In this Python TKinter article i want to show you Creating Label Frame. first of all let’s talk about label frame in TKinter.
What is Label Frame in Python TKinter?
In Python Tkinter, a LabelFrame is a widget, and it is used to group and organize other widgets inside a container. LabelFrame provides a visual boundary around its child widgets and typically includes a title or label to describe the purpose of the grouped widgets.
A LabelFrame serves a similar purpose to a frame but with the added functionality of displaying a label or title. It helps in organizing the layout of complex GUIs by visually grouping related widgets together.
Why Use Label Frame in TKinter?
LabelFrames are particularly useful when you want to organize and categorize different sections or groups of widgets inside a window. They enhance the readability and usability of the GUI.
This is the complete source code for this article
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17 18 19 20 21 22 23 24 25 26 27 28 29 30 31 32 33 34 |
import tkinter as tk from tkinter import ttk class LabelFrameExample(tk.Tk): def __init__(self): super(LabelFrameExample, self).__init__() # Initialize the main window self.title("Codeloop.org - LabelFrame Example") # Set window icon self.iconphoto(True, tk.PhotoImage(file='codeloop.png')) # Create widgets self.create_widgets() def create_widgets(self): # Create a LabelFrame with a title self.label_frame = ttk.LabelFrame(self.master, text="Grouped Widgets") self.label_frame.pack(padx=10, pady=10) # Add widgets inside the LabelFrame self.label = ttk.Label(self.label_frame, text="This is a Label") self.label.grid(row=0, column=0, padx=5, pady=5) self.button = ttk.Button(self.label_frame, text="Click Me") self.button.grid(row=1, column=0, padx=5, pady=5) def main(): app = LabelFrameExample() app.mainloop() if __name__ == "__main__": main() |
In the above code:
- We have defined a class LabelFrameExample to encapsulate the functionality of creating the Tkinter GUI.
- In the __init__ method of the class, we initialize the main window, set its title, and set the window icon.
- The create_widgets method creates the LabelFrame and its child widgets (Label and Button).
- We define a main function to create an instance of LabelFrameExample and start the Tkinter event loop.
- And lastly we check if the script is being run directly (not imported as a module) and call the main function accordingly.
Run the complete code and this will be the result
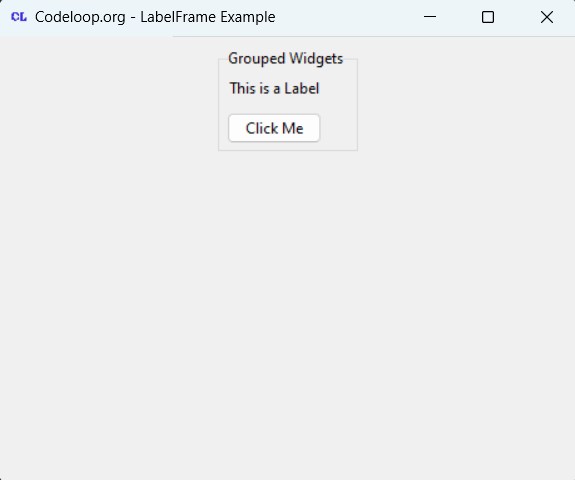
FAQs:
How to create a label frame in Tkinter?
A label frame in Tkinter can be created using LabelFrame widget from the ttk module. First, you need to create an instance of the LabelFrame class and specify its parent window. You can also provide text for the label frame using text parameter. After that you can add other widgets, such as labels, buttons, or entry fields to the label frame.
How to design a label in Tkinter?
In Tkinter, you can design a label using the Label widget from the ttk module. You can create an instance of the Label class and specify its parent window along with other parameters such as text, font, foreground (text color), background (label color) and alignment.
1 2 3 4 5 6 7 8 9 10 11 12 13 |
import tkinter as tk from tkinter import ttk # Create main window root = tk.Tk() root.title("Label Design Example") # Create Label widget label = ttk.Label(root, text="TKinter Tutorial", font=("Arial", 12), foreground="blue", background="lightgray", anchor="center") label.pack(padx=20, pady=20) # Run Tkinter event loop root.mainloop() |
What are label frames?
Label frames in Tkinter are container widgets used to group and organize other widgets, such as labels, buttons, or entry fields inside a frame. They provide a visual boundary around the grouped widgets and typically include a title or label to describe the purpose of the grouped widgets. Label frames enhance the readability and organization of GUIs.
Subscribe and Get Free Video Courses & Articles in your Email