In this Python OpenCV article i want to show you how you can do Writing in To A Video, so writing to a video in Python with OpenCV involves capturing frames from a source, processing them as needed, and then encoding and writing these frames sequentially to a video file.
Also you can watch my previous post on How To Read Mp4 Videos In Python OpenCV .
This is the complete source code for this article
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17 18 19 20 21 22 23 24 25 26 27 28 29 30 31 32 33 34 35 36 37 38 39 40 41 42 43 44 45 46 47 48 49 50 51 52 53 54 55 56 |
import cv2 def main(): # Define the window name windowname = "Codeloop - Writing A Video" # Create a named window for display cv2.namedWindow(windowname) # Open the video file for reading cap = cv2.VideoCapture('vid2.mp4') # Define the output filename, codec, frame rate, and resolution for the output video filename = "out.avi" codec = cv2.VideoWriter_fourcc('X', 'V', 'I', 'D') framerate = 29 resolution = (640, 480) # Create a VideoWriter object for writing the output video VideoOutPut = cv2.VideoWriter(filename, codec, framerate, resolution) # Check if the video capture device is opened successfully if cap.isOpened(): ret, frame = cap.read() else: ret = False # Loop through the video frames while ret: # Read the next frame from the video ret, frame = cap.read() # Draw a green circle on the frame cv2.circle(frame, (200, 200), 80, (0, 255, 0), -1) # Write the frame to the output video VideoOutPut.write(frame) # Display the frame in the window cv2.imshow(windowname, frame) # Check for key press to exit the loop if cv2.waitKey(1) == 27: break # Destroy all OpenCV windows cv2.destroyAllWindows() # Release the VideoWriter object and video capture device VideoOutPut.release() cap.release() if __name__ == "__main__": main() |
In the above code first we have imported cv2
1 |
import cv2 |
In these line of codes we are going to give name for our OpenCV window.
1 2 |
windowname = "Codeloop - Writing A Video" cv2.namedWindow(windowname) |
This section of code is for creating and reading from input file.if the input is a camera pass 0instead of the video filename.
1 |
cap = cv2.VideoCapture('vid2.mp4') |
When we want to write a video in OpenCV we need to take some more steps. Firs we need to create a VideoWriter object. After that we should specify the output file name with its format (eg: output.avi). Then, we should specify the FourCC code and the number of frames per second (FPS). Lastly, the frame size should be passed.
FourCC is 4-character code of codec used to compress the frames. For example, VideoWriter::fourcc(‘P’,’I’,’M’,’1′) is a MPEG-1 codec, VideoWriter::fourcc(‘M’,’J’,’P’,’G’) is a motion-jpeg codec etc. List of codes can be obtained at Video Codecs by FOURCC page. FFMPEG backend with MP4 container natively uses other values as fourcc code.
1 2 3 4 |
filename = "out.avi" codec = cv2.VideoWriter_fourcc('X', 'V', 'I', 'D') framerate = 29 resolution = (640, 480) |
In here we have added our resolution and also frame rate.
1 |
VideoOutPut = cv2.VideoWriter(filename, codec, framerate, resolution) |
After that we are checking that if the capture is opened we are reading the frames.
1 2 3 4 5 6 7 8 9 |
if cap.isOpened(): ret, frame = cap.read() else: ret = False while ret: ret, frame = cap.read() |
Because we want to draw a circle in the recorder video so you can do like this
1 |
cv2.circle(frame, (200,200), 80, (0,255,0), -1) |
Run the code you will see the video
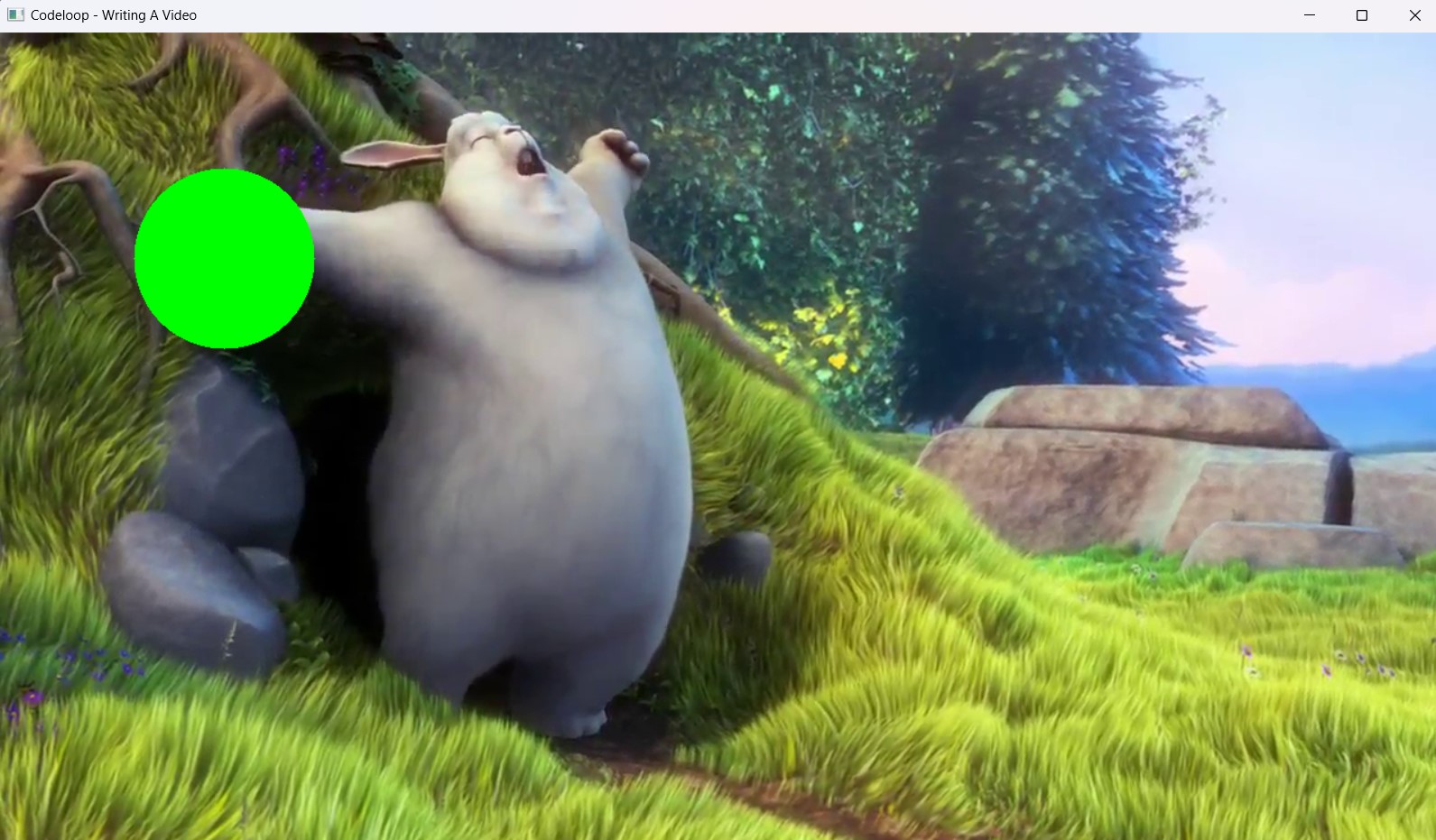
FAQs:
How do I write text on a video in OpenCV Python?
You can write text on a video in OpenCV Python using cv2.putText() function. This function takes the video frame, the text to be written, the position of the text, the font face, font scale, color, thickness and other optional parameters as input.
How to write a video using cv2?
For writing a video using cv2 in Python, you need to follow these steps:
- Open video file for reading using cv2.VideoCapture().
- Define the output video codec and create a cv2.VideoWriter object using cv2.VideoWriter().
- Loop through the video frames.
- Process each frame as needed.
- Write each processed frame to the output video using write() method of the cv2.VideoWriter object.
- Release resources after processing is complete.
What is a cv2 VideoWriter?
cv2.VideoWriter is a class in the OpenCV library, and it is used to write video files. It provides functionality to create video files and write frames sequentially to the file. You can specify parameters such as the output file name, codec, frame rate, frame size and more when creating a cv2.VideoWriter object.
Subscribe and Get Free Video Courses & Articles in your Email