In this Python GUI article i want to show you creating Bitmap Button in wxPython. A bitmap button is a control that contains a bitmap.
So This class supports the following styles:
- wx.BU_LEFT: Left-justifies the bitmap label.
- wx.BU_TOP: Aligns the bitmap label to the top of the button.
- wx.BU_RIGHT: Right-justifies the bitmap label.
- wx.BU_BOTTOM: Aligns the bitmap label to the bottom of the button.
Python GUI Bitmap Button in wxPython
Let’s create our example, this is complete code for Python GUI Bitmap Button in wxPython
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17 18 19 20 21 22 23 24 25 26 27 28 29 30 31 32 33 34 35 36 37 38 39 40 41 42 43 44 45 46 47 48 49 50 51 52 53 54 55 56 57 58 59 60 61 62 63 |
import wx class MyFrame(wx.Frame): def __init__(self, parent, title): super(MyFrame, self).__init__(parent, title=title, size=(600, 400)) # Set the icon for the frame self.SetIcon(wx.Icon("codeloop.png", wx.BITMAP_TYPE_PNG)) # Initialize panel self.panel = MyPanel(self) class MyPanel(wx.Panel): def __init__(self, parent): super(MyPanel, self).__init__(parent) # Create vertical and horizontal box sizers vbox = wx.BoxSizer(wx.VERTICAL) hbox = wx.BoxSizer(wx.HORIZONTAL) # Load and create first bitmap button imageFile = "copy.png" image1 = wx.Image(imageFile, wx.BITMAP_TYPE_ANY).ConvertToBitmap() self.button1 = wx.BitmapButton(self, id=-1, bitmap=image1, size=(image1.GetWidth() + 40, image1.GetHeight() + 40)) self.button1.SetLabel("Copy") hbox.Add(self.button1, 0, wx.ALIGN_CENTER) self.button1.Bind(wx.EVT_BUTTON, self.OnClicked) # Load and create the second bitmap button imageFile2 = "folder.png" image2 = wx.Image(imageFile2, wx.BITMAP_TYPE_ANY).ConvertToBitmap() self.button2 = wx.BitmapButton(self, id=-1, bitmap=image2, size=(image2.GetWidth() + 40, image2.GetHeight() + 40)) self.button2.SetLabel("Folder") hbox.Add(self.button2, 0, wx.ALIGN_CENTER) self.button2.Bind(wx.EVT_BUTTON, self.OnClicked) # Load and create the third bitmap button imageFile3 = "save.png" image3 = wx.Image(imageFile3, wx.BITMAP_TYPE_ANY).ConvertToBitmap() self.button3 = wx.BitmapButton(self, id=-1, bitmap=image3, size=(image3.GetWidth() + 40, image3.GetHeight() + 40)) self.button3.SetLabel("Save") hbox.Add(self.button3, 0, wx.ALIGN_CENTER) self.button3.Bind(wx.EVT_BUTTON, self.OnClicked) # Add the horizontal sizer to the vertical sizer vbox.Add(hbox, 1, wx.ALIGN_CENTER) self.SetSizer(vbox) def OnClicked(self, event): # Event handler for button clicks btn = event.GetEventObject().GetLabel() print("Label Of Pressed Button Is", btn) class MyApp(wx.App): def OnInit(self): # Initialize the main frame self.frame = MyFrame(parent=None, title="Bitmap Button") self.frame.Show() return True # Entry point of the application app = MyApp() app.MainLoop() |
This is our MyFrame class that inherits from wx.Frame. and it is our top level window. and also we create the object of MyPanel in here.
1 2 3 |
class MyFrame(wx.Frame): def __init__(self, parent, title): super(MyFrame, self).__init__(parent, title =title, size = (600,400)) |
So this is MyPanel class that inherits from wx.Panel.this is the container class for our widgets also we can create layouts in this MyPanel class.
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17 18 19 20 21 22 23 24 25 26 27 28 29 30 31 32 33 34 35 36 37 38 39 |
class MyPanel(wx.Panel): def __init__(self, parent): super(MyPanel, self).__init__(parent) vbox = wx.BoxSizer(wx.VERTICAL) hbox = wx.BoxSizer(wx.HORIZONTAL) imageFile = "copy.png" image1 = wx.Image(imageFile, wx.BITMAP_TYPE_ANY).ConvertToBitmap() self.button1 = wx.BitmapButton(self, id=-1, bitmap=image1, size=(image1.GetWidth() +40, image1.GetHeight() + 40)) self.button1.SetLabel("Copy") hbox.Add(self.button1, 0, wx.ALIGN_CENTER) self.button1.Bind(wx.EVT_BUTTON, self.OnClicked) imageFile2 = "folder.png" image2 = wx.Image(imageFile2, wx.BITMAP_TYPE_ANY).ConvertToBitmap() self.button2 = wx.BitmapButton(self, id=-1, bitmap=image2, size=(image2.GetWidth() + 40, image1.GetHeight() + 40)) self.button2.SetLabel("Folder") hbox.Add(self.button2, 0, wx.ALIGN_CENTER) self.button2.Bind(wx.EVT_BUTTON, self.OnClicked) imageFile3 = "save.png" image3 = wx.Image(imageFile3, wx.BITMAP_TYPE_ANY).ConvertToBitmap() self.button3 = wx.BitmapButton(self, id=-1, bitmap=image3, size=(image3.GetWidth() + 40, image3.GetHeight() + 40)) self.button1.SetLabel("Save") hbox.Add(self.button3, 0, wx.ALIGN_CENTER) self.button3.Bind(wx.EVT_BUTTON, self.OnClicked) vbox.Add(hbox, 1, wx.ALIGN_CENTER) self.SetSizer(vbox) |
Now let me describe some codes in this MyPanel class. these codes are related to our layouts.
1 2 |
vbox = wx.BoxSizer(wx.VERTICAL) hbox = wx.BoxSizer(wx.HORIZONTAL) |
By using this class you can create Bitmap Button.
1 2 |
self.button2 = wx.BitmapButton(self, id=-1, bitmap=image2, size=(image2.GetWidth() + 40, image1.GetHeight() + 40)) |
Because in this article we are going to create three Bitmap Buttons, so this is the complete section for three Bitmap Buttons. and also make sure that you have three icon images in your working directory.
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17 18 19 20 21 22 23 24 25 26 27 28 29 30 |
imageFile = "copy.png" image1 = wx.Image(imageFile, wx.BITMAP_TYPE_ANY).ConvertToBitmap() self.button1 = wx.BitmapButton(self, id=-1, bitmap=image1, size=(image1.GetWidth() +40, image1.GetHeight() + 40)) self.button1.SetLabel("Copy") hbox.Add(self.button1, 0, wx.ALIGN_CENTER) self.button1.Bind(wx.EVT_BUTTON, self.OnClicked) imageFile2 = "folder.png" image2 = wx.Image(imageFile2, wx.BITMAP_TYPE_ANY).ConvertToBitmap() self.button2 = wx.BitmapButton(self, id=-1, bitmap=image2, size=(image2.GetWidth() + 40, image1.GetHeight() + 40)) self.button2.SetLabel("Folder") hbox.Add(self.button2, 0, wx.ALIGN_CENTER) self.button2.Bind(wx.EVT_BUTTON, self.OnClicked) imageFile3 = "save.png" image3 = wx.Image(imageFile3, wx.BITMAP_TYPE_ANY).ConvertToBitmap() self.button3 = wx.BitmapButton(self, id=-1, bitmap=image3, size=(image3.GetWidth() + 40, image3.GetHeight() + 40)) self.button1.SetLabel("Save") hbox.Add(self.button3, 0, wx.ALIGN_CENTER) self.button3.Bind(wx.EVT_BUTTON, self.OnClicked) vbox.Add(hbox, 1, wx.ALIGN_CENTER) self.SetSizer(vbox) |
This is simple event handling method that we are going to bind that with one of our Bitmap Button.
1 2 3 |
def OnClicked(self,event): btn = event.GetEventObject().GetLabel() print("Label Of Pressed Button Is", btn) |
Run the code this is the result for our Bitmap Button
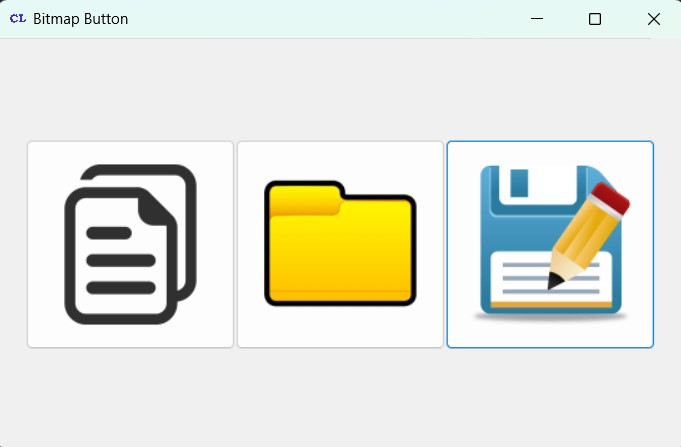
How to Create StatusBar in wxPython
FAQs:
How to add a button in wxPython?
Adding a button in wxPython is easy. You can follow these steps:
- Import the wx module: import wx.
- Create a frame or panel where you want to place the button.
- Instantiate a wx.Button object with the desired parent and label.
- Optionally, bind an event handler to the button for handling click events.
- Add the button to the frame or panel using a sizer or directly positioning it.
- And lastly show the frame or panel.
This is a basic example
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17 18 19 20 21 22 |
import wx class MyFrame(wx.Frame): def __init__(self, parent, title): super(MyFrame, self).__init__(parent, title=title, size=(300, 200)) panel = wx.Panel(self) button = wx.Button(panel, label="Click me", pos=(100, 50)) self.Bind(wx.EVT_BUTTON, self.on_button_click, button) self.Show() def on_button_click(self, event): print("Button clicked") if __name__ == "__main__": app = wx.App(False) frame = MyFrame(None, "Button Example") app.MainLoop() |
Is wxPython better than tkinter?
The selection between wxPython and Tkinter depends on different factors such as personal preference, project requirements, available documentation and community support.
These are some points to consider:
- wxPython: Offers more native look and feel across different platforms (Windows, macOS, Linux). It has a larger widgets and provides more customization options. However, the learning curve might be slightly steeper for beginners.
- Tkinter: Comes bundled with Python, and it is easily available for development without any additional installations. It has a simpler and more lightweight API compared to wxPython, and it is so easy for beginners to get started. However, it might not provide as much flexibility or advanced features as wxPython.
What are the different types of buttons in wxPython?
In wxPython, you can create different types of buttons according to your different needs. Some common types include:
- wx.Button: standard push button that triggers an action when clicked.
- wx.ToggleButton: Toggles between the pressed and released states when clicked.
- wx.BitmapButton: Displays a bitmap image as a button.
- wx.RadioButton: Part of a group of buttons where only one button can be selected at a time.
- wx.CheckBox: A box that can be checked or unchecked by the user.
- wx.ToggleButton: Toggles between two states when clicked.
- wx.BitmapToggleButton: Displays a bitmap image that toggles between two states when clicked.
Which is better, PyQt or wxPython?
Both PyQt and wxPython are popular GUI frameworks for Python programming language, and selection between them depends on different factors such as project requirements, personal preference, platform support and community resources.
These are some considerations:
- PyQt: Provides a lot of features, extensive documentation and large community. You can easily integrates that with Qt Designer for designing GUIs visually. PyQt applications have a native look and feel across multiple platforms. However, PyQt is licensed under the GPL or a commercial license.
- wxPython: Offers native look and feel across platforms, different widget set, and good documentation. It has also open-source license (wxWindows License), it means that using wxPython you can build open source or commercial applications. However development pace might be slower compared to PyQt, and the documentation might not be as extensive.
Subscribe and Get Free Video Courses & Articles in your Email