In this Python TKinter article i want to show How To Add Legends In TKinter Matplotlib Window. so first of all let’s talk about TKinter and Matplotlib.
What is TKinter?
Tkinter is standard Python library, and it is used to build graphical user interfaces (GUIs). The name of Tkinter comes from Tk interface, and it provides Python bindings for Tk GUI toolkit. Tkinter is included with most Python installations, and you don’t need to install TKinter. using TKinter you can create desktop applications for different platforms, including Windows, macOS and Linux.
What Is Matplotlib ?
Matplotlib is a Python 2D plotting library which produces publication quality figures in a variety of hardcopy formats and interactive environments across platforms. Matplotlib can be used in Python scripts, the Python and IPython shells, the Jupyter notebook, web application servers, and four graphical user interface toolkits.
How to Install Matplotlib?
You can install matplotib using pip, open your command prompt or terminal and write this command.
1 |
pip install matplotlib |
How to Add Legends in TKinter Matplotlib Window
So now this is the complete code for this article
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17 18 19 20 21 22 23 24 25 26 27 28 29 30 31 32 33 34 35 36 37 38 39 40 41 42 43 44 45 46 47 48 49 50 51 52 53 54 55 56 57 58 59 60 61 62 63 64 65 66 67 68 69 70 |
from tkinter import * from matplotlib.figure import Figure from matplotlib.backends.backend_tkagg import FigureCanvasTkAgg, NavigationToolbar2Tk class Root(Tk): def __init__(self): super(Root, self).__init__() self.title("Codeloop.org - Tkinter Adding Legends") # Set window title # Set window icon self.iconphoto(True, PhotoImage(file='codeloop.png')) # Call method to create the Matplotlib canvas self.matplotCanvas() def matplotCanvas(self): # Create Figure object fig = Figure(figsize=(12, 5), facecolor='white') # Add subplot to the figure axis = fig.add_subplot(111) # Define some sample data for the plot xValues = [1, 2, 3, 4] yValues0 = [6, 7.5, 8, 7.5] yValues1 = [5.5, 6.5, 8, 6] yValues2 = [6.5, 7, 8, 7] # Plot data on the subplot t0, = axis.plot(xValues, yValues0) t1, = axis.plot(xValues, yValues1) t2, = axis.plot(xValues, yValues2) # Set labels for the axes axis.set_ylabel('Vertical Label') axis.set_xlabel('Horizontal Label') # Add grid to the plot axis.grid() # Create handles and labels for the legend handles_labels = [(t0, 'First line'), (t1, 'Second line'), (t2, 'Third Line')] handles, labels = zip(*handles_labels) # Add legend to the plot axis.legend(handles, labels, loc='upper right') # Create a FigureCanvasTkAgg object canvas = FigureCanvasTkAgg(fig, self) # Draw the canvas canvas.draw() # Pack the canvas widget to the bottom of the window canvas.get_tk_widget().pack(side=BOTTOM, fill=BOTH, expand=True) # Create a NavigationToolbar2Tk object toolbar = NavigationToolbar2Tk(canvas, self) # Update the toolbar toolbar.update() # Pack the canvas widget to the top of the window canvas._tkcanvas.pack(side=TOP, fill=BOTH, expand=True) # Create an instance of the Root class root = Root() root.mainloop() |
First of all we have imported our required classes that we need, basically we need the class from tkinter library along with matplotlib library.
1 2 3 |
from tkinter import * from matplotlib.figure import Figure from matplotlib.backends.backend_tkagg import FigureCanvasTkAgg, NavigationToolbar2Tk |
So this is the method that we are going to create our Matplotlib canvas, with our data that we want to plot also in this method we create the legends.
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17 18 19 20 21 22 23 24 25 26 27 28 29 30 31 32 33 34 35 36 37 38 39 40 41 42 43 44 45 46 47 48 49 |
def matplotCanvas(self): # Create Figure object fig = Figure(figsize=(12, 5), facecolor='white') # Add subplot to the figure axis = fig.add_subplot(111) # Define some sample data for the plot xValues = [1, 2, 3, 4] yValues0 = [6, 7.5, 8, 7.5] yValues1 = [5.5, 6.5, 8, 6] yValues2 = [6.5, 7, 8, 7] # Plot data on the subplot t0, = axis.plot(xValues, yValues0) t1, = axis.plot(xValues, yValues1) t2, = axis.plot(xValues, yValues2) # Set labels for the axes axis.set_ylabel('Vertical Label') axis.set_xlabel('Horizontal Label') # Add grid to the plot axis.grid() # Create handles and labels for the legend handles_labels = [(t0, 'First line'), (t1, 'Second line'), (t2, 'Third Line')] handles, labels = zip(*handles_labels) # Add legend to the plot axis.legend(handles, labels, loc='upper right') # Create a FigureCanvasTkAgg object canvas = FigureCanvasTkAgg(fig, self) # Draw the canvas canvas.draw() # Pack the canvas widget to the bottom of the window canvas.get_tk_widget().pack(side=BOTTOM, fill=BOTH, expand=True) # Create a NavigationToolbar2Tk object toolbar = NavigationToolbar2Tk(canvas, self) # Update the toolbar toolbar.update() # Pack the canvas widget to the top of the window canvas._tkcanvas.pack(side=TOP, fill=BOTH, expand=True) |
These are the values that we want to plot
1 2 3 4 |
xValues = [1, 2, 3, 4] yValues0 = [6, 7.5, 8, 7.5] yValues1 = [5.5, 6.5, 8, 6] yValues2 = [6.5, 7, 8, 7] |
These are the labels for our Matplotlib.
1 2 |
axis.set_ylabel('Vertical Label') axis.set_xlabel('Horizontal Label') |
This is the legends for our Matplotlib.
1 |
fig.legend((t0, t1, t2), ('First line', 'Second line', 'Third Line '), 'upper right') |
Run the complete code and this will be the result
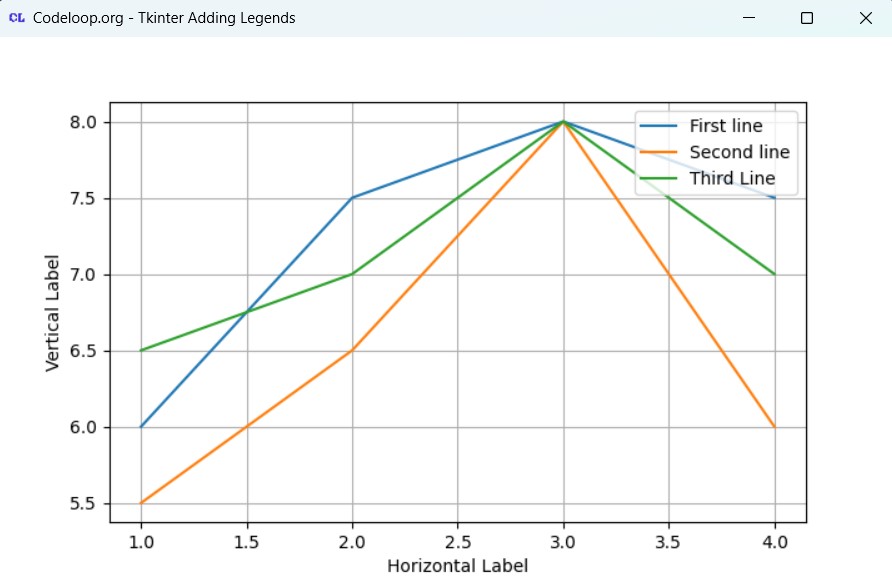
Subscribe and Get Free Video Courses & Articles in your Email