This is our twenty first tutorial in Django, in this tutorial we are going to learn about Django Models Creating Customs Methods. before we have saw that how you can use built in methods in Django Models, for example in your Django Models when you use def __str__ , this is a built in method. with Django Models you can add your own custom methods. for example i have an Article model and i want to shorten the description text, so for this we are using our own methods.
Flask Web Development Tutorials
1: Flask CRUD Application with SQLAlchemy
2: Flask Creating News Web Application
3: Flask Creating REST API with Marshmallow
Python GUI Development Tutorials
1: PyQt5 GUI Development Tutorials
2: Pyside2 GUI Development Tutorials
3: wxPython GUI Development Tutorials
4: Kivy GUI Development Tutorials
5: TKinter GUI Development Tutorials
So now we need to create a New Project in Django, also for django installation and creating New Project you can read this article Django Introduction & Installation. but you can use this command for creating of the New Project.
1 |
django-admin startproject MyProject |
After creating of the Django Project, you need to migrate your project.
make sure that you have changed the directory to the created project.
1 |
python manage.py migrate |
So after doing this, let’s create our Django App, i have already talked about
Django App in one of my articles, you can check this Django Apps & URL Routing.
1 |
python manage.py startapp MyApp |
After creation of the App, you need to add the created App in your settings.py file
INSTALLED_APP section.
1 2 3 4 5 6 7 8 9 |
INSTALLED_APPS = [ 'django.contrib.admin', 'django.contrib.auth', 'django.contrib.contenttypes', 'django.contrib.sessions', 'django.contrib.messages', 'django.contrib.staticfiles', 'MyApp', ] |
Now open your models.py in your MyApp App, and add your model, basically we have
an Article model with three fields, and the important point is that we have added our
own custom method ShortenText() in our model.
in the method we are going to just return the 100 character of our body field text.
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17 18 |
from django.db import models # Create your models here. class Article(models.Model): title = models.CharField(max_length=30) body = models.TextField() date = models.DateTimeField(auto_now_add=True) def __str__(self): return self.title #custom method added for the body def ShortenText(self): return self.body[:100] |
After creating of the models, we need to migrate our project, first you need to do
migrations and after that migrate.
1 |
python manage.py makemigrations |
Now you need to migrate.
1 |
python manage.py migrate |
OK now let’s just add our model to the Django admin panel, you can read this article for django super user Django Creating Super User. open your admin.py file and add this code.
1 2 3 4 5 6 7 |
from django.contrib import admin from .models import * # Register your models here. admin.site.register(Article) |
Before adding our html files, we need to create our views, so open your views.py file and add this code for creating a simple view. basically in this view we are going to get our all articles from the database.
1 2 3 4 5 6 7 8 9 10 11 |
from django.shortcuts import render from .models import Article # Create your views here. def Index(request): article = Article.objects.all() context = {'article':article} return render(request, "index.html", context) |
Now we are going to add our html files, make a templates folder in your working directory.
make sure that after creating of the templates, you need to add that in your settings.py file.
1 2 3 4 |
TEMPLATES = [ { 'BACKEND': 'django.template.backends.django.DjangoTemplates', 'DIRS': ["templates"], # templates name added here |
This is our base.html file, for django template inheritance you can read
this article Django Template Inheritance.
1 2 3 4 5 6 7 8 9 10 11 12 13 |
<!DOCTYPE html> <html lang="en"> <head> <meta charset="UTF-8"> <title>{% block title %} {% endblock %}</title> </head> <body> {% block body %} {% endblock %} </body> </html> |
And this is our index.html file.
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17 18 19 20 |
{% block title %}Articles {% endblock %} {% block body %} <h1>This is articles page</h1> {% for articles in article %} <h3>{{articles.title}}</h3> <h1>{{articles.ShortenText}}</h1> <h4>{{articles.date}}</h4> {% endfor %} {% endblock %} |
You can see that for the body we have used our {{articles.ShortenText}}.
Also you need to create your urls, you can read this article for url routing Django Apps & URL Routing . but just create a new python file in your MyApp app at name of urls.py and add these codes.
1 2 3 4 5 6 |
from django.urls import path from .views import * urlpatterns = [ path('', Index, name = 'index'), ] |
And also you need to include your app urls.py in your project urls.py file.
1 2 3 4 5 6 7 |
from django.contrib import admin from django.urls import path, include urlpatterns = [ path('admin/', admin.site.urls), path('', include('MyApp.urls')), ] |
Now you can run your Django Project.
1 |
python manage.py runserver |
First you need to open your Django Admin Panel and add some data. i have already added some data like this. you can see that for the body text, i have added a long text, because i want to make this shorter using my custom method that i have already created in my models.py file.
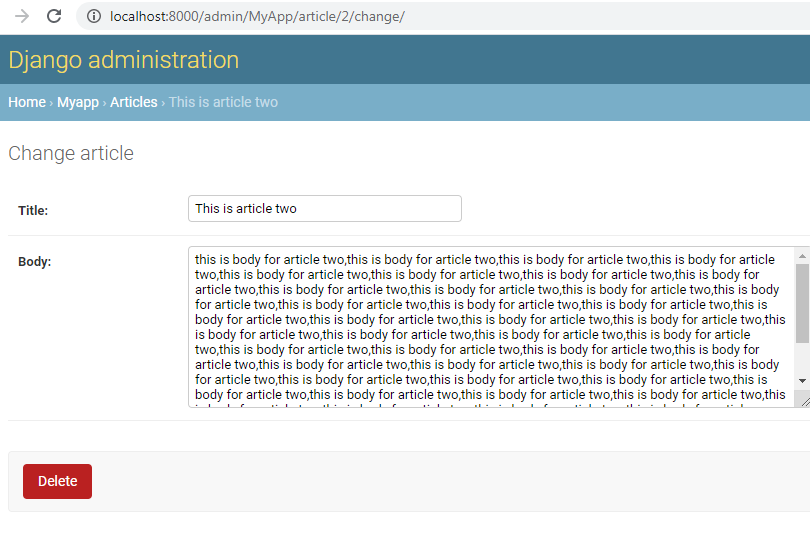
Now if you go to http://localhost:8000/ , you can see that we have just 100
character of body text.
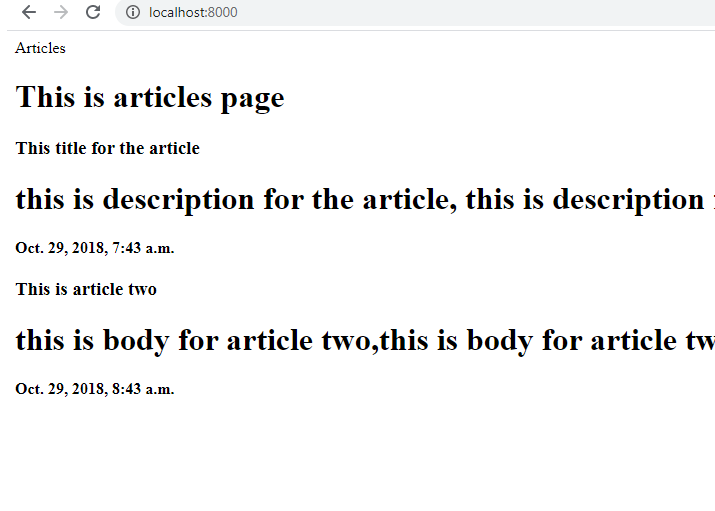
Subscribe and Get Free Video Courses & Articles in your Email