In this OpenCV article i want to talk about Python Eye Detection with OpenCV. so for this article we are using Haar Cascade Classifier.
What is Eye Detection In OpenCV?
Eye detection with OpenCV is the process of identifying and locating eyes inside images or video frames using OpenCV library. It is a computer vision technique commonly used in different applications, such as face detection, gaze tracking and driver drowsiness detection systems.
Applications of eye detection with OpenCV are:
- Face recognition systems: Identifying the eyes is an important step in recognizing faces, as they provide valuable information for feature extraction and comparison.
- Gaze tracking: Tracking the movement and direction of the eyes can be used in human-computer interaction systems, virtual reality applications and assistive technologies.
- Driver monitoring systems: Detecting and tracking the driver’s eyes can help in detecting drowsiness, distraction, or fatigue, and it enhance road safety.
- Biometric authentication: Eye features, such as iris patterns are unique to individuals and can be used for biometric authentication in security systems.
What is Haar Cascade ?
A Haar Cascade is basically a classifier which is used to detect the object for which it has been trained for, from the source. The Haar Cascade is trained by superimposing the positive image over a set of negative images. The training is generally done on a server and on various stages. Better results are obtained by using high quality images and increasing the amount of stages for which the classifier is trained.
You can also used predefined Haar Cascades which are available on github.
Python Eye Detection with OpenCV
Now let’s create our example, This is the complete code for Python Eye Detection With OpenCV.
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17 18 19 20 21 22 23 |
import cv2 # Load input image image = cv2.imread("lena.tif") # Load Haar cascade classifier for eye detection eye_cascade = cv2.CascadeClassifier('haarcascade_eye.xml') # Detect eyes in the image using the detectMultiScale function # scaleFactor and minNeighbors are parameters that # affect the detection sensitivity eyes = eye_cascade.detectMultiScale(image, scaleFactor=1.1, minNeighbors=5) # Iterate over detected eyes and draw rectangles around them for (ex, ey, ew, eh) in eyes: cv2.rectangle(image, (ex, ey), (ex+ew, ey+eh), (255, 0, 0), 5) # Display image with detected eyes cv2.imshow("Eye Detected", image) # Wait for key press and close all windows cv2.waitKey(0) cv2.destroyAllWindows() |
So in this line of code we have loaded the image, you need to have an image in your working directory.
1 |
image = cv2.imread("lena.tif") |
This is for loading our Haar Cascade Classifier that we have already copied in our directory
1 |
eye_cascade = cv2.CascadeClassifier('haarcascade_eye.xml') |
detecMultiScale() function is for detecting objects if it finds a face in the image it will return in the form of x,y,w,h. and it needs some parameters.
ScaleFactor: This is parameter is for specifying how much the image size is reduced at each image scale.
minNeighbors: Parameter specifying how many neighbors each candidate rectangle should have to retain it, this parameter will affect the quality of the detected faces.
1 |
eyes = eye_cascade.detectMultiScale(image, scaleFactor = 1.1, minNeighbors = 5) |
So in this code we want to draw rectangle in the eyes in image
1 2 |
for (ex,ey,ew,eh) in eyes: cv2.rectangle(image,(ex,ey),(ex+ew,ey+eh),(255,0,0),5) |
In this line of code we want to show our image
1 |
cv2.imshow("Eye Detected", image) |
So run the complete code and this will be the result
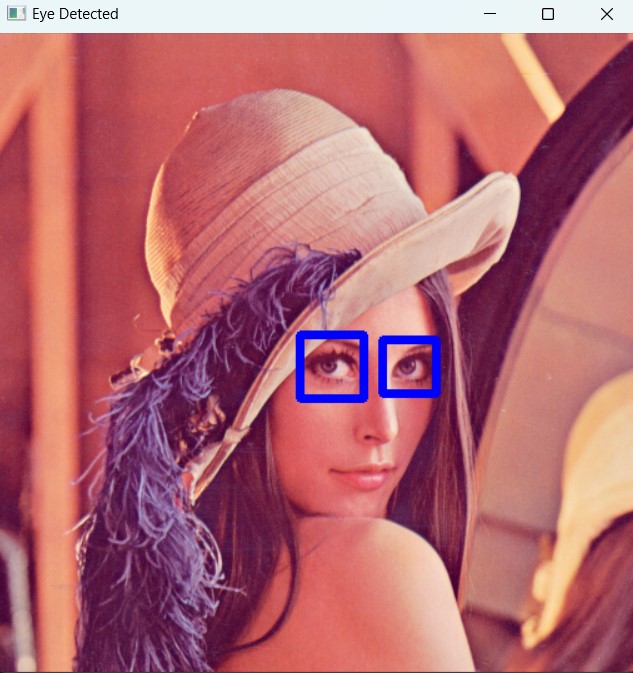
FAQs:
How to detect face using OpenCV Python?
For detecting faces using OpenCV in Python, you can use the Haar Cascade classifier, a pre-trained model for face detection. Here’s a basic outline of the steps:
- Load the Haar Cascade classifier.
- Read the image.
- Convert the image to grayscale.
- Detect faces using the classifier.
- Draw rectangles around the detected faces.
- Display the result.
What is face recognition in Python?
Face recognition in Python is the process of identifying or verifying a person from a digital image or a video frame. It involves detecting a face in the image and after that comparing it with stored faces to find a match. The process typically uses deep learning techniques and libraries like dlib, face_recognition, and OpenCV.
- Face Detection: Locating the face in the image.
- Feature Extraction: Extracting unique features from the face (e.g., using a deep neural network).
- Face Matching: Comparing extracted features with known faces to find a match.
Subscribe and Get Free Video Courses & Articles in your Email
excellent! do u have a c++ example of the eye cascade using video?