
Most OOP Programming Languages have the concept of a constructor, a special method that creates and initializes the object when it is created, now in python we have initializer method this is a special method and it is called when an object is created from a class and it allows the class to initialize the attributes of the class.
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17 |
class Employee: def __init__(self, name, age): self.name = name self.age = age emp1 = Employee("John", 30) emp2= Employee("Codeloop", 25) print(emp1.name) print(emp1.age) print(emp2.name) print(emp2.age) |
In the above example we have created an initializer method, and the self represents the instance of the class. By using the “self” keyword we can access the attributes and methods of the class in python, when you want to create the instance of the class you need to pass the name and age in the class.
Run the code and this is the result.
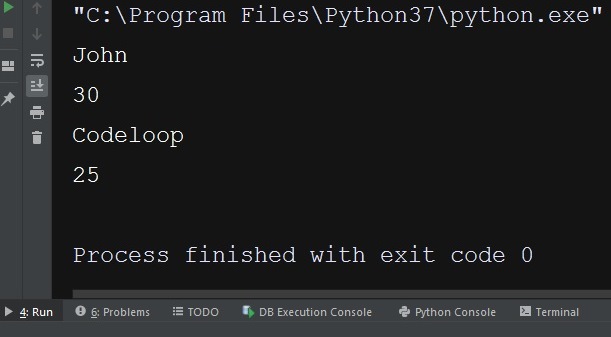
Adding Methods in Python Class
Let’s add methods in our Python Classes, in this example we have added a full_details() method in our class and we are going to print the information in this class.
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 |
class Employee: def __init__(self, name, age, email): self.name = name self.age = age self.email = email def full_details(self): print(f"My name is {self.name} My Age is " f"{self.age} and My email is {self.email} ") emp = Employee("John", 25, "john@gmail.com") emp.full_details() |
Run the code and this is the result.
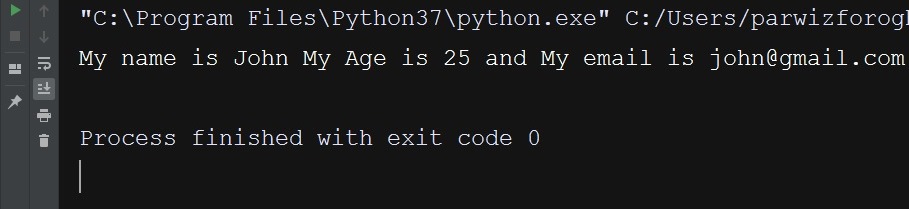
Now let’s talk about Class and Instance Attributes in Python.
Python Class Attribute
A class attribute is a Python variable that is belong to a class rather than a particular object. It is shared between all the objects of the class and it is defined outside the initializer method of the class.
Python Instance Attribute
An instance attribute is a Python variable belonging to only one object. This variable is only accessible in the scope of this object and it is defined inside the init() method also Instance Attributes are unique to each object.
Let’s create an example, if you see in our class we have three attributes or variables, our email attribute is a class attributes because it is out side the __init__() method, and also it is shared by all instances. When you change the value of a class attributes, it will affect all instances that share the same exact value. the variable name and age are instance variable, because they are unique to the instance, if you change one instance variable, the second instance variable will not be affected. but in class attribute when you change the variable, it will affect all the instances.
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17 18 19 |
class Employee: email = 'codeloop@gmail.com' def __init__(self, name, age): self.name = name self.age = age emp1 = Employee("Codeloop", 20) emp2 = Employee('John', 25) print(emp1.name) print(emp1.age) print(emp1.email) print(emp2.name) print(emp2.age) print(emp2.email) |
FAQs:
What are the classes in Python?
Classes in Python are blueprints for creating objects. They define the structure and behavior of objects by encapsulating attributes (data) and methods (functions) that operate on those attributes. Classes enable you to create objects with specific properties and functionalities, using classes your code is more organized, reusable and easier to maintain.
What is __init__ in Python?
In Python, __init__ is a special method (also known as a constructor), and it is automatically called when a new instance of a class is created. It initializes the object’s attributes or performs any necessary setup operations. By defining an __init__ method inside class, when you create this constructor, you are specifying how objects of that class should be initialized.
What is the difference between object and class in Python?
- Class: A class in Python is a blueprint or template for creating objects. It defines the structure and behavior of objects by encapsulating attributes (data) and methods (functions) that operate on those attributes. Classes serve as a blueprint for creating multiple instances (objects) with similar properties and behaviors.
- Object: An object in Python is an instance of a class. It represents a specific entity or instance created using the blueprint defined by the class. Objects have their own unique state (attributes) and behavior (methods), but they share the structure and behavior defined by their class. Each object is an independent instance with its own set of attributes and can interact with other objects and the rest of the program.
How do you create a class in Python?
For creating a class in Python, you use the class keyword followed by the class name and a colon. Inside the class block, you define attributes and methods that belong to the class.
How to add a class to Python?
Adding a class to Python is easy, you can just define a new class using the class keyword along with a unique class name. You can define the class in Python code or module, and after that you can use that by importing the module or script where the class is defined.
What is a class method in Python?
A class method in Python is a method that is bound to the class rather than the instance of the class. It is defined using @classmethod decorator and takes the class itself (often denoted as cls) as its first parameter, instead of the instance (self). Class methods can access and modify class-level variables and are commonly used for tasks that involve the class itself, such as creating new instances or accessing class attributes.
Is Python a hard class?
The difficulty of learning Python, or any programming language may be different depending on individual ability, prior experience and the resources available for learning. Python is often considered one of the easier programming languages to learn, because it is simple and has readable syntax, we can say that it is like pseudo-code, also Python has large community, and there are alot of resources that a beginner level person can use from that.
Is Python free for students?
Yes, Python is free and open-source for everyone, including students. Python’s creator, Guido van Rossum, implemented an open-source license for Python, known as the Python Software Foundation License (PSF). This license allows users to freely use, modify, and distribute Python for any purpose.
Subscribe and Get Free Video Courses & Articles in your Email