This is our twenty second tutorial in Django, in this tutorial we are going to learn about Django Abstract Base Class Model Inheritance. there are different types of Model Inheritance in Django, but we are going to just talk about Abstract Base Class Model Inheritance , also if you are interested in Flask Web Development, you can check the below links.
Flask Web Development Tutorials
1: Flask CRUD Application with SQLAlchemy
2: Flask Creating News Web Application
3: Flask Creating REST API with Marshmallow
Python GUI Development Tutorials
1: PyQt5 GUI Development Tutorials
2: Pyside2 GUI Development Tutorials
3: wxPython GUI Development Tutorials
4: Kivy GUI Development Tutorials
5: TKinter GUI Development Tutorials
What is Django Model Inheritance ?
Model inheritance in Django works almost identically to the way normal class inheritance
works in Python, but with a difference that in Django Model Inheritance you need to
extends from django.db.models.Model.
What is Django Abstract Base Class Model Inheritance ?
According to Django Documentation Abstract base classes are useful when you want to put some common information into a number of other models. You write your base class and put abstract=True
in the Meta class. This model will then not be used to create any database table. Instead, when it is used as a base class for other models, its fields will be added to those of the child class.
So now we need to create a New Project in Django, also for django installation and creating New Project you can read this article Django Introduction & Installation. but you can use this command for creating of the New Project.
1 |
django-admin startproject MyProject |
After creating of the Django Project, you need to migrate your project. make sure
that you have changed the directory to the created project.
1 |
python manage.py migrate |
So after doing this, let’s create our Django App, i have already talked about Django
App in one of my articles, you can check this Django Apps & URL Routing.
1 |
python manage.py startapp MyApp |
After creation of the App, you need to add the created App in your settings.py file
INSTALLED_APP section.
1 2 3 4 5 6 7 8 9 |
INSTALLED_APPS = [ 'django.contrib.admin', 'django.contrib.auth', 'django.contrib.contenttypes', 'django.contrib.sessions', 'django.contrib.messages', 'django.contrib.staticfiles', 'MyApp', ] |
Now open your models.py in your MyApp App, and add your model.
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17 18 19 20 21 22 23 24 25 |
from django.db import models # Create your models here. class ContactInfo(models.Model): name = models.CharField(max_length=30) email = models.EmailField(max_length=30) address = models.CharField(max_length=30) class Meta: abstract = True class Customer(ContactInfo): phone = models.CharField(max_length=30) class Staff(ContactInfo): position = models.CharField(max_length=30) |
So in this model the ContactInfo model cannot be used as a normal Django model,
since it is an abstract base class. It does not generate a database table or have a manager,
and cannot be instantiated or saved directly. but our Customer and Staff models will have 4 fields.
because the three fields are extended from our ContactInfo model. and there will not be any database table for ContactInfo model, but for Customer and Staff we have database tables.
After creating of the models, we need to migrate our project, first you need to do
migrations and after that migrate.
1 |
python manage.py makemigrations |
You can see that django created just two database models, we don’t have database
model for our ContactInfo model.
1 2 3 4 |
Migrations for 'MyApp': MyApp\migrations\0003_customer_staff.py - Create model Customer - Create model Staff |
Now you need to migrate.
1 |
python manage.py migrate |
This is the output in the terminal.
1 2 3 |
Apply all migrations: MyApp, admin, auth, contenttypes, sessions Running migrations: Applying MyApp.0003_customer_staff... OK |
Also you can add your Model in Django Admin Panel, so first of all create a superuser, you can read this article for creating Django SuperUser Django Admin Panel , after that open your admin.py file and add your models.
1 2 3 4 5 6 7 8 |
from django.contrib import admin from .models import Customer, Staff # Register your models here. admin.site.register(Customer) admin.site.register(Staff) |
Now run your Django Project.
1 |
python manage.py runserver |
Go to http://localhost:8000/admin/, you can see that we have just our two models,
and if you open the model you will see four fields.
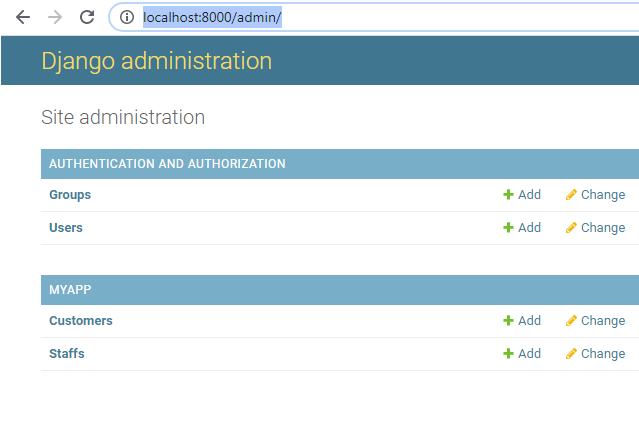
Subscribe and Get Free Video Courses & Articles in your Email
Hi, first, great article. Second I would like to know how to send/order the fields of the abstract class at the end of the child class. Any hint are more than appreciated.