In this Python Tutorial we are going to talk about Class Inheritance in Python, also we are going to talk about about different types of python class inheritance like multiple inheritance, multi level inheritance and hierarchical inheritance.
What is Python Class Inheritance ?
Class inheritance is one of the most important concepts in Object Oriented Programming, basically a class inheritance allows us to create a relationship between two or more classes. now in object oriented programming one class can inherit attributes and methods from another class.
In this image you can see that we have two classes, the first one is our Class People and we have some variables with one method in this class, in term of class inheritance we can call this class a Base Class, Parent Class or Super class. we have another Class Student, now this class extends or inherit from Class People, and for this class we can call Derived Class, Child Class or Sub Class. as we have already said when a class extends from the the base class, the derived class can access to all attributes and methods of the base class. for example in here our Class Student child class can access to the fname and lname attributes of the Class People super class.
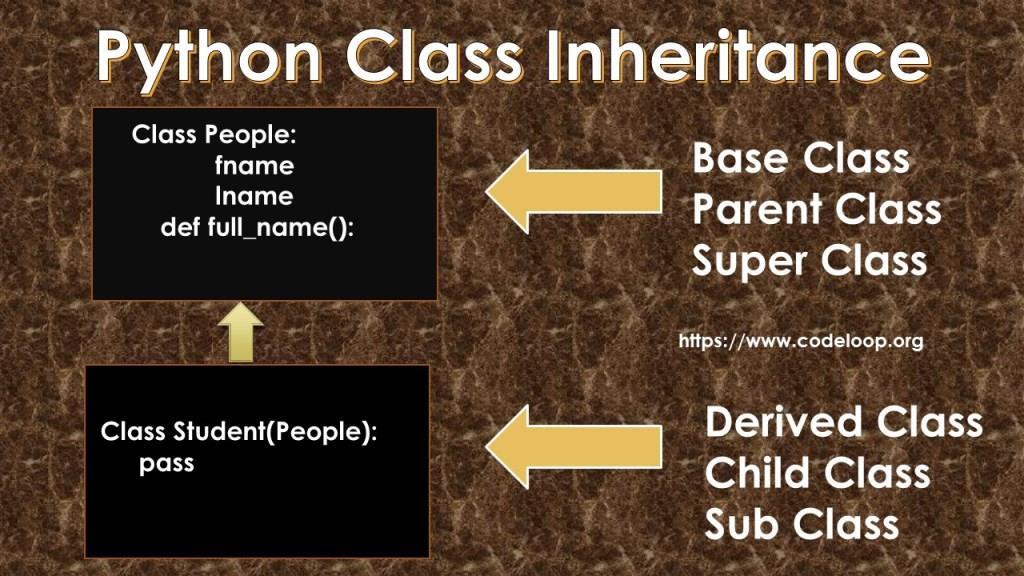
Now we want to create a practical example in Python Class Inheritance.
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17 18 19 20 21 |
class Employee: def __init__(self, name, email): self.name = name self.email = email def full_info(self): print(f'Name Is {self.name} And Email Is' f' {self.email}') class Male(Employee): pass m1 = Male("Parwiz", 'par@gmail.com') m2 = Male("codeloop", 'codeloop@gmail.com') m1.full_info() m2.full_info() |
So in the above code we have a base class and we have a derived class, in the base class we have some instance attributes and a method for printing the variables. now our derived class can access to all attributes and method of the base class, for example Male class can access to the name, email variables and also full_info() method.
Run the code and this will be the result.
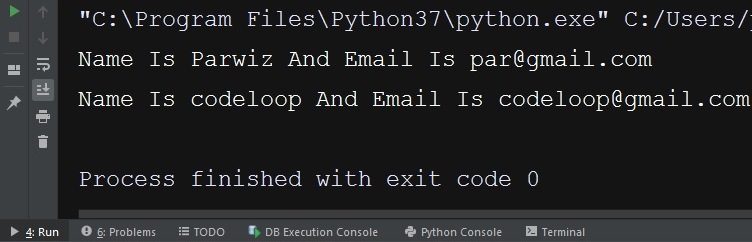
Learn More
- How to Create Classes in Python
- Python Google Translate API
- How to Convert Text to Speech in Python
- Convert PY File to EXE
- PyQt5 QTableWidget Tutorial: Create a Dynamic Table
So there are different types of class inheritance in Python, in this section we want to learn about Python Multiple Inheritance, Python Multi Level Inheritance and Python Hierarchical Inheritance.
Python Multiple Inheritance
If a class extends from more than one class, that is called multiple inheritance.
Let’s take a look at the image example.
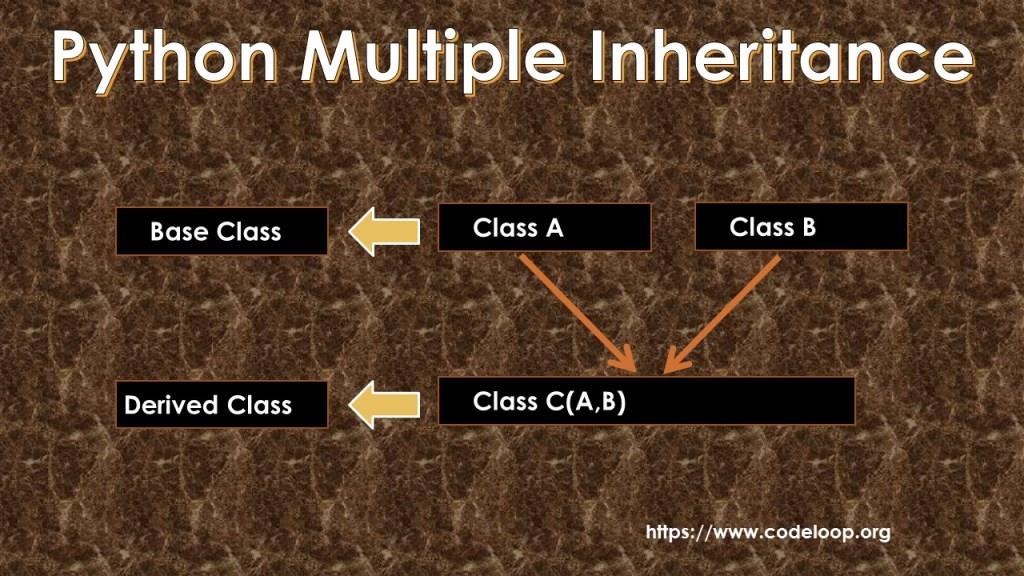
In the above example we have three classes, we have two base classes that are Class A and Class B. we have another class at name of Class C, this class extends from Class A and Class B and that is our derived class. as we have already said that if a class extends from more than one class, that is called multiple inheritance. in here our C Class extends from two base classes, now the C class can access to all attributes and methods of the Class A and Class B.
So this is our practical example in Python Multiple Inheritance, In the this example we have two base classes and the third class is extending from these two base class, and if you see in our base classes we have methods, after extending Class C can access to these two methods.
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17 |
class A: def a_method(self): print("A method from class A") class B: def b_method(self): print("B method from Class B") class C(A,B): pass c = C() c.a_method() c.b_method() |
Let’s create another example, in this example we want to override a method in to our base classes, also we are going to talk about Method Resolution Order (MRO).
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 |
class A: def full_name(self): print("This is my full name - Class A") class B: def full_name(self): print("This is my full name - Class B") class C(B, A): pass c = C() c.full_name() |
You can see that when we are extending our Class C, we have the order of B and A, now it will first check the method in the C class, if it does not find the method in the C class, than it checks the B Class, now it means that the order matters in Python Multiple Inheritance, if we run this code you will see that first we have our B Class method.
This is the result.
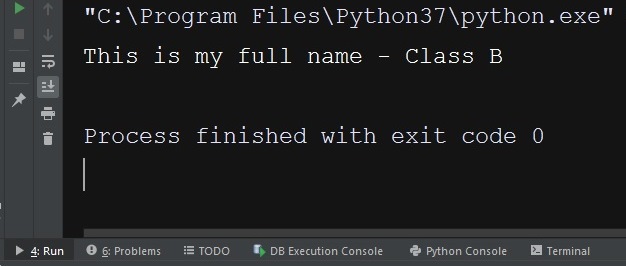
Python Multi Level Inheritance
In this part we want to talk about Python Multi Level Inheritance, in Object Oriented Programming when you inherits a derived class from another derived class this is called multi level inheritance and it can be done at any depth.
Let’s see this image example.
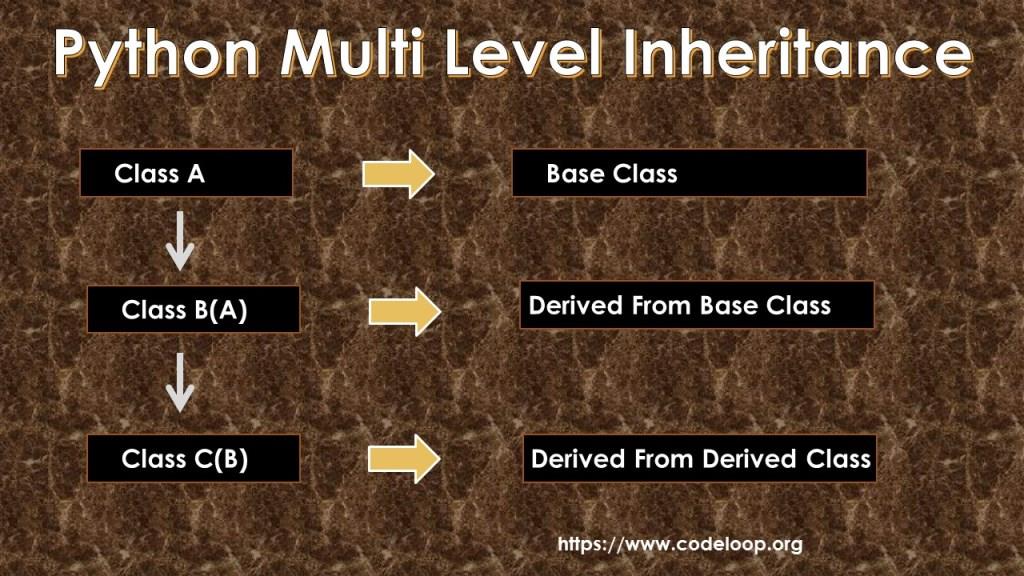
In the above image we have three classes, we have a Class A, this class is our base class. we have another Class B, this class extends from the base Class A. we have another Class C, now this class extends from Class B. as we have already said when you extends a derived class from another derived class this is called multi level inheritance and it can be done at any depth. now our Class B can access to all attributes and method of the Class A, and our Class C can access to all attributes and methods of the Class A and Class B.
Now we want to create an example in Python Multi Level Inheritance, so in this example we have three classes, class Person is our base class, class Student is extending from the Person class, so it became a derived class for Person class, we have another Programmer class, this class extends from the Student class, now if you create the instance of the Programmer class, this class can access to the method of the Person and Student classes.
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17 18 19 20 |
class Person: def per_method(self): print("Iam a Person") class Student(Person): def stu_method(self): print("Iam a Student") class Programmer(Student): pass pro = Programmer() pro.per_method() pro.stu_method() |
You can see that in the above example we have instantiated our Programmer class, and we don’t have any method in this class, but this class can access to the method of Person and Student class, run the code and this is the result.
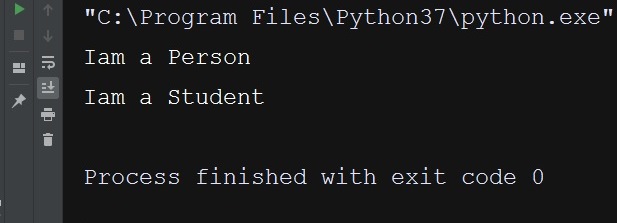
Python Hierarchical Inheritance
In this section we want to learn about Python Hierarchical Inheritance, in Object Oriented Programming When more than one derived classes are created from a single base class that is called hierarchical inheritance.
Let’s take a look at this image example.
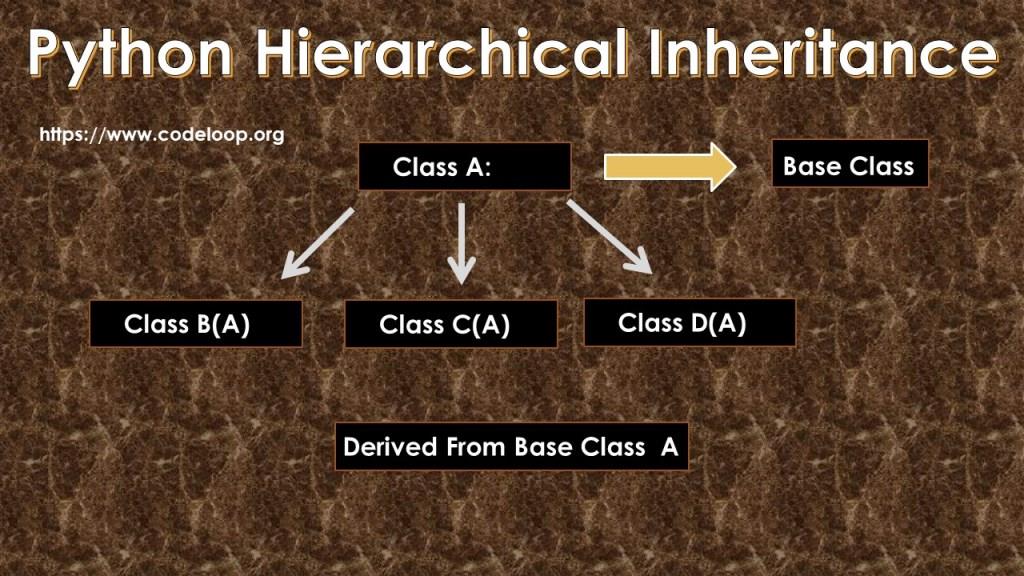
In the above image, we have four classes, Class A is our base or parent class, we have another three classes and all of them are extending from just one base class, as i have already said when more than one derived classes are created from a single base class that is called hierarchical inheritance. now there is a relationship between Class A and three derived classes(B, C, D), but there is no relationship between the derived classes, it means that we have a relationship between base class and derived classes, but there is no relationship between derived classes, for example we don’t have any relationship between Class B, Class C and Class D.
Now let’s create practical example in Python Hierarchical Inheritance, In the example we have three python classes, class Animal is our base class and we have two more classes that are inheriting from just one base class. we have relationship between Class Animal and Class Cat, also between Class Animal and Class Dog, but there is no relationship between Class Cat and Class Dog.
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17 18 19 20 21 22 23 |
class Animal: def run(self, name): self.name = name print(f'{self.name} Is Runing') class Dog(Animal): pass class Cat(Animal): pass dog = Dog() dog.run("Dog") cat = Cat() cat.run("Cat") |
FAQs:
How do you write a class inheritance in Python?
To write a class inheritance in Python, you define a new class that inherits attributes and methods from another class, and we can call that superclass or base class. You specify the superclass in parentheses after the class name. for example:
1 2 3 4 5 6 7 |
class Animal: def speak(self): print("Animal speaks") class Dog(Animal): # Dog class inherits from Animal class def bark(self): print("Dog barks") |
How do you check inheritance in Python?
In Python, you can check inheritance using the issubclass() function. This function takes two arguments: the class you want to check and the potential superclass. If the first class is a subclass of the second class, it returns True; otherwise it returns False. This is an example:
1 2 3 4 5 6 7 |
class Animal: pass class Dog(Animal): pass print(issubclass(Dog, Animal)) # Output: True |
What is base class and derived class in Python?
In Python, a base class (or superclass) is the class that we inherit attributes and methods from that class. Also derived class (or subclass) is a class that inherits from another class, and it extends its functionality or customizing its behavior. The derived class can add new attributes or methods, or override existing ones inherited from the base class.
Which statement correctly creates a class using inheritance?
The correct statement to create a class using inheritance in Python is:
1 2 |
class DerivedClassName(BaseClassName): # Class definition goes here |
What is class inheritance in Python?
Class inheritance in Python is mechanism in which we can inherits attributes and methods from another class, and that is called superclass or base class, the inherited class is called subclass or derived class. Inheritance allows the subclass to reuse code from the superclass, and this functionality create code reusability and enable hierarchical relationships between classes.
What are the 5 types of inheritance in Python?
In Python, there are five types of inheritance:
- Single Inheritance: A subclass inherits from only one superclass.
- Multiple Inheritance: A subclass inherits from multiple superclasses.
- Multilevel Inheritance: A subclass inherits from a superclass, and another subclass inherits from the first subclass.
- Hierarchical Inheritance: Multiple subclasses inherit from the same superclass.
- Hybrid Inheritance: A combination of two or more types of inheritance.
Can you inherit from two classes in Python?
Yes, Python supports multiple inheritance, which means a class can inherit attributes and methods from more than one superclass. You can specify multiple superclasses separated by commas in the class definition. However, you need to be careful with multiple inheritance to avoid potential issues like method name clashes and the diamond problem. This is an example of multiple inheritance in Python:
1 2 3 4 5 6 7 8 |
class SuperClass1: pass class SuperClass2: pass class SubClass(SuperClass1, SuperClass2): pass |
Subscribe and Get Free Video Courses & Articles in your Email