In this Python TKinter we want to learn about Python TKinter Creating Menu & MenuItems, so first of all let’s talk about Menu & MenuItems in TKinter.
What is Menu & MenuItems in Tkinter?
In Tkinter Menu is a widget that provides a set of options or commands to the user, and i will displayed as a horizontal or vertical, also it will be clickable. Each item in a menu is represented by a MenuItem. This is a brief overview of both:
TKinter Menu:
- Widget: Menu is a built-in class in Tkinter, and it is a menu widget.
- Purpose: Menus are used to organize and present a list of options or commands to the user, and it is often a part of a graphical user interface (GUI).
- Types: There are different types of menus in Tkinter, for example we have top-level menus, cascading menus (submenus) and context menus (pop-up menus).
- Creation: You can create a Menu widget using Menu class constructor in TKinter.
- Attributes: Menus can have different attributes such as tear-off capability (and it allows the menu to be detached from the window), accelerators (keyboard shortcuts) and more.
- Items: Menus can contain multiple menu items (MenuItem), and it can be added using methods like add_command(), add_separator() and add_cascade().
TKinter MenuItem:
- Widget: MenuItem is not a separate class in Tkinter but it is a generic term used to refer to items inside menu.
- Purpose: MenuItem is an individual item or option inside a menu.
- Types: Menu items can include commands like triggering a function when it is clicked.
- Creation: Menu items are created and added to a menu using methods like add_command(), add_separator() and add_cascade().
- Event Handling: You can bind events to menu items to trigger specific actions when the user interacts with them, such as clicking or hovering.
This is the complete source code for this article
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17 18 19 20 21 22 23 24 25 26 27 28 29 30 31 32 33 34 35 36 37 38 39 40 41 42 43 44 45 46 47 48 |
import tkinter as tk from tkinter import ttk from tkinter import Menu class App(tk.Tk): def __init__(self): super(App, self).__init__() # Set title of the window self.title("Codeloop.org - Menus & MenuItems") # Set window icon self.iconphoto(True, tk.PhotoImage(file='codeloop.png')) # Call method to create the menu self.createMenu() def createMenu(self): # Create menu bar menuBar = Menu(self) self.config(menu=menuBar) # Create File menu file_menu = Menu(menuBar, tearoff=0) # Add File menu to the menu bar menuBar.add_cascade(label="File", menu=file_menu) # Add commands to File menu file_menu.add_command(label='New') file_menu.add_command(label='Exit') file_menu.add_separator() file_menu.add_command(label="Open") # Create Help menu help_menu = Menu(menuBar, tearoff=0) # Add Help menu to menu bar menuBar.add_cascade(label='Help', menu=help_menu) # Add command to the Help menu help_menu.add_command(label='About') # Create an instance of the App class app = App() app.mainloop() |
These are the imports that we need for this article, basically it is tkinter library, also we are going to import ttk from tkinter , with Menu.
1 2 3 |
import tkinter as tk from tkinter import ttk from tkinter import Menu |
So in this part we have used Object Oriented Programming (OOP), we have created a class that extends from tk.TKand we have added our window title, window size and window icon in the constructor of the class, make sure that you have added an icon to your working directory . also we have called our def createMenu() method in this class.
1 2 3 4 5 6 7 8 9 10 11 12 |
class App(tk.Tk): def __init__(self): super(App, self).__init__() # Set title of the window self.title("Codeloop.org - Menus & MenuItems") # Set window icon self.iconphoto(True, tk.PhotoImage(file='codeloop.png')) # Call method to create the menu self.createMenu() |
In this method first we have created the object of Menu. and also have we created a file menu and we add some menuitem like new, exit and open to our File menu.
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 |
def createMenu(self): menuBar = Menu(self) self.config(menu = menuBar) file_menu = Menu(menuBar, tearoff = 0) menuBar.add_cascade(label="File", menu=file_menu) file_menu.add_command(label='New') file_menu.add_command(label='Exit') file_menu.add_separator() file_menu.add_command(label="Open") # Add Another Menu to Menbar help_menu = Menu(menuBar, tearoff = 0) menuBar.add_cascade(label = 'Help', menu = help_menu) help_menu.add_command(label = 'About') |
Run the complete code and this will be the result.
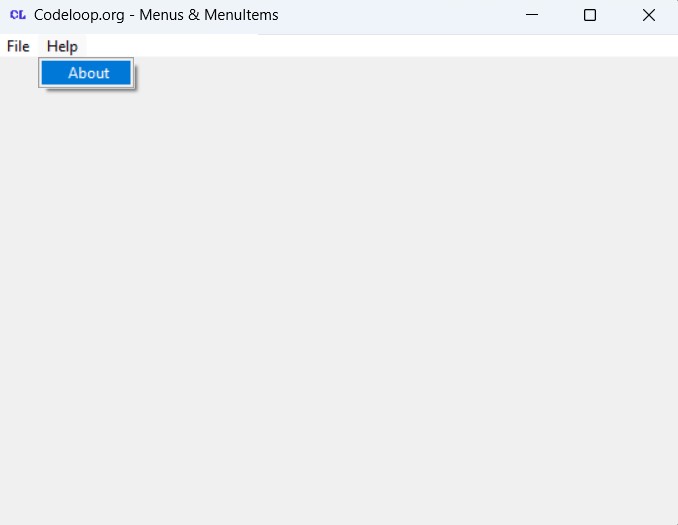
FAQs:
What is TKinter?
Tkinter is standard GUI (Graphical User Interface) toolkit for Python. It is included with most Python installations, it is used for creating desktop applications with graphical interfaces. Tkinter provides different Python modules, and it allows you to create windows, buttons, menus and other GUI elements. TKinter is based on the Tk GUI toolkit.
How to Install TKinter?
Tkinter is included as standard library in most Python installations, so you don’t need to install it separately.
How to create a menu in tkinter python?
For creating a menu in tkinter Python, you can use Menu widget provided by tkinter. This is a basic example:
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 |
import tkinter as tk root = tk.Tk() menu = tk.Menu(root) root.config(menu=menu) file_menu = tk.Menu(menu) menu.add_cascade(label="File", menu=file_menu) file_menu.add_command(label="New") file_menu.add_command(label="Open") file_menu.add_separator() file_menu.add_command(label="Exit", command=root.quit) root.mainloop() |
How to create a side menu in Tkinter?
Tkinter doesn’t have specific widget for creating side menus, but you can simulate a side menu using frames and buttons. This is a basic example:
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17 18 |
import tkinter as tk root = tk.Tk() side_menu = tk.Frame(root, bg="gray", width=100) side_menu.pack(side="left", fill="y") button1 = tk.Button(side_menu, text="Option 1") button1.pack() button2 = tk.Button(side_menu, text="Option 2") button2.pack() button3 = tk.Button(side_menu, text="Option 3") button3.pack() content = tk.Frame(root, bg="white") content.pack(side="right", fill="both", expand=True) root.mainloop() |
Subscribe and Get Free Video Courses & Articles in your Email