In this PyQt5 article we are going to learn about Creating QCompleter Practical Example. the QCompleter object is used to provide auto-completions when text is entered into some widgets such as the LineEdit or ComboBox. when user begins to type, the model content is matched and suggestions are provided.
What is QCompleter in PyQt5 ?
QCompleter is a widget provided by PyQt5 that provides automatic completion for user input based on provided list of possible values. it is typically used in text input fields such as QLineEdit or QTextEdit to help users enter values more efficiently and accurately.
When QCompleter widget is attached to text input field, it provides drop down list of possible completions based on the current input. after that the users can select completion from the list or continue typing their own input. QCompleter widget can be customized to match the specific needs of the application, including:
- Providing a custom list of possible completions.
- Customizing the appearance and behavior of the drop down list.
- Handling user input events such as filtering the list based on the current input or selecting a completion.
QCompleter is often used in applications that require data entry, such as address books, search fields, or form input. It can also be used to provide autocompletion for command-line interfaces or other text based interfaces.
Also you can read more Python GUI articles in the below links
- Kivy GUI Development Tutorials
- TKinter GUI Development Tutorials
- Psyide2 GUI Development
- wxPython GUI Development Tutorials
- PyQt5 GUI Development Tutorials
These are the imports that we need for this article.
1 2 3 |
from PyQt5 import QtGui from PyQt5.QtWidgets import QApplication, QDialog, QVBoxLayout, QCompleter, QLineEdit import sys |
This is our main window class that extends from window class and we initialize some window requirements like title and geometry . also we call our InitWindow() method in this class.
1 2 3 4 5 6 7 8 9 10 11 12 |
class Window(QDialog): def __init__(self): super().__init__() self.title = "PyQt5 QCompleter" self.top = 200 self.left = 500 self.width = 300 self.height = 200 self.InitWindow() |
These line of codes are setting of our window geometry , window title and window icon. also we have called our Completer() method in here.
1 2 3 4 5 |
self.setWindowIcon(QtGui.QIcon("icon.png")) self.setWindowTitle(self.title) self.setGeometry(self.left, self.top, self.width, self.height) self.Completer() |
So now in here we create the object of QVBoxLayout and we have created a list of countries, because we want to add that list in our completer. also we need to create QLineEdit object.
1 2 3 4 5 6 7 |
vbox = QVBoxLayout() names = ["Afghanistan", "India", "Pakistan", "Japan", "Indonesia", "China", "UAE", "America"] completer = QCompleter(names) self.lineedit = QLineEdit() self.lineedit.setCompleter(completer) |
In here we have added our QLineEdit in the vbox layout and we set the vbox layout to the main window.
1 2 |
vbox.addWidget(self.lineedit) self.setLayout(vbox) |
Also every PyQt5 application must create an application object.
1 |
App = QApplication(sys.argv) |
Finally, we enter the mainloop of the application. The event handling starts from this point.
1 2 |
window = Window() sys.exit(App.exec()) |
Complete source for the QCompleter
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17 18 19 20 21 22 23 24 25 26 27 28 29 30 31 32 33 34 35 36 37 38 39 40 41 42 43 44 45 46 47 48 49 50 |
from PyQt5 import QtGui from PyQt5.QtWidgets import QApplication, QDialog, QVBoxLayout, QCompleter, QLineEdit import sys class Window(QDialog): def __init__(self): super().__init__() self.title = "PyQt5 QCompleter" self.top = 200 self.left = 500 self.width = 300 self.height = 200 self.InitWindow() def InitWindow(self): self.setWindowIcon(QtGui.QIcon("icon.png")) self.setWindowTitle(self.title) self.setGeometry(self.left, self.top, self.width, self.height) self.Completer() self.show() def Completer(self): vbox = QVBoxLayout() names = ["Afghanistan", "India", "Pakistan", "Japan", "Indonesia", "China", "UAE", "America"] completer = QCompleter(names) self.lineedit = QLineEdit() self.lineedit.setCompleter(completer) vbox.addWidget(self.lineedit) self.setLayout(vbox) App = QApplication(sys.argv) window = Window() sys.exit(App.exec_()) |
Run the code and this is the result
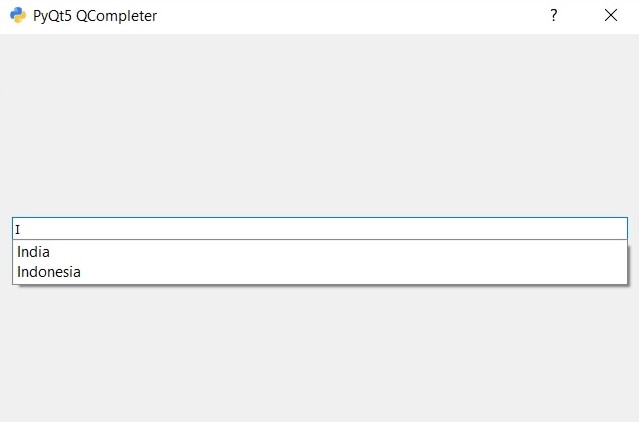
Also you can watch the complete video for this article
Subscribe and Get Free Video Courses & Articles in your Email