In this Python GUI article i want to show you creating Question Dialog in wxPython. So we are going to use wx.MessageDialogclass for this. and this class represents a dialog that shows a single or multi line message.
Python GUI Question Dialog in wxPython
Let’s create our example, so this is the complete code for Question Dialog in wxPython.
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17 18 19 20 21 22 23 24 25 26 27 28 29 30 31 32 33 34 35 36 37 38 39 40 41 42 43 44 45 46 |
import wx class MyFrame(wx.Frame): def __init__(self, parent, title): super(MyFrame, self).__init__(parent, title=title) # Set frame icon self.SetIcon(wx.Icon('codeloop.png', wx.BITMAP_TYPE_PNG)) self.panel = MyPanel(self) class MyPanel(wx.Panel): def __init__(self, parent): super(MyPanel, self).__init__(parent) # Creat button self.button = wx.Button(self, label="Open Question Dialog", pos=(100, 100)) # Bind button click event to questionDialog method self.Bind(wx.EVT_BUTTON, self.questionDialog) def questionDialog(self, event): # Create message dialog with a question icon dialog = wx.MessageDialog(None, "Do You Want To Close?", 'Close', wx.YES_NO | wx.ICON_QUESTION) # Show dialog and get the result result = dialog.ShowModal() # Print the result if result == wx.ID_YES: print("Yes Closed") else: print("No Close") class MyApp(wx.App): def OnInit(self): self.frame = MyFrame(parent=None, title="Codeloop.org - Message Box") self.frame.Show() return True app = MyApp() app.MainLoop() |
So this class is MyFrame class that inherits from wx.Frame and it is a top level window that contains our panel.
1 2 3 4 5 6 7 8 9 |
class MyFrame(wx.Frame): def __init__(self, parent, title): super(MyFrame, self).__init__(parent, title =title) self.panel = MyPanel(self) |
After that we have MyPanel class that inherits from wx.Panel and it is the place that we create our widgets and layouts in this class. And in this class we have created a button also we have done binding of our def questionDialog() method.
1 2 3 4 5 6 |
class MyPanel(wx.Panel): def __init__(self, parent): super(MyPanel, self).__init__(parent) self.button = wx.Button(self, label = "Open Question Dialog", pos = (100,100)) self.Bind(wx.EVT_BUTTON, self.questionDialog) |
This is the binding process for our Question Dialog
1 |
self.Bind(wx.EVT_BUTTON, self.questionDialog) |
And this is the Question Dialog method
1 2 3 4 5 6 7 8 9 |
def questionDialog(self, event): dialog = wx.MessageDialog(None, "Do You Want To Close?",'Close',wx.YES_NO | wx.ICON_QUESTION) result = dialog.ShowModal() if result == wx.ID_YES: print("Yes Closed") else: print("No Close") |
So the last class is MyApp class that inherits from wx.App. the OnInit() method is where you will most often create frame subclass objects. and start our main loop.That’s it. Once the application’s main event loop processing takes over, control passes to wxPython. Unlike procedural programs, a wxPython GUI program primarily responds to the events taking place around it, mostly determined by a human user clicking with a mouse and typing at the keyboard. When all the frames in an application have been closed, the app.MainLoop() method will return and the program will exit.
1 2 3 4 5 6 7 8 9 10 |
class MyApp(wx.App): def OnInit(self): self.frame = MyFrame(parent=None, title="Codeloop.org - Message Box") self.frame.Show() return True app = MyApp() app.MainLoop() |
So run the code and this will be the result
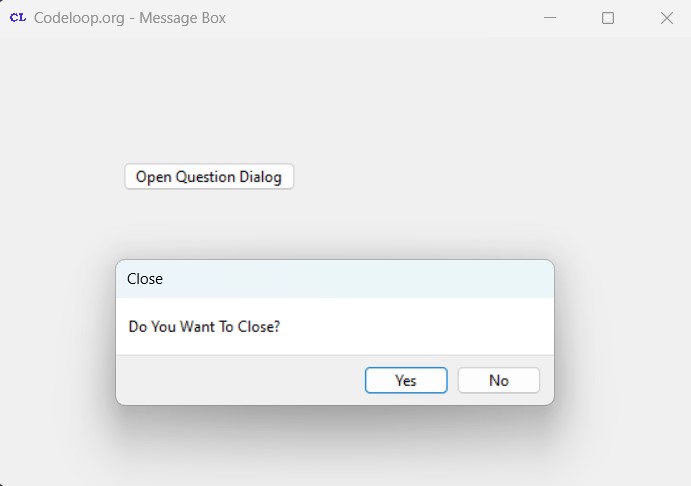
Subscribe and Get Free Video Courses & Articles in your Email