In this Python Modern Opengl i want to show you GLFW Window creation. So when you are going to work with Modern Opengl, for Window creation you need to use another library that is called GLFW.
Because Opengl is a graphic library and we don’t have window, keyboard inputs, mouse inputs and some other low level functionality.
What is Opengl?
Opengl is an application programming interface also we call call it API which is merely software library for accessing features in graphic hardware and also opengl is designed as streamlined, hardware independent interface that can be implemented on many different types of graphic hardware systems. So in this article we are not going to use modern opengl programming but in the further articles we will discuss about that.
What is GLFW ?
GLFW is an Open Source, multi-platform library for OpenGL, OpenGL ES and Vulkan development on the desktop. It provides a simple API for creating windows, contexts and surfaces, receiving input and events.GLFW is written in C and supports Windows, macOS, the X Window System and the Wayland protocol.
Key Features of GLFW
- Window Management: GLFW allows you to create windows and OpenGL contexts with a few lines of code. It provides functions for configuring window properties such as size, title, and fullscreen mode.
- Input Handling: GLFW supports handling input events such as keyboard key presses, mouse movements and joystick/controller input. It provides functions for querying the state of input devices and registering callbacks to respond to input events.
- OpenGL Context Management: GLFW provides functions for creating and managing OpenGL contexts. It supports modern OpenGL features, including support for specifying OpenGL version, profile and compatibility mode.
- Multiplatform Support: GLFW is designed to be portable and works across different platforms, including Windows, macOS and different Unix-like systems (such as Linux and BSD). This allows you to write OpenGL applications that can run on multiple operating systems without modification.
PyOpengl & GLFW Installation
You can simply open your command prompt or terminal and write this command.
1 |
pip install pyopengl |
1 |
pip install glfw |
Python Modern Opengl GLFW Window
It is time to create our example, this is our complete code for this article
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17 18 19 20 21 22 23 24 25 26 27 28 29 30 31 |
import glfw def main(): # Initialize GLFW library if not glfw.init(): return # Create window with the specified dimensions and title window = glfw.create_window(720, 600, "Codeloop.org - Opengl GLFW Window", None, None) # Check if window creation was successful if not window: # Terminate GLFW if window creation failed glfw.terminate() return # Set the current OpenGL context to the created window glfw.make_context_current(window) # Main render loop while not glfw.window_should_close(window): # Poll for events like keyboard and mouse input glfw.poll_events() # Swap the front and back buffers to display the rendered image glfw.swap_buffers(window) glfw.terminate() # Terminate GLFW once the main loop exits # Entry point of the script if __name__ == "__main__": main() # Call the main function |
In this line of code we have initialized the library
1 2 |
if not glfw.init(): return |
Now create a windowed mode window and its OpenGL context
1 2 3 4 5 |
window = glfw.create_window(720, 600, "Opengl GLFW Window", None, None) if not window: glfw.terminate() return |
Make the window’s context current
1 |
glfw.make_context_current(window) |
Loop until the user closes the window
1 2 3 4 5 6 |
while not glfw.window_should_close(window): glfw.poll_events() glfw.swap_buffers(window) glfw.terminate() |
In the loop code this is for swap front and back buffers
1 |
glfw.swap_buffers(window) |
This is for poll for and process the events
1 |
glfw.poll_events() |
Run the code and this will be the result
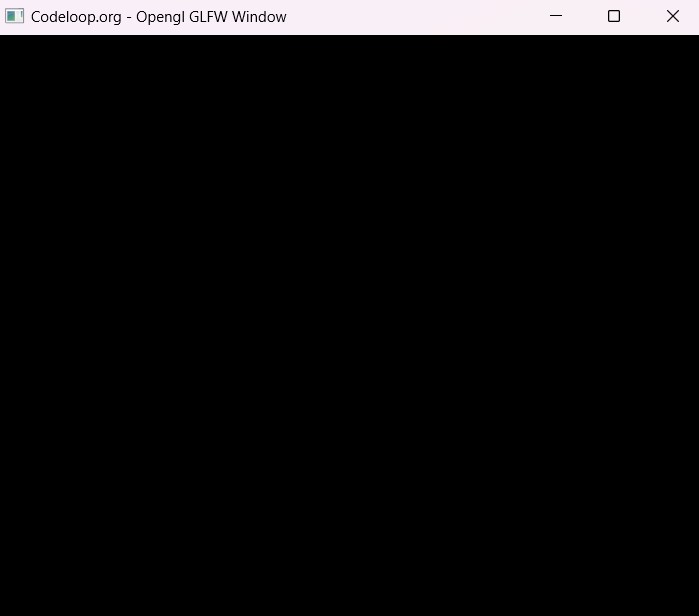
Subscribe and Get Free Video Courses & Articles in your Email