In this PyQt5 article i want to show how to Open Second Dialog By Clicking Button in PyQt5. we are going to use QDialog class for this article, a dialog window is a top level window mostly used for short-term tasks and brief communications with the user. QDialogs may be modal or modeless.
What is QDialog ?
QDialog class is subclass of the QWidget class in PyQt5. it provides dialog window that can be used to display messages, ask for user input, or perform specific actions.
Dialogs are commonly used in graphical user interface (GUI) applications to interact with users, prompt them for input, and display messages or alerts. QDialog class provides different features and functionality for creating custom dialogs, including:
- Built-in standard dialogs for common tasks, such as file selection or message display.
- Customizable buttons, labels, and input fields.
- Support for modal and non-modal dialogs.
- Custom dialog styles, including custom backgrounds, fonts, and colors.
- Support for multiple windows and layouts within a dialog.
- Access to the parent window and application, allowing for easy integration with other components.
In addition, the QDialog class provides several signals and slots for communicating with other widgets and components in the application, making it a powerful tool for building complex and interactive user interfaces.
Also you can read more Python GUI articles in the below links
- Kivy GUI Development Tutorials
- TKinter GUI Development Tutorials
- Psyide2 GUI Development
- wxPython GUI Development Tutorials
- PyQt5 GUI Development Tutorials
So now this is the complete code for PyQt5 Open Second Dialog By Clicking Button.
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17 18 19 20 21 22 23 24 25 26 27 28 29 30 31 32 33 34 35 36 37 38 39 40 41 42 43 44 45 46 47 48 49 50 51 52 53 |
from PyQt5 import QtGui from PyQt5.QtWidgets import QApplication, QWidget, QPushButton, QDialog, QVBoxLayout import sys class Window(QWidget): def __init__(self): super().__init__() self.title = "PyQt5 Window" self.top = 200 self.left = 500 self.width = 400 self.height = 300 self.InitWindow() def InitWindow(self): self.setWindowIcon(QtGui.QIcon("icon.png")) self.setWindowTitle(self.title) self.setGeometry(self.left, self.top, self.width, self.height) vbox = QVBoxLayout() self.btn = QPushButton("Open Second Dialog") self.btn.setFont(QtGui.QFont("Sanserif", 15)) self.btn.clicked.connect(self.openSecondDialog) vbox.addWidget(self.btn) self.setLayout(vbox) self.show() def openSecondDialog(self): mydialog = QDialog(self) #mydialog.setModal(True) #mydialog.exec() mydialog.show() App = QApplication(sys.argv) window = Window() sys.exit(App.exec_()) |
These are the initialization of our window requirements like title and geometry.
1 2 3 4 5 |
self.title = "PyQt5 Window" self.top = 200 self.left = 500 self.width = 400 self.height = 300 |
OK now in here we are going to set our window icon, window title and window geometry. make sure that you have added an icon to your working directory.
1 2 3 |
self.setWindowIcon(QtGui.QIcon("icon.png")) self.setWindowTitle(self.title) self.setGeometry(self.left, self.top, self.width, self.height) |
Also we need to create a layout and we are going to use a QVBoxLayout.
1 |
vbox = QVBoxLayout() |
In here we have created the object of QPushButton, because we want when a user click on the button a new dialog will be open. also we have connected the clicked signal of QPushButton with the openSecondDialog() slot or method.
1 2 3 |
self.btn = QPushButton("Open Second Dialog") self.btn.setFont(QtGui.QFont("Sanserif", 15)) self.btn.clicked.connect(self.openSecondDialog) |
Also we need to add our button to the QVBoxLayout also we have set the vbox layout for our main window.
1 2 |
vbox.addWidget(self.btn) self.setLayout(vbox) |
Also every PyQt5 application must create an application object.
1 |
App = QApplication(sys.argv) |
Finally, we enter the mainloop of the application. The event handling starts from this point.
1 2 |
window = Window() sys.exit(App.exec()) |
Run the complete code and this will be the result.
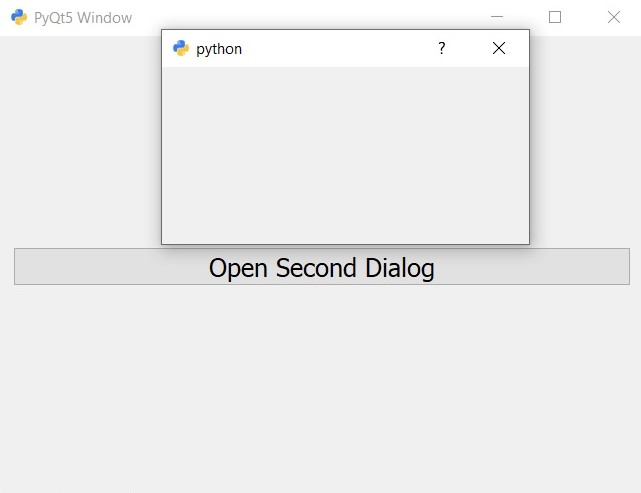
Also you can watch the complete video for this article
Subscribe and Get Free Video Courses & Articles in your Email