Python is one of the most powerful programming languages, and it has become one of the best choices for beginners and professional developers. Its simplicity, readability, and extensive libraries make it an ideal language to learn for anyone interested in computer programming. If you wantto build websites, analyze data, develop games, or automate tasks, Python can pave the way for endless possibilities. In this beginner guide, we’ll take a journey to explore the fundamentals of Python programming.
Introduction to Python (Beginner Guide to Learn Python Programming)
We already mentioned that Python is dynamic and powerful programming language, and it has gained a lot of popularity in recent years. because it has simple syntax, extensive libraries and broad applications, also Python is one of the best choice for beginners, now let’s talk more about Python, and also we will Learn Python Step by Step.
What is Python and why is it popular?
So Python is high level, interpreted programming language that was first released in 1991. It was created by Guido van Rossum and it has since gained a lot of popularity among developers. Python design philosophy emphasizes code readability and simplicity, and this makes it easy to learn and use.
These are some reasons why Python has become popular:
-
- Simplicity and Readability: Python has a clean and easy syntax that is like English, and this makes it easy to read and write. This simplicity helps beginners learn programming concepts quickly and allows experienced developers to be more productive.
-
- Large and Active Community: Python has a large a community of developers who contribute to its growth. This community provides extensive support, documentation, and different third party libraries, and this makes it easier to find solutions to problems and accelerate development.
- Cross-Platform Compatibility: Python is a cross platform language, which means that Python programs can run on different operating systems without requiring major modifications. This portability is advantageous for developing applications that need to run on multiple platforms.
- Powerful Libraries: Python has a rich ecosystem of libraries and frameworks that simplify complex tasks. For example, libraries like NumPy, pandas and Matplotlib are widely used for data analysis and visualization, also TensorFlow and PyTorch are popular for machine learning and deep learning.
- Integration and Extensibility: Python can easily integrate with other languages such as C, C++ and Java. This capability allows developers to leverage existing code and libraries written in other languages, and it enhance Python capabilities and performance.
- Job Market and Career Opportunities: Python popularity has led to a growing demand for Python developers. Many companies and industries, including tech giants and startups are using Python for different applications, and it offer nice job opportunities for Python programmers.
Installing Python on different platforms (Windows, macOS, Linux)
Now let’s talk that how we can install Python for different platforms, first let’s start from Window.
Windows:
- Visit the official Python website at python.org/downloads.
- On the Downloads page, click on the Download Python button for the latest stable release.
- Scroll down to the Files section and select the Windows installer appropriate for your system (32-bit or 64-bit).
- Run the downloaded installer.
- On the first page of the installer, select the option to Add Python to PATH and then click Customize installation if you want to customize the installation location.
- Click Next and follow the prompts to complete the installation.
macOS:
- Visit the official Python website at python.org/downloads.
- On the Downloads page, click on the Download Python button for the latest stable release.
- Scroll down to the Files section and select the macOS installer package.
- Run the downloaded package, and the installer should open.
- Follow the prompts in the installer, and make sure to select the option to Install for all users to ensure Python is available system wide.
- Complete the installation process by following the prompts.
Linux:
- Most Linux distributions come with Python pre installed. For checking if Python is already installed, open a terminal and type python3 –version or python –version. If Python is not installed, you can proceed with the installation.
- Open a terminal and enter the following command to update the package lists:
1 |
sudo apt update |
Next, install Python by running this command:
1 |
sudo apt install python3 |
After installing Python, you can run Python programs by opening a terminal or command prompt and typing python3 (or python for Python 2) followed by the name of the code you want to execute.
Also take a note that some Linux distributions may have different package managers or package names for Python. The above instructions are general guidelines, and you may need to adapt them based on your specific distribution.
Writing your first “Hello, World” program
So in the above sections, we have learned how we can install Python, now it is time to create our first Hello World code in Python programming language, first of all, you need to open your favorites like PyCharm or VSCode, or even you can Python IDE itself, writing your first Hello World code in Python is so simple and easy, add this code in your file.
1 |
print("Hello World") |
This code uses the print() function in Python to display the message Hello World on the console. print() function is a built-in function in Python that takes an argument (in this case, the string “Hello World”) and prints it as output. When the program is executed, the message Hello World is displayed on the console.
This will be the result

Python Variables and Data Types
So we have learned about the basics and fundamental concepts of Python, In this section of Learn Python step by step, we want to talk about Python variables, we will cover different topics on Python variables, for example how we can create variables in Python, we will talk about naming convention, data types and many more.
Understanding Python Variables and their Naming Conventions
Variables play an important role in Python programming, because it allows us to store and manipulate data. In addition to understanding how variables work in Python, it is essential to follow proper naming conventions to write clean and readable code.
What are Variables in Python ?
In Python a variable is a symbolic name that represents a memory location used to store data. Unlike some other programming languages, Python is dynamically typed, which means you don’t need to declare the type of a variable explicitly. Instead, the type is inferred based on the value assigned to the variable.
Declaring and Assigning Values to Python Variables:
In Python, you can declare a variable by simply assigning a value to it using the ‘=’ operator. For example, In here we have declared two variables, name and age, and assigned them the values geekscoders and 25.
1 2 |
name = "codeloop" age = 25 |
Naming Conventions for Python Variables:
So proper naming conventions is an important factor for writing clean and maintainable Python code. These are some commonly accepted practices for naming variables in Python:
- Use descriptive and meaningful names: Choose names that accurately reflect the purpose or content of the variable. This makes the code more readable and self explanatory. Example: total_sales, customer_name
- Use lowercase letters with words separated by underscores: This convention is known as snake_case. It enhances readability by making variable names more distinguishable. Example: num_students, average_temperature
- Avoid using reserved keywords: Python has a set of reserved keywords that have predefined meanings in the language. These keywords cannot be used as variable names. Example: if, for, while
- Be consistent in your naming style: Maintain a consistent naming style throughout your codebase to enhance code readability and reduce confusion. Choose either snake_case or CamelCase and stick to it. Example: total_sales (snake_case), customerName (CamelCase)
- Use singular nouns for single values and plural nouns for collections: This convention helps to differentiate between singular and plural entities in your code. Example: car, cars
- Avoid using single letters or ambiguous names: While Python allows single letter variable names, it is recommended to use meaningful names to make the code more understandable. Example: Instead of x, use count or index.
Python Numeric data types: integers, floats, and complex numbers
Python provides different data types to represent and work with numbers. Understanding these numeric data types is fundamental for performing mathematical operations and manipulating numerical data in Python. there are three primary numeric data types in Python, integers, floats and complex numbers.
Python Integers:
Python Integers are whole numbers without any fractional or decimal part. In Python, integers are represented using the int data type. They can be positive or negative numbers, or even zero. For example in this code x and y are integer variables.
1 2 |
x = 5 y = -10 |
Python Floats:
Floats are also known as floating point numbers, they are numbers that contain a fractional part. They are represented using the float data type in Python. Floats can be positive or negative and can include decimal points. For example:
1 |
a = 3.14 b = -2.5 |
Python Complex Numbers:
Complex numbers are consist of a real part and an imaginary part. They are represented using the complex data type in Python. The imaginary part is denoted by the letter j or J. For example:
1 2 |
c = 2 + 3j d = -1j |
Python Text data type: strings and their manipulation
Text data plays an important role in programming, and understanding how to work with strings is essential for effectively manipulating and processing textual information in Python. In this section, we want to talk about different string manipulation techniques using Python.
Strings in Python:
In Python a string is a sequence of characters enclosed within single quotes (”) or double quotes (“”). Strings can contain letters, digits, symbols and even spaces. For example in this code name and message are string variables that store the name and a greeting.
1 2 |
name = 'geekscoders' message = "Hello World" |
Python String Manipulation Techniques:
Python provides different built-in functions and operators to manipulate strings. Let’s explore some common string manipulation techniques:
Concatenation:
You can concatenate or join two or more strings using the + operator like this.
1 2 3 4 |
first_name = 'Code' last_name = 'loog' full_name = first_name + ' ' + last_name print(full_name) |
This will be the result
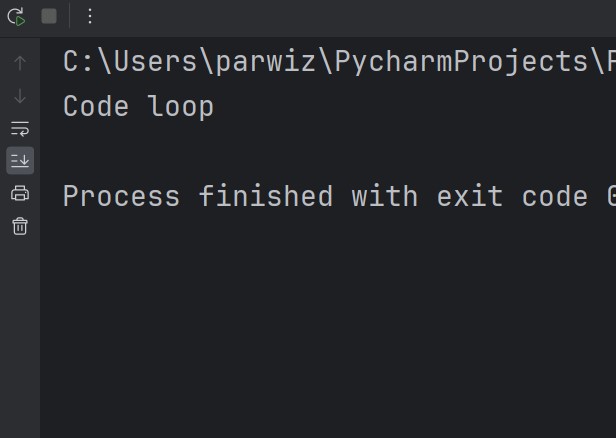
String Length:
The len() function returns the length of a string, which represents the number of characters it contains. For example:
1 2 |
message = 'Welcome to codeloop.org' length = len(message) |
Accessing Individual Characters:
You can access individual characters inside a string by using square brackets [] along with the index. Python uses zero based indexing, where the first character is at index 0. For example:
1 2 |
message = 'Hello' first_character = message[0] |
String Slicing:
Slicing allows you to extract a portion of a string. You can specify a range of indices inside square brackets [] to extract the desired substring like this:
1 2 |
message = 'Welcome to codeloop.org' substring = message[7:12] |
Python Boolean Data Type:
In Python Boolean data type represents two possible values, True and False. Booleans are often used to evaluate conditions or store the results of logical comparisons. For example in this code, is_raining and is_sunny are Boolean variables that represent whether it is raining or sunny.
1 2 |
is_raining = True is_sunny = False |
Python Logical Operators:
Python provides three primary logical operators, and, or and not. These operators allow us to combine and manipulate Boolean values to create more complex logical expressions.
- and Operator: The and operator returns True if both operands are True , and False otherwise. It represents the logical conjunction. This is an example:
1 2 3 |
x = True y = False result = x and y |
-
or Operator:
The or operator returns True if at least one of the operands is True, and False if both operands are False. It represents the logical disjunction.
1 2 |
a = True b = False |
-
not Operator:
not operator is a unary operator that returns the opposite of a Boolean value. If the operand is True, it returns False, and if the operand is False, it returns True. It represents the logical negation.
1 2 |
is_open = True is_closed = not is_open |
Combining Logical Operators:
You can combine multiple logical operators to create more complex logical expressions. Parentheses can be used to define the order of evaluation.
1 2 3 4 |
x = True y = False z = True result = (x and y) or (not z) |
Python Control Structures
In this section of Learn Python Step by Step we want to learn about Control Structures in Python, so Control structures in Python are programming constructs that enable the execution of specific code blocks based on certain conditions or criteria. They allows you to control the flow of execution in a program and make decisions based on the values of variables or the outcome of logical expressions. Python provides different control structures like if statements, loops and function definitions, that helps in creating structured and dynamic programs.
Python if, else, and elif statements
In Python, if, else, and elif are conditional statements that allows you to control the flow of execution based on certain conditions. These statements helps you make decisions and execute specific blocks of code accordingly.
Python if Statement:
The if statement is the simplest form of a conditional statement in Python. It allows you to execute a block of code only if a given condition is true. The basic syntax of an if statement is as follows:
1 2 |
if condition: # code to execute if the condition is true |
The condition can be any expression that evaluates to either True or False. If the condition is true, the code block under the if statement is executed; otherwise it is skipped.
Python else Statement:
The else statement is used in conjunction with the if statement to provide an alternative code block to execute when the if condition is false. This is the syntax for that:
1 2 3 4 |
if condition: # code to execute if the condition is true else: # code to execute if the condition is false |
When the condition is true, the code block under the if statement is executed. If the condition is false, the code block under the else statement is executed instead.
Python elif Statement:
The elif statement is short for else if, it allows you to test multiple conditions in sequence. It is used when you have more than two possible outcomes. This is the syntax for that:
1 2 3 4 5 6 |
if condition1: # code to execute if condition1 is true elif condition2: # code to execute if condition1 is false and condition2 is true else: # code to execute if both condition1 and condition2 are false |
The elif statement is evaluated only if the preceding if statement or elif statement(s) evaluated to false. It provides an additional condition to test, allowing for more complex decision-making scenarios.
Python Nested Conditional Statements:
You can also nest conditional statements inside one another to create even more complex decision structures. This allows for evaluating multiple conditions and executing code blocks accordingly. This is an example:
1 2 3 4 5 6 7 |
if condition1: if condition2: # code to execute if both condition1 and condition2 are true else: # code to execute if condition1 is true but condition2 is false else: # code to execute if condition1 is false |
This is a practical example of Python if, else, and elif statements.
1 2 3 4 5 6 7 8 9 10 |
# Checking if a number is positive or negative number = float(input("Enter a number: ")) if number > 0: print("The number is positive.") elif number < 0: print("The number is negative.") else: print("The number is zero.") |
In the above example, the user is prompted to enter a number. After that the program uses an if statement to check the value of the number.
- If the number is greater than 0, the condition number > 0 is true, and the code block under the if statement is executed. It prints The number is positive.
- If the number is less than 0, the condition number < 0 is true, and the code block under the elif statement is executed. It prints The number is negative.
- If the number is neither greater than 0 nor less than 0, the conditions number > 0 and number < 0 are false, and the code block under the else statement is executed. It prints The number is zero.
So this will be the result if you run the code

Python Looping structures, for and while loops
Looping structures in Python, namely for loops and while loops, allows you to repeat a block of code multiple times. They are powerful constructs for iterating over sequences, performing repetitive tasks and controlling the flow of execution in a program.
Python For Loop:
A for loop is used when you want to iterate over a sequence of elements, such as a list, tuple, string or range. It allows you to execute a block of code for each item in the sequence. This is the basic syntax of a for loop:
1 2 |
for item in sequence: # code to execute for each item in the sequence |
In each iteration, the variable item takes on the value of the current element in the sequence. The code block under the for loop is executed for each item in the sequence until the loop reaches the end of the sequence.
This is a practical example on printing numbers from 1 to 4 using a for loop
1 2 |
for num in range(1, 5): print(num) |
This will be the result

Python While Loop:
A while loop is used when you want to repeat a block of code as long as a given condition is true. It continues iterating as long as the condition remains true. This is the basic syntax of a while loop:
1 2 |
while condition: # code to execute while the condition is true |
In the above syntax the condition is checked before each iteration. If it evaluates to true, the code block under the while loop is executed. If it becomes false, the loop is exited, and program execution continues with the next statement after the while loop.
This is a practical example on printing numbers from 1 to 5 using a while loop:
1 2 3 4 |
num = 1 while num <= 5: print(num) num += 1 |
In the above example, the variable num is incremented by 1 in each iteration until it reaches 6, causing the condition num <= 5 to become false and ending the while loop.
Both for loops and while loops are valuable tools for controlling program flow and performing repetitive tasks. Depending on the situation, you can choose the appropriate loop structure to achieve the desired functionality in your Python programs.
Python Break and Continue Statements
In Python break and continue statements are powerful tools that allows you to control the flow of loops. These statements provides flexibility and nice control when working with loops, and it enables you to terminate a loop prematurely or skip iterations based on certain conditions, so now let’s talk about these two concepts practically.
Python Break Statement:
break statement is used to abruptly terminate a loop, regardless of whether the loop condition is still true. When we encountered to the break statement, it immediately exits the loop, and program execution continues with the next statement after the loop. The break statement is often used when a specific condition is met, and there is no need to continue the loop. This is an example to illustrate its usage:
1 2 3 4 |
for num in range(1, 5): if num == 4: break print(num) |
In the above example for loop iterates over the numbers from 1 to 4. but when the value of num becomes 4, the condition num == 4 is satisfied, and the break statement is executed. As a result, the loop is terminated prematurely, and the number 4 is not printed.
This will be the result

Python Continue Statement:
The continue statement is used to skip the rest of the current iteration and move on to the next iteration of a loop. When we encountered in this scenario, the continue statement jumps to the next iteration without executing the remaining code within the loop block. It is typically used when you want to skip certain iterations based on specific conditions. This is an example for that.
1 2 3 4 |
for num in range(1, 5): if num == 2: continue print(num) |
In the above example, the for loop iterates over the numbers from 1 to 4. When num equals 2, the condition num == 2 is satisfied, and the continue statement is executed. As a result, the code block below the continue statement is skipped for that iteration, and the loop proceeds to the next iteration.
This will be the result

Subscribe and Get Free Video Courses & Articles in your Email