In this Pygame Tutorial we want to learn Drawing Rectangle in Pygame, if you are creating a game or any other kind of interactive program with Pygame, some times you will need to draw rectangles. Rectangles are useful for creating game objects, UI elements and much more. in this article we want to talk that how to draw rectangles using pygame.draw.rect() function.
First of all you need to import Pygame module and initialize it with pygame.init() function.
1 2 |
import pygame pygame.init() |
So before you draw a rectangle, you need to set dimensions of the Pygame display. you can do this byusing pygame.display.set_mode() function.
1 2 3 |
display_width = 800 display_height = 600 game_display = pygame.display.set_mode((display_width, display_height)) |
Now we are ready to draw rectangle, pygame.draw.rect() function takes several arguments, including display surface to draw on, color of the rectangle, position of the top left corner of rectangle and dimensions of the rectangle. this is an example of how to draw a rectangle:
1 2 3 |
# Draw red rectangle at (100, 100) with a # width of 50 and a height of 100 pygame.draw.rect(game_display, (255, 0, 0), (100, 100, 50, 100)) |
After drawing a rectangle, you need to update Pygame display for the changes to take effect. you can do this with pygame.display.update() function.
1 |
pygame.display.update() |
This is the complete code for this article
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17 18 19 20 21 22 23 24 25 26 27 28 29 30 31 |
import pygame pygame.init() # Set dimensions of the display window display_width = 800 display_height = 600 game_display = pygame.display.set_mode((display_width, display_height)) # Draw red rectangle at (100, 100) # with a width of 50 and height of 100 pygame.draw.rect(game_display, (255, 0, 0), (100, 100, 50, 100)) # Updat display pygame.display.update() # Game loop game_exit = False while not game_exit: for event in pygame.event.get(): if event.type == pygame.QUIT: game_exit = True # Your game code here # Update display pygame.display.update() # Quit Pygame pygame.quit() quit() |
This code initializes Pygame and sets dimensions of the display window. after that it draws red rectangle using pygame.draw.rect(). and finally this code enters a game loop that continually updates the display until the user closes the window.
Run the complete code and this will be the result
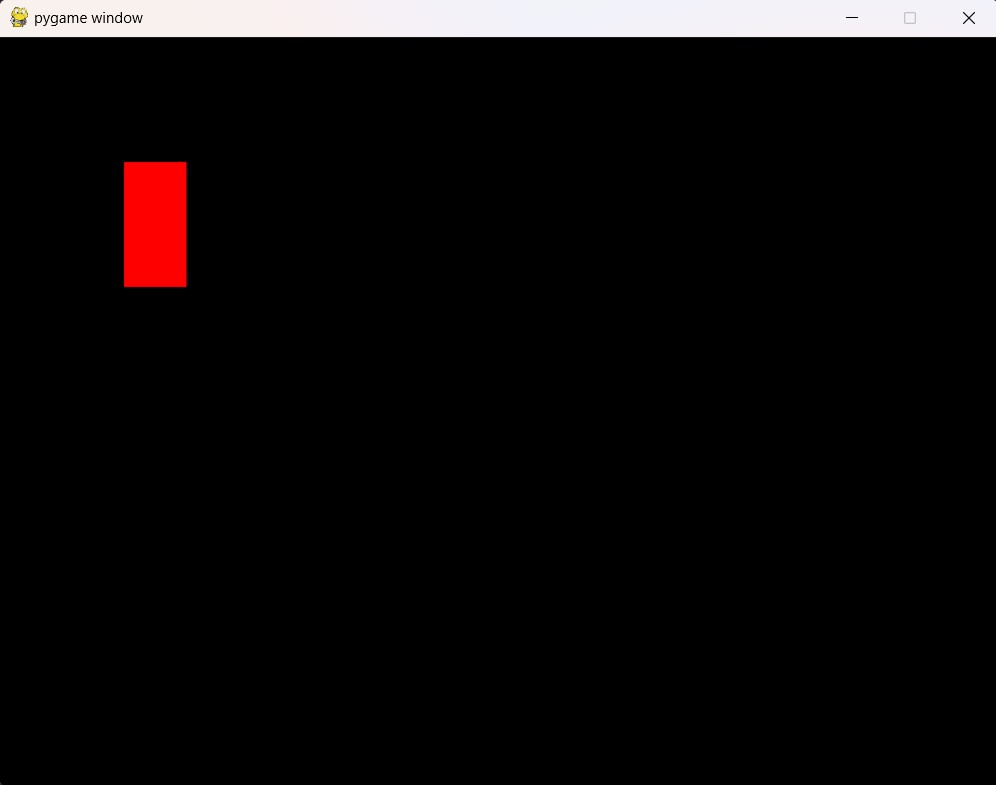
Subscribe and Get Free Video Courses & Articles in your Email