In this Python OpenCV article we are going to learn about Image Scaling with OpenCV,
so Scaling is just resizing of the image. OpenCV comes with a function cv2.resize() for this
purpose. The size of the image can be specified manually, or you can specify the scaling factor.
Different interpolation methods are used. Preferable interpolation methods
are cv2.INTER_AREA for shrinking and cv2.INTER_CUBIC (slow) & cv2.INTER_LINEAR
for zooming. By default, the interpolation method cv2.INTER_LINEAR is used for all resizing
purposes. You can resize an input image with either of following methods.
Learn How to Rotate an Image in OpenCV Python
Read Image Smoothing Techniques in OpenCV
1: OpenCV Averaging Image Blurring in Python
2: OpenCV Gaussian Blurring for Images in Python
3: OpenCV Median Blurring for Images in Python
4: OpenCV Smooth Image with Bilateral Filtering
So now this is the complete code for OpenCV Image Scaling with Python
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17 |
img = cv2.imread('lena.tif') img_scaled = cv2.resize(img,None,fx=1.2, fy=1.2, interpolation = cv2.INTER_LINEAR) cv2.imshow('Scaling Linear Interpolation', img_scaled) img_scaled=cv2.resize(img,None,fx=1.2, fy=1.2, interpolation = cv2.INTER_CUBIC) cv2.imshow('Scaling Cubic Interpolation', img_scaled) img_scaled = cv2.resize(img,(450, 400), interpolation = cv2.INTER_AREA) cv2.imshow('Scaling Skewed Size', img_scaled) cv2.waitKey(0) cv2.destroyAllWindows() |
This line of code is used for reading of the image, make sure that you have added an image
in your working directory.
1 |
img = cv2.imread('lena.tif') |
So we can use cv2.resize() method for image resizing, you need to give some parameters like
image source , dsize, factor x and factor y also interpolation.
1 2 3 4 5 6 7 8 9 10 11 12 |
img_scaled = cv2.resize(img,None,fx=1.2, fy=1.2, interpolation = cv2.INTER_LINEAR) cv2.imshow('Scaling Linear Interpolation', img_scaled) img_scaled=cv2.resize(img,None,fx=1.2, fy=1.2, interpolation = cv2.INTER_CUBIC) cv2.imshow('Scaling Cubic Interpolation', img_scaled) img_scaled = cv2.resize(img,(450, 400), interpolation = cv2.INTER_AREA) cv2.imshow('Scaling Skewed Size', img_scaled) |
Note: cv2.waitKey() is a keyboard binding function. Its argument is the time in milliseconds.
the function waits specified milliseconds for any keyboard event. If you press any key in that
time, the program continues. If 0 is passed, it waits indefinitely for a key stroke.
So run the complete code and this is the result.
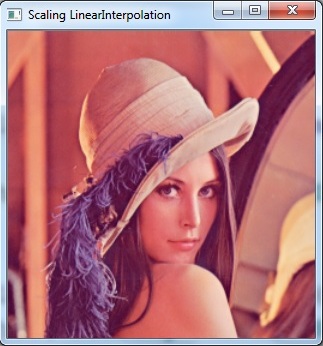
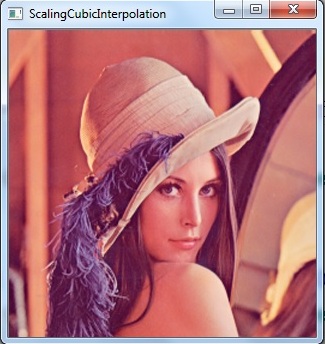
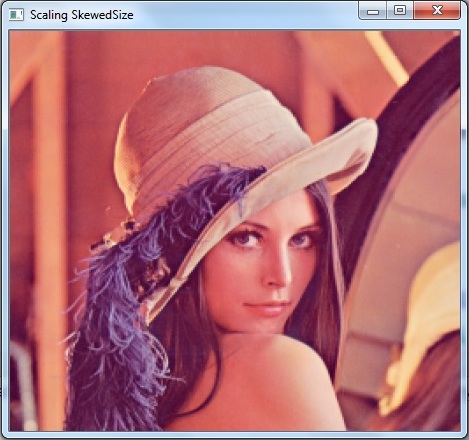
Subscribe and Get Free Video Courses & Articles in your Email