Python Socket | How To Convert IPv4 Address – When you would like to deal with low-level network functions, sometimes, the usual string
notation of IP addresses are not very useful. They need to be converted to the packed 32-bit binary formats.
The Python socket library has utilities to deal with the various IP address formats. Here, we will use two of them: inet_aton() and inet_ntoa().
Also you can check Python Socket Programming Articles
1: Python Socket Programming How To Create Socket
2: Python Socket How To Get Local Machine IP Address
3: Python Socket How To Get Website IP Address
4: Python Socket How To Connect TCP Client To Server
So now this is the complete code
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17 18 19 |
import socket from binascii import hexlify def ipConversion(): for ip_addr in ['127.0.0.1', '192.168.0.1']: packed_ip_addr = socket.inet_aton(ip_addr) unpacked_ip_addr = socket.inet_ntoa(packed_ip_addr) print("IP Address : {} => Packed: {}, Unpacked: {} " .format(ip_addr, hexlify(packed_ip_addr), unpacked_ip_addr)) ipConversion() |
So at the top we have created ipConversion() method, where inet_aton() and inet_ntoa() will be used for the IP address conversion. We will use two sample IP
addresses, 127.0.0.1 and 192.168.0.1.
Run the complete code this will be the result
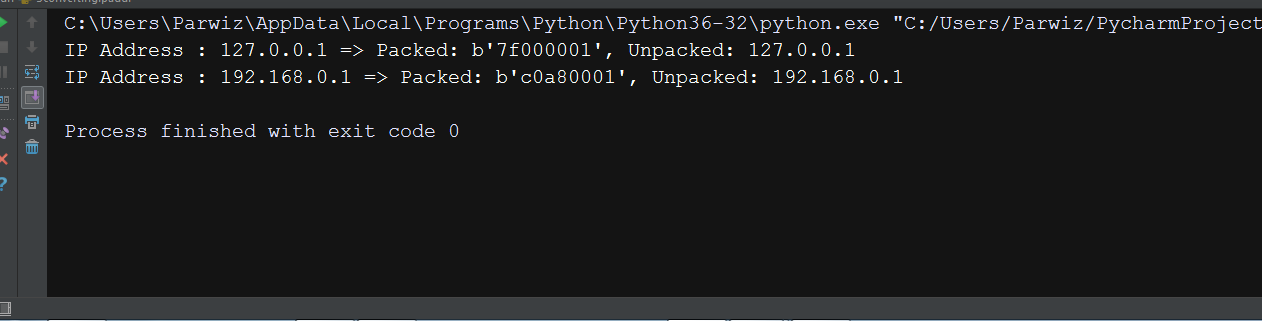
How it Works
In this article, the two IP addresses have been converted from a string to a 32-bit packed
format using a for-in statement. Additionally, the Python hexlify function is called from
the binascii module. This helps to represent the binary data in a hexadecimal format.
Also you can watch the complete video for this article
Subscribe and Get Free Video Courses & Articles in your Email
Great tutorial, nice and clear. Thanks
welcome