In this PyQt6 Tutorial we want to learn How to Create TextBox in PyQt6, so PyQt6 is Python binding for the popular Qt framework, PyQt6 provides a powerful toolkit for developing GUI applications, and PyQt6 TextBox allows users to input and display text.
First of all you need to install PyQt6 and you can use pip for that.
1 |
pip install PyQt6 |
First of all we need to import our required modules and classes.
1 2 |
from PyQt6.QtWidgets import QApplication, QMainWindow, QTextEdit import sys |
After that we need to create an application instance and a main window. The main window will act as the container for our textbox.
1 2 3 |
app = QApplication(sys.argv) window = QMainWindow() window.setWindowTitle("Codeloop.org - TextBox Example") |
Now that we have our main window set up, let’s create the textbox itself. we want to use the QTextEdit class to achieve this, in this code we creates an instance of QTextEdit and associate it with our main window. after that we use the setGeometry() method to specify the position and size of the textbox within the window.
1 2 |
textbox = QTextEdit(window) textbox.setGeometry(10, 10, 280, 180) |
To provide an initial hint or instruction to the user, you can set a placeholder text for the textbox using the setPlaceholderText() method.
1 |
textbox.setPlaceholderText("Enter your text here...") |
If you want to prevent the user from modifying the textbox content, you can enable the readonly mode using the setReadOnly() method.
1 |
textbox.setReadOnly(True) |
This is the complete code for this article
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17 18 19 20 21 22 23 24 25 26 27 28 29 30 31 32 33 34 35 36 37 38 |
# Import necessary classes from PyQt6.QtWidgets and PyQt6.QtGui from PyQt6.QtWidgets import QApplication, QMainWindow, QTextEdit import sys # Import sys module to handle system-level operations from PyQt6.QtGui import QIcon # Import QIcon class to set window icon def create_textbox(): # Create an instance of QApplication app = QApplication(sys.argv) # Create main window for the application window = QMainWindow() # Set title of the main window window.setWindowTitle("Codeloop.org - TextBox Example") # Set icon for the main window window.setWindowIcon(QIcon("codeloop.png")) # Create QTextEdit widget textbox = QTextEdit(window) # Set the position and size textbox.setGeometry(10, 10, 280, 180) # Set placeholder text textbox.setPlaceholderText("Enter your text here...") # Display main window on the screen window.show() # Enter the main event loop sys.exit(app.exec()) if __name__ == '__main__': # Call the function create_textbox() |
Run the complete code and this will be the result
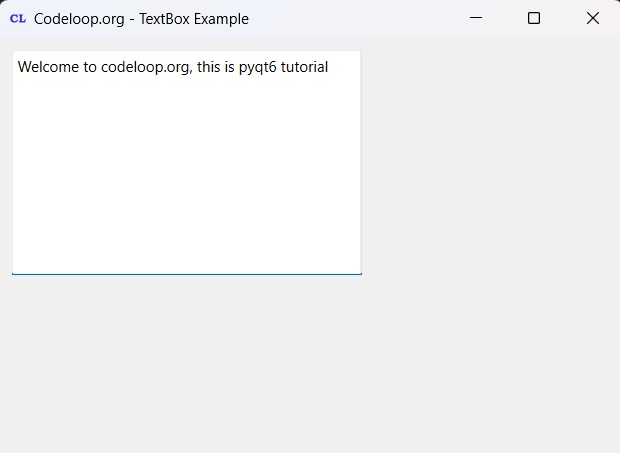
Subscribe and Get Free Video Courses & Articles in your Email