In this Python Pyglet article i want to show you Adding GIF Image to the Window. so in general we are going to talk about Animations in Pyglet. While image sequences and atlases provide storage for related images, they alone are not enough to describe a complete animation.
The Animation class manages a list of AnimationFrame objects, each of which references an image and a duration (in seconds). The storage of the images is up to the application developer: they can each be discrete, or packed into a texture atlas, or any other technique.
An animation can be loaded directly from a GIF 89a image file with load_animation() (supported on Linux, Mac OS X and Windows) or constructed manually from a list of images or an image sequence using the class methods (in which case the timing information will also need to be provided). The add_to_texture_bin() method provides a convenient way to pack the image frames into a texture bin for efficient access.
Individual frames can be accessed by the application for use with any kind of rendering, or the entire animation can be used directly with a Sprite (see next section).
The following example loads a GIF animation and packs the images in that animation into a texture bin. A sprite is used to display the animation in the window.
What is Python Pyglet?
Pyglet is a cross platform multimedia library for Python. It provides an easy interface for creating games, interactive applications, multimedia software and many more. Pyglet is built on top of OpenGL and provides bindings for audio, video and input devices, and this is good for different types of multimedia applications.
Key features of Pyglet:
-
- Graphics: Pyglet provides powerful graphics module for rendering 2D and 3D graphics using OpenGL. It supports hardware-accelerated rendering and provides utilities for handling textures, sprites, shapes and transformations.
- Audio: Pyglet includes audio module for playing and managing audio files. It supports different audio formats and provides features for controlling playback, adjusting volume, and handling sound effects.
- Video: Pyglet has built-in support for playing video files using FFmpeg. It allows you to load, play and manipulate video files inside your Python applications.
- Input Handling: Pyglet provides input handling capabilities for handling user interactions such as keyboard, mouse and joystick events. It allows you to easily detect and respond to user input in your applications.
- Windowing: Pyglet includes a windowing module for creating and managing windows and OpenGL contexts. It allows you to create customizable windows with support for resizing, fullscreen mode, and multiple monitors.
How to Install Pyglet?
You can install Python Pyglet using pip like this
1 |
pip install pyglet |
This is the complete code for Python Pyglet Adding GIF Image To Window
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17 18 19 20 21 22 23 24 25 26 27 28 29 |
import pyglet # Load GIF animation from file animation = pyglet.image.load_animation('simple.gif') # Create sprite from the animation animSprite = pyglet.sprite.Sprite(animation) # Get width and height of the sprite w = animSprite.width h = animSprite.height # Create Pyglet window with the same dimensions as the sprite window = pyglet.window.Window(width=w, height=h) # Set clear color for the window r, g, b, alpha = 0.5, 0.5, 0.8, 0.5 pyglet.gl.glClearColor(r, g, b, alpha) # Define the on_draw event handler @window.event def on_draw(): # Clear the window window.clear() # Draw the sprite animSprite.draw() # Start the Pyglet application event loop pyglet.app.run() |
Explanation of each part:
- Import Pyglet: Import Pyglet library.
- Load Animation: Load GIF animation from the file named ‘simple.gif’ using pyglet.image.load_animation().
- Create Sprite: Create a sprite (animSprite) from the loaded animation.
- Get Sprite Dimensions: Get the width (w) and height (h) of the sprite.
- Create Window: Create Pyglet window with dimensions matching the sprite’s width and height.
- Set Clear Color: Set clear color for the window using pyglet.gl.glClearColor().
- Define on_draw Event Handler: Define the on_draw() function, which is called whenever the window needs to be redrawn. In this function, clear the window and draw the sprite.
- Start Event Loop: Start the Pyglet application event loop using pyglet.app.run(), which continuously listens for events and updates the window accordingly
This is the result but in this image you can not see the animation when you run the code in your IDE you will see the animation.
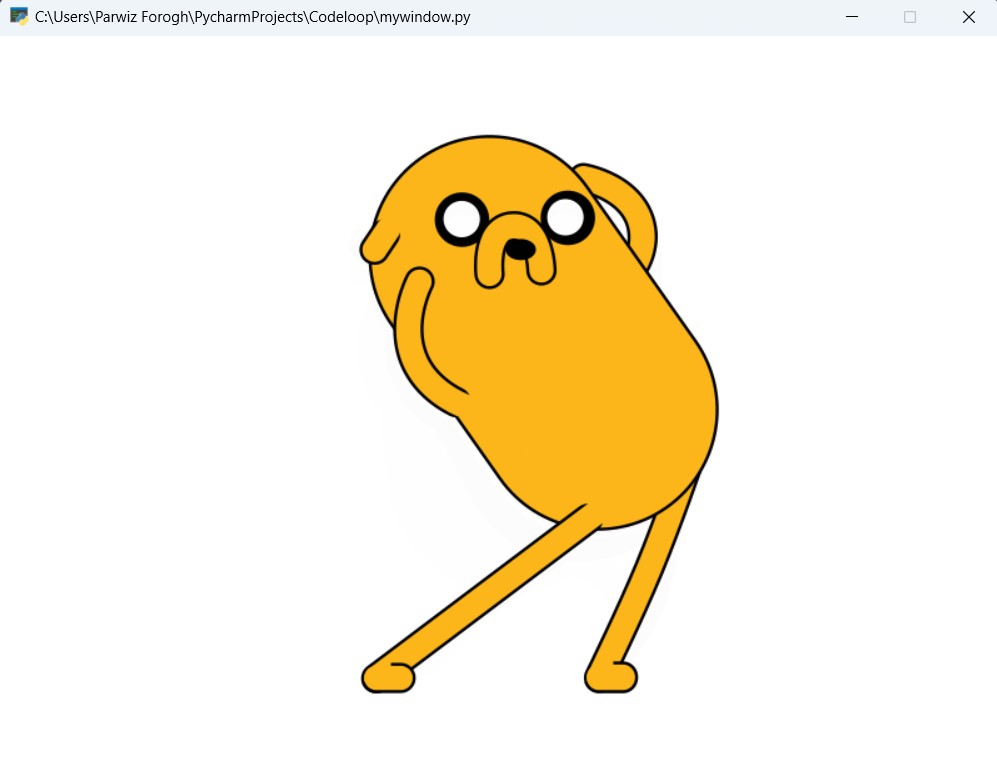
FAQs:
How do I import a GIF into Python?
For importing a GIF into Python, you can use different libraries, and it depends on your needs. If you’re working with GUI frameworks like Tkinter or Pygame, you can directly use the GIF file as an image resource.
How to make a GIF of plots in Python?
For creating a GIF of plots in Python, you can use libraries like Matplotlib or Seaborn to generate the plots, and after that use a library like imageio to save the plots as individual frames and create a GIF. This is a basic example:
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17 18 19 20 |
import matplotlib.pyplot as plt import numpy as np import imageio frames = [] for i in range(10): x = np.linspace(0, 2 * np.pi, 100) y = np.sin(x + i) plt.plot(x, y) plt.title(f'Frame {i}') plt.xlabel('X') plt.ylabel('Y') plt.grid(True) plt.savefig(f'frame_{i}.png') plt.close() frames.append(imageio.imread(f'frame_{i}.png')) imageio.mimsave('animation.gif', frames, fps=10) |
Subscribe and Get Free Video Courses & Articles in your Email