In this PyQt5 article i want to show you creating QMessageBox In PyQt5 with Practical Example. a message box displays a primary text to alert the user to a situation, an informative text to further explain the alert or to ask the user a question, and an optional detailed text to provide even more data if the user requests it. A message box can also display an icon and standard buttons for accepting a user response.
What is PyQt5 QMessageBox ?
In PyQt5, QMessageBox is a dialog box that displays a message to the user and provides a set of standard buttons for the user to choose from. It is commonly used to display informative messages, warnings, errors, or to ask the user for confirmation.
QMessageBox provides a set of standard buttons, such as OK, Cancel, Yes, No, and Apply, which are displayed in a button box at the bottom of the dialog box. You can use these buttons to give the user a choice or to request confirmation for an action.
Also you can read more Python GUI articles in the below links
- Kivy GUI Development Tutorials
- TKinter GUI Development Tutorials
- Pyside2 GUI Development
- wxPython GUI Development Tutorials
- PyQt5 GUI Development Tutorials
First of we need some imports classes from PyQt5 library.
1 2 3 4 5 6 |
from PyQt5 import QtGui from PyQt5.QtWidgets import QApplication, QMainWindow, QAction, QTextEdit, QFontDialog, QColorDialog, QFileDialog, QMessageBox import sys from PyQt5.QtGui import QIcon from PyQt5.QtPrintSupport import QPrintDialog, QPrinter, QPrintPreviewDialog from PyQt5.Qt import QFileInfo |
After that we are going to create our main Window class that extends from QMainWindow and in that class we add our basic requirements of our window . there are some codes from the previous articles that i have already described. we are going to just focus on creating QMessageBox, and basically there are different messagebox that you can use in PyQt5.
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17 18 19 20 21 22 23 24 25 26 27 28 29 30 31 32 33 34 35 36 37 38 39 40 41 42 43 44 45 46 47 48 49 50 51 52 53 54 55 56 57 58 59 60 61 62 63 64 65 66 67 68 69 70 71 72 73 74 75 76 77 78 79 80 81 82 83 84 85 86 87 88 89 90 91 92 93 94 95 96 97 98 99 100 101 102 103 104 105 106 107 |
class Window(QMainWindow): def __init__(self): super().__init__() self.title = "PyQt5 QToolbar" self.top = 200 self.left = 500 self.width = 680 self.height = 480 self.setWindowIcon(QtGui.QIcon("icon.png")) self.setWindowTitle(self.title) self.setGeometry(self.left, self.top, self.width, self.height) self.createEditor() self.CreateMenu() self.show() def CreateMenu(self): mainMenu = self.menuBar() fileMenu = mainMenu.addMenu('File') editMenu = mainMenu.addMenu('Edit') viewMenu = mainMenu.addMenu('View') helpMenu = mainMenu.addMenu('Help') printAction = QAction(QIcon("print.png"), "Print", self) printAction.triggered.connect(self.printDialog) fileMenu.addAction(printAction) printPreviewAction = QAction(QIcon("printprev.png"), "Print Preview", self) printPreviewAction.triggered.connect(self.printpreviewDialog) fileMenu.addAction(printPreviewAction) exportpdfAction = QAction(QIcon("pdf.png"), "Export PDF", self) exportpdfAction.triggered.connect(self.printPDF) fileMenu.addAction(exportpdfAction) exiteAction = QAction(QIcon("exit.png"), 'Exit', self) exiteAction.setShortcut("Ctrl+E") exiteAction.triggered.connect(self.exitWindow) fileMenu.addAction(exiteAction) copyAction = QAction(QIcon("copy.png"), 'Copy', self) copyAction.setShortcut("Ctrl+C") editMenu.addAction(copyAction) saveAction = QAction(QIcon("Save.png"), 'Save', self) saveAction.setShortcut("Ctrl+S") editMenu.addAction(saveAction) pasteAction = QAction(QIcon("Paste.png"), 'Paste', self) pasteAction.setShortcut("Ctrl+P") editMenu.addAction(pasteAction) fontAction = QAction(QIcon("font.png"), "Font", self) fontAction.setShortcut("Ctrl+F") fontAction.triggered.connect(self.fontDialog) viewMenu.addAction(fontAction) colorAction = QAction(QIcon("color.png"), "Color", self) colorAction.triggered.connect(self.colorDialog) viewMenu.addAction(colorAction) helpAction = QAction(QIcon('about.png'), "About Application", self) helpAction.triggered.connect(self.AboutmessageBox) helpMenu.addAction(helpAction) choiceAction = QAction(QIcon('new.png'), "Choice MessageBox", self) choiceAction.triggered.connect(self.choiceMessageBox) helpMenu.addAction(choiceAction) self.toolbar = self.addToolBar('Toolbar') self.toolbar.addAction(copyAction) self.toolbar.addAction(saveAction) self.toolbar.addAction(pasteAction) self.toolbar.addAction(exiteAction) self.toolbar.addAction(fontAction) self.toolbar.addAction(colorAction) self.toolbar.addAction(printAction) self.toolbar.addAction(printPreviewAction) self.toolbar.addAction(exportpdfAction) self.toolbar.addAction(helpAction) def exitWindow(self): self.close() def createEditor(self): self.textEdit = QTextEdit(self) self.setCentralWidget(self.textEdit) def fontDialog(self): font, ok = QFontDialog.getFont() if ok: self.textEdit.setFont(font) def colorDialog(self): color = QColorDialog.getColor() self.textEdit.setTextColor(color) def printDialog(self): printer = QPrinter(QPrinter.HighResolution) dialog = QPrintDialog(printer, self) if dialog.exec_() == QPrintDialog.Accepted: self.textEdit.print_(printer) def printpreviewDialog(self): printer = QPrinter(QPrinter.HighResolution) previewDialog = QPrintPreviewDialog(printer, self) previewDialog.paintRequested.connect(self.printPreview) previewDialog.exec_() def printPreview(self, printer): self.textEdit.print_(printer) def printPDF(self): fn, _ = QFileDialog.getSaveFileName(self, 'Export PDF', None, 'PDF files (.pdf);;All Files()') if fn != '': if QFileInfo(fn).suffix() == "": fn += '.pdf' printer = QPrinter(QPrinter.HighResolution) printer.setOutputFormat(QPrinter.PdfFormat) printer.setOutputFileName(fn) self.textEdit.document().print_(printer) |
so this is the method for creating of QMessageBox and we are going to create about messagebox in here.
1 2 3 4 5 6 7 8 9 |
def AboutmessageBox(self): QMessageBox.about(self, "About Application", "This is a simple texteditor application") def choiceMessageBox(self): message = QMessageBox.question(self, "Choice Message", "Do You Like PyQt5 ?", QMessageBox.Yes | QMessageBox.No, QMessageBox.No) if message == QMessageBox.Yes: print("Yes I Like PyQt5") else: print("No I Dot Like PyQt5") |
Also every PyQt5 application must create an application object.
1 |
App = QApplication(sys.argv) |
Finally, we enter the mainloop of the application. The event handling starts from this point.
1 2 |
window = Window() sys.exit(App.exec()) |
This is the result image for the complete code.
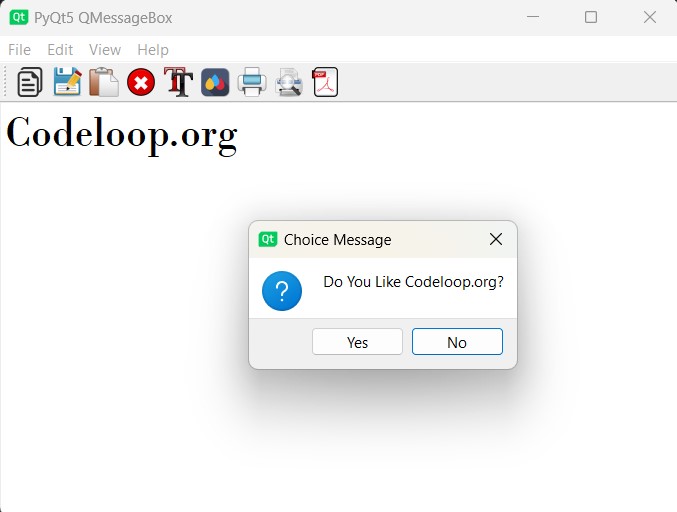
Complete source code for this article
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17 18 19 20 21 22 23 24 25 26 27 28 29 30 31 32 33 34 35 36 37 38 39 40 41 42 43 44 45 46 47 48 49 50 51 52 53 54 55 56 57 58 59 60 61 62 63 64 65 66 67 68 69 70 71 72 73 74 75 76 77 78 79 80 81 82 83 84 85 86 87 88 89 90 91 92 93 94 95 96 97 98 99 100 101 102 103 104 105 106 107 108 109 110 111 112 113 114 115 116 117 118 119 120 121 122 123 124 125 126 127 128 129 130 131 132 133 134 135 136 137 138 139 140 141 142 143 144 145 146 147 148 149 150 151 152 153 154 155 156 157 158 159 160 161 162 163 164 165 166 |
from PyQt5 import QtGui from PyQt5.QtWidgets import QApplication, QMainWindow, QAction, QTextEdit, QFontDialog, QColorDialog, QFileDialog, \ QMessageBox import sys from PyQt5.QtGui import QIcon from PyQt5.QtPrintSupport import QPrintDialog, QPrinter, QPrintPreviewDialog from PyQt5.Qt import QFileInfo class Window(QMainWindow): def __init__(self): super().__init__() # Set window properties self.title = "PyQt5 QMessageBox" self.top = 200 self.left = 500 self.width = 680 self.height = 480 self.setWindowIcon(QtGui.QIcon("icon.png")) self.setWindowTitle(self.title) self.setGeometry(self.left, self.top, self.width, self.height) # Create the text editor and menu self.createEditor() self.CreateMenu() self.show() # Method to create the menu def CreateMenu(self): mainMenu = self.menuBar() fileMenu = mainMenu.addMenu('File') editMenu = mainMenu.addMenu('Edit') viewMenu = mainMenu.addMenu('View') helpMenu = mainMenu.addMenu('Help') # Add actions to File menu printAction = QAction(QIcon("print.png"), "Print", self) printAction.triggered.connect(self.printDialog) fileMenu.addAction(printAction) printPreviewAction = QAction(QIcon("printprev.png"), "Print Preview", self) printPreviewAction.triggered.connect(self.printpreviewDialog) fileMenu.addAction(printPreviewAction) exportpdfAction = QAction(QIcon("pdf.png"), "Export PDF", self) exportpdfAction.triggered.connect(self.printPDF) fileMenu.addAction(exportpdfAction) exiteAction = QAction(QIcon("exit.png"), 'Exit', self) exiteAction.setShortcut("Ctrl+E") exiteAction.triggered.connect(self.exitWindow) fileMenu.addAction(exiteAction) # Add actions to Edit menu copyAction = QAction(QIcon("copy.png"), 'Copy', self) copyAction.setShortcut("Ctrl+C") editMenu.addAction(copyAction) saveAction = QAction(QIcon("Save.png"), 'Save', self) saveAction.setShortcut("Ctrl+S") editMenu.addAction(saveAction) pasteAction = QAction(QIcon("Paste.png"), 'Paste', self) pasteAction.setShortcut("Ctrl+P") editMenu.addAction(pasteAction) # Add actions to View menu fontAction = QAction(QIcon("font.png"), "Font", self) fontAction.setShortcut("Ctrl+F") fontAction.triggered.connect(self.fontDialog) viewMenu.addAction(fontAction) colorAction = QAction(QIcon("color.png"), "Color", self) colorAction.triggered.connect(self.colorDialog) viewMenu.addAction(colorAction) # Add actions to Help menu helpAction = QAction(QIcon('about.png'), "About Application", self) helpAction.triggered.connect(self.AboutmessageBox) helpMenu.addAction(helpAction) choiceAction = QAction(QIcon('new.png'), "Choice MessageBox", self) choiceAction.triggered.connect(self.choiceMessageBox) helpMenu.addAction(choiceAction) # Add actions to the toolbar self.toolbar = self.addToolBar('Toolbar') self.toolbar.addAction(copyAction) self.toolbar.addAction(saveAction) self.toolbar.addAction(pasteAction) self.toolbar.addAction(exiteAction) self.toolbar.addAction(fontAction) self.toolbar.addAction(colorAction) self.toolbar.addAction(printAction) self.toolbar.addAction(printPreviewAction) self.toolbar.addAction(exportpdfAction) self.toolbar.addAction(helpAction) # Method to exit the window def exitWindow(self): self.close() # Method to create the text editor def createEditor(self): self.textEdit = QTextEdit(self) self.setCentralWidget(self.textEdit) # Method to open font dialog and set font def fontDialog(self): font, ok = QFontDialog.getFont() if ok: self.textEdit.setFont(font) # Method to open color dialog and set text color def colorDialog(self): color = QColorDialog.getColor() self.textEdit.setTextColor(color) # Method to open print dialog and print text def printDialog(self): printer = QPrinter(QPrinter.HighResolution) dialog = QPrintDialog(printer, self) if dialog.exec_() == QPrintDialog.Accepted: self.textEdit.print_(printer) # Method to open print preview dialog def printpreviewDialog(self): printer = QPrinter(QPrinter.HighResolution) previewDialog = QPrintPreviewDialog(printer, self) previewDialog.paintRequested.connect(self.printPreview) previewDialog.exec_() # Method to handle printing in print preview dialog def printPreview(self, printer): self.textEdit.print_(printer) # Method to print text as PDF def printPDF(self): fn, _ = QFileDialog.getSaveFileName(self, 'Export PDF', None, 'PDF files (.pdf);;All Files()') if fn != '': if QFileInfo(fn).suffix() == "": fn += '.pdf' printer = QPrinter(QPrinter.HighResolution) printer.setOutputFormat(QPrinter.PdfFormat) printer.setOutputFileName(fn) self.textEdit.document().print_(printer) # Method to display about message box def AboutmessageBox(self): QMessageBox.about(self, "About Application", "This is a simple text editor application") # Method to display choice message box def choiceMessageBox(self): message = QMessageBox.question(self, "Choice Message", "Do You Like Codeloop.org?", QMessageBox.Yes | QMessageBox.No, QMessageBox.No) if message == QMessageBox.Yes: print("Yes, I Like PyQt5") else: print("No, I Don't Like PyQt5") # Create a PyQt application instance App = QApplication(sys.argv) # Create an instance of Window class window = Window() # Execute the application sys.exit(App.exec()) |
FAQs:
What is PyQt5?
PyQt5 is a set of Python bindings for Qt application framework, and it was developed by Riverbank Computing. It allows you to create cross-platform desktop applications with Python using Qt toolkit. PyQt5 provides to different functionality of the underlying Qt framework, and you can use that for creation of powerful and feature-rich graphical user interfaces (GUIs). It includes modules for handling GUI elements, multimedia, network communication, database integration and more.
Subscribe and Get Free Video Courses & Articles in your Email