In this Python OpenCV article we are going to talk about Image Rotation in OpenCV, so we can
use cv2.rotate() function for rotating an image in OpenCV and there are three constant types of
rotation that you can pass as parameter in the function. so these are the constants.
- cv2.ROTATE_90_CLOCKWISE
- cv2.ROTATE_90_COUNTERCLOCKWISE
- cv2.ROTATE_180
Read Image Smoothing Techniques in OpenCV
1: OpenCV Averaging Image Blurring in Python
2: OpenCV Gaussian Blurring for Images in Python
3: OpenCV Median Blurring for Images in Python
4: OpenCV Smooth Image with Bilateral Filtering
So now this is the complete source code for OpenCV Image Rotation in Python
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17 18 19 20 21 22 23 24 25 26 27 28 29 30 31 32 33 34 35 |
import cv2 import matplotlib.pyplot as plt img = cv2.imread('lena.tif') #Our three rotation img_rotate_90_clockwise = cv2.rotate(img, cv2.ROTATE_90_CLOCKWISE) img_rotate_90_counterclockwise = cv2.rotate(img, cv2.ROTATE_90_COUNTERCLOCKWISE) img_rotate_180 = cv2.rotate(img, cv2.ROTATE_180) #converting images from bgr to rgb original_image = cv2.cvtColor(img, cv2.COLOR_BGR2RGB) img_clockwise = cv2.cvtColor(img_rotate_90_clockwise, cv2.COLOR_BGR2RGB) img_counterclockwise = cv2.cvtColor(img_rotate_90_counterclockwise, cv2.COLOR_BGR2RGB) img_180 = cv2.cvtColor(img_rotate_180, cv2.COLOR_BGR2RGB) titles = ["Original Image", "Image 90 Clockwise", "Image 90 CounterClockWise", "Image Rotate 180" ] images = [original_image, img_clockwise, img_counterclockwise,img_180 ] for i in range(4): plt.subplot(2,2, i+1) plt.imshow(images[i]) plt.title(titles[i]) plt.xticks([]), plt.yticks([]) plt.show() cv2.waitKey(0) cv2.destroyAllWindows() |
This line of code is used for reading of the image, make sure that you have added an image
in your working directory.
1 |
img = cv2.imread('lena.tif') |
So in here we have used cv2.rotate() function and we have added our three types of rotation.
1 2 3 |
img_rotate_90_clockwise = cv2.rotate(img, cv2.ROTATE_90_CLOCKWISE) img_rotate_90_counterclockwise = cv2.rotate(img, cv2.ROTATE_90_COUNTERCLOCKWISE) img_rotate_180 = cv2.rotate(img, cv2.ROTATE_180) |
Because we are going to show our images in Matplotlib, so Matplotlib uses RGB (Red, Green, Blue)
color system, and OpenCV uses BGR (Blue, Green, Red) color system, we need to convert the BGR
color to RGB. if we don’t do this there will be messed up in the color.
1 2 3 4 |
original_image = cv2.cvtColor(img, cv2.COLOR_BGR2RGB) img_clockwise = cv2.cvtColor(img_rotate_90_clockwise, cv2.COLOR_BGR2RGB) img_counterclockwise = cv2.cvtColor(img_rotate_90_counterclockwise, cv2.COLOR_BGR2RGB) img_180 = cv2.cvtColor(img_rotate_180, cv2.COLOR_BGR2RGB) |
Note: cv2.waitKey() is a keyboard binding function. Its argument is the time in milliseconds.
the function waits specified milliseconds for any keyboard event. If you press any key in that
time, the program continues. If 0 is passed, it waits indefinitely for a key stroke.
So now run the complete code and this will be the result.
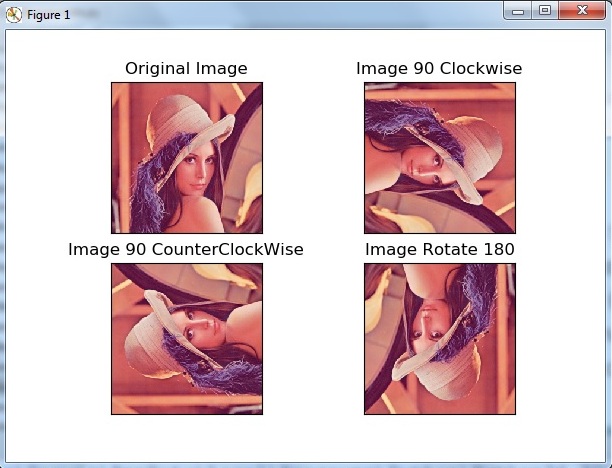
Subscribe and Get Free Video Courses & Articles in your Email