In this Numpy article we want to learn about Array Operations in Numpy Python, Numpy is a powerful library in Python for numerical computations, and it provides support for multi-dimensional arrays and different mathematical operations. it has the ability to efficiently manipulate arrays, also it is an essential tool for scientific computing, data analysis, and machine learning tasks. in this article we want to talk about some common numpy array operations.
First of all you need to install Numpy, for that you can use pip.
1 |
pip install numpy |
How to Create Numpy Arrays
Before we dive into array operations, let’s start by creating numpy arrays. Numpy provides different methods to create arrays, including:
- Using the np.array() function: This function takes a Python list or tuple and converts it into a numpy array.
- Using functions like np.zeros(), np.ones() and np.arange(): These functions create arrays of zeros, ones, or a range of values.
This is an example
1 2 3 4 5 6 7 8 9 10 11 12 13 |
import numpy as np # Creating numpy array from a list arr = np.array([1, 2, 3, 4, 5]) print(arr) # Output: [1 2 3 4 5] # Creating 2D numpy array of zeros zeros_arr = np.zeros((3, 3)) print(zeros_arr) # Creating a 1D numpy array with a range of values range_arr = np.arange(1, 10, 2) print(range_arr) |
Run the complete code and this will be the result
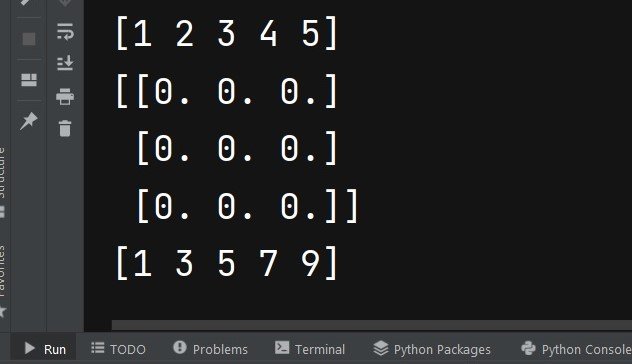
Numpy Array Manipulation
Numpy provides several functions to manipulate arrays, such as reshaping, slicing and concatenating.
- Reshaping Arrays: The np.reshape() function allows us to change the shape of an array, while maintaining the same elements.
This is an example
1 2 3 4 5 6 7 8 9 10 |
import numpy as np arr = np.array([1, 2, 3, 4, 5, 6]) reshaped_arr = np.reshape(arr, (2, 3)) print(reshaped_arr) #Slicing Arrays: Numpy arrays arr = np.array([1, 2, 3, 4, 5]) slice_arr = arr[1:4] print(slice_arr) |
This will be the result

- Concatenating Arrays: Numpy provides functions like np.concatenate() and np.vstack() to concatenate arrays vertically or horizontally.
1 2 3 4 5 6 |
import numpy as np arr1 = np.array([1, 2, 3]) arr2 = np.array([4, 5, 6]) concatenated_arr = np.concatenate((arr1, arr2)) print(concatenated_arr) |
This will be the result

Numpy Mathematical Operations
Numpy simplifies mathematical computations by allowing element wise operations on arrays, as well as matrix operations.
- Element-wise Operations: Numpy supports arithmetic operations like addition, subtraction, multiplication and division on arrays.
Example:
1 2 3 |
arr = np.array([1, 2, 3, 4, 5]) result = arr + 2 print(result) # Output: [3 4 5 6 7] |
- Matrix Operations: Numpy provides functions for matrix operations like dot product (np.dot()), matrix multiplication (np.matmul()) and transpose (np.transpose()).
Example:
1 2 3 4 5 6 |
import numpy as np matrix1 = np.array([[1, 2], [3, 4]]) matrix2 = np.array([[5, 6], [7, 8]]) dot_product = np.dot(matrix1, matrix2) print(dot_product) |
Learn More on Python Numpy
- Python Numpy for Machine Learning
- How to Install Numpy
- Working with Linear Algebra in Numpy
- Numpy vs Pandas
- Advance Python Numpy Techniques
- Numpy Array Indexing and Slicing
- Numpy Mathematical Functions
- Numpy Random Number Generation in Python
- Working with Multi-Dimensional Arrays in Numpy
Subscribe and Get Free Video Courses & Articles in your Email