In this article we want to talk about Tkinter vs PyQt, also we will talk that Which GUI Framework is Good? Both Tkinter and PyQt are popular GUI frameworks for Python and they both have their advantages and disadvantages. we can say that the choice between the two depends on your specific needs and preferences, first of all let’s talk about these two GUI Frameworks. and after that we will talk that Which GUI Framework is good in Python.
What is TKinter?
tkinter is standard GUI library for Python. TKinter is already built-in in Python, it means that you don’t need to install that, TKinter is one of the best library for creating desktop applications that has graphical user interface, also tkinter is easy to use, it means that you can easily build simple GUI applications with TKinter.
Key Components of tkinter
- Widgets: Basic building blocks of a tkinter application. Examples are buttons, labels, text boxes, frames and menus.
- Geometry Managers: Methods to control the layout of widgets. most commonly used geometry managers are pack, grid and place.
- Events and Bindings: Mechanisms to handle user interactions such as button clicks, key presses, and other events.
Basic Example of TKinter
This is a basic example of TKinter GUI application, in this GUI application we have a window with label and button.
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17 18 19 20 21 22 |
import tkinter as tk def on_button_click(): label.config(text="This is codeloop.org") # Create main window root = tk.Tk() root.title("Codeloop.org - Simple Tkinter App") # Set window icon root.iconphoto(True, tk.PhotoImage(file='codeloop.png')) # Create label widget label = tk.Label(root, text="Hello, Tkinter") label.pack(pady=10) # Create button widget button = tk.Button(root, text="Click Me", command=on_button_click) button.pack(pady=10) # Run the application root.mainloop() |
Now let’s describe this code:
- Importing tkinter: The library is imported as tk.
- Creating the Main Window: Tk class is instantiated for creating the main application window.
- Creating Widgets: Label widget and a Button widget are created. and command parameter of the Button widget is set to the on_button_click function, which will be called when the button is clicked.
- Adding Widgets to the Window: pack geometry manager is used to add the widgets to the window and manage their layout.
- Running the Application: mainloop method is called on the root window to start the event loop, which waits for user interactions.
Run the code and this will be the result
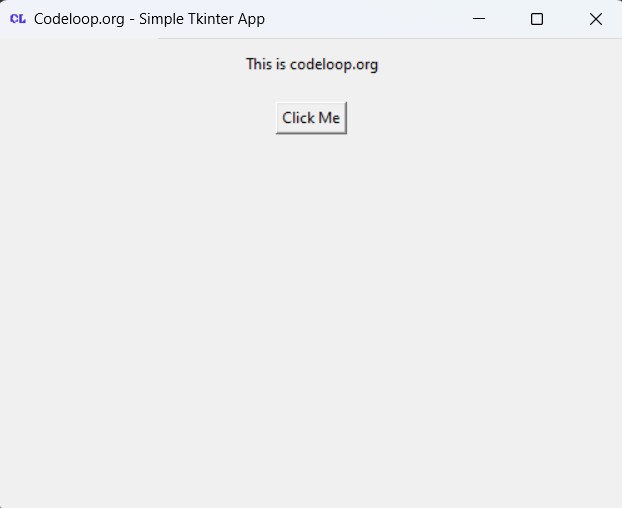
What is PyQt?
PyQt is a set of Python bindings for Qt application framework, PyQt was developed by Riverbank Computing. Using PyQt you can build desktop applications with graphical user interfaces (GUIs) in Python. Qt itself is a cross platform GUI framework for developing GUI Applications in C++ programming language.
Key Features of PyQt
- Cross-Platform: PyQt applications can run on different operating systems, like Windows, macOS, and Linux, and you don’t need to bring changes in codebase
- Comprehensive: PyQt provides different widgets and tools for creating complex and highly functional user interfaces.
- Integrated: PyQt supports different non-GUI features such as networking, threading, and database integration.
- Customizable: You can use PyQt for creation of custom widgets and functionalities.
- Licensing: PyQt is available under the GPL and a commercial license. And using this dual license you can build free and commercial gui applications.
Key Components of PyQt
- Widgets: It is building blocks for creating a user interface, such as buttons, labels, text boxes and many more.
- Layouts: It Manages the arrangement of widgets in the window, and it will ensure responsive and well-organized interface.
- Signals and Slots: It is a mechanism for event handling, connecting user actions (signals) to functions (slots) that define the application’s behavior.
- Qt Designer: It is drag-and-drop interface, you can use that for designing and building GUIs visually, after that it will be integrated into PyQt applications.
Basic Example of PyQt
Now let’s create a basic gui example in PyQt.
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17 18 19 20 21 22 23 24 25 26 27 28 29 30 31 32 33 34 35 |
import sys from PyQt5.QtWidgets import QApplication, QWidget, QLabel, QPushButton, QVBoxLayout from PyQt5.QtGui import QIcon def on_button_click(): label.setText("This is codeloop.org") # Create application object app = QApplication(sys.argv) # Create main window window = QWidget() window.setWindowTitle("Codeloop.org - Simple PyQt App") window.setWindowIcon(QIcon('codeloop.png')) # Create label widget label = QLabel("Hello PyQt") # Create button widget button = QPushButton("Click Me") # Connect button click to the function button.clicked.connect(on_button_click) # Create layout and add widgets layout = QVBoxLayout() layout.addWidget(label) layout.addWidget(button) window.setLayout(layout) # Show the window window.show() # Run the application event loop sys.exit(app.exec_()) |
Now let’s describe the code
- Importing PyQt Modules: These are necessary PyQt modules that we have imported.
- Application Object: QApplication is instantiated to manage application level resources and settings.
- Main Window: It is QWidget instance, and it is created for the main application window.
- Widgets: It is QLabel and QPushButton are that we have created.
- Signal and Slot: The button’s clicked signal is connected to on_button_click function using the clicked.connect method.
- Layout: QVBoxLayout is used to arrange the label and button vertically.
- Running the Application: app.exec_() starts the event loop, and sys.exit() ensures a clean exit from the application.
Run the code and this will be the result
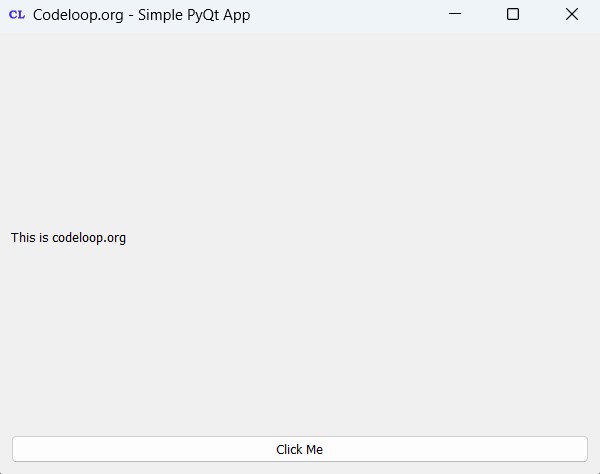
Tkinter vs PyQt: Which GUI Framework is Good?
So now let’s talk about the best GUI between these two , Now these are some factors to consider when choosing Python GUI Framework:
- Easy of use: Tkinter is easier to learn than PyQt, especially for beginners who are new to GUI programming. but PyQt has more advanced features and capabilities, and we don’t have much powerful widgets in TKinter, so if you have complex project then you need to go with PyQt, for simple project it good to go with TKinter.
- Documentation and support: Both frameworks have good documentation and active communities that provide support and resources. but PyQt has more extensive documentation and support resources.
- Performance: PyQt has better performance and it is more scalable than Tkinter. It also has more modern and flexible architecture.
- Licensing: Tkinter is included with Python, so it is free and open source. On the other hand PyQt is licensed unde GPL or a commercial license, which means you may need to purchase license if you want to use it for commercial purposes.
So we can say if you are new to GUI programming or need to create simple application, Tkinter is good choice. If you need more advanced features or more powerful framework for large scale project, PyQt is the better option.
Subscribe and Get Free Video Courses & Articles in your Email