In this Java OOP tutorial we want to learn about Object Oriented Concepts in Java, so Java is an object oriented programming language, it means that it is designed around the concepts of objects, classes and inheritance. if we understand basic principles of Object Oriented Programming, than we can write clean and maintainable code, so now let’s talk about some basics of Java OOP.
-
Java Classes and Objects
In Java everything is an object, and objects are created from classes. class is a blueprint or a template that defines the properties and behaviors of an object. also an object is an instance of a class. this is the basic syntax for creating a class:
Basic Syntax for Creating a Class
1 2 3 4 5 6 7 8 9 10 11 12 13 14 |
class ClassName { // Attributes (fields) dataType attributeName; // Constructor ClassName(dataType parameter) { this.attributeName = parameter; } // Methods returnType methodName() { // Method body } } |
Creating and Using a Class and Object
Now let’s create a class named Person and an object from this class. now in here we want to create a Person class in Java.
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 |
class Person { // Attributes String name; int age; // Constructor Person(String name, int age) { this.name = name; this.age = age; } // Method to display person details void displayDetails() { System.out.println("Name: " + name + ", Age: " + age); } } |
Now let’s create object if Person class in Java OOP.
1 2 3 4 5 6 7 8 9 |
public class Main { public static void main(String[] args) { // Creating an object of the Person class Person person1 = new Person("Codeloop", 25); // Using the object to call the method person1.displayDetails(); } } |
If you run your code, this will be the result
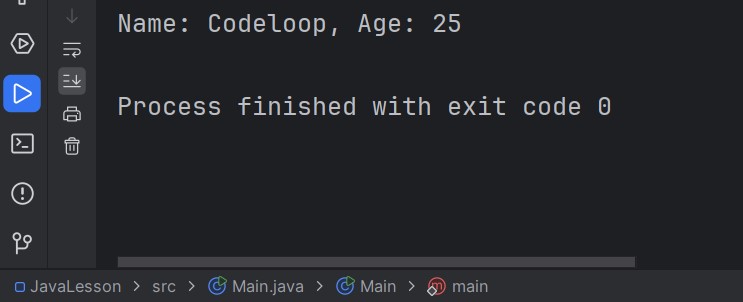
Java Class Code Description
- Class Definition: We have defined Person class with two attributes: name and age. also the class has a constructor to initialize these attributes and a method displayDetails to print the details of a person.
- Constructor: Our constructor Person(String name, int age) initializes the name and age attributes using the this keyword, which refers to the current object.
- Method: displayDetails method prints the name and age of the person.
- Creating an Object: In the Main class, we create an object of the Person class using the new keyword and call the displayDetails method on this object.
Key Points on Java Class
- Class: A blueprint that defines attributes and methods.
- Object: An instance of a class created using the new keyword.
- Constructor: Special method used to initialize objects.
- Method: Function defined within a class to perform a specific action.
-
Java Encapsulation
Encapsulation is the concept of hiding internal details of an object and exposing only the necessary functionalities via public interface. Using encapsulation you can protect the state of an object and prevent it from being accessed or modified by external entities. for creating encapsulation we can use access modifiers such as public, private and protected.
Basic Syntax for Java Encapsulation
For encapsulating data, you generally:
- Declare the class variables as private.
- Provide public getter and setter methods to access and update the private variables.
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17 18 19 20 21 22 23 24 25 26 27 28 29 30 31 32 33 34 35 36 37 38 39 40 |
class Person { // Private attributes private String name; private int age; // Constructor public Person(String name, int age) { this.name = name; this.age = age; } // Public getter for name public String getName() { return name; } // Public setter for name public void setName(String name) { this.name = name; } // Public getter for age public int getAge() { return age; } // Public setter for age public void setAge(int age) { if (age > 0) { this.age = age; } else { System.out.println("Age must be positive"); } } // Method to display person details public void displayDetails() { System.out.println("Name: " + name + ", Age: " + age); } } |
Using the Encapsulated Person Class
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 |
public class Main { public static void main(String[] args) { // Creating an object of Person class Person person1 = new Person("John", 25); // Accessing and modifying private attributes via public methods person1.setName("Jane"); person1.setAge(30); // Displaying details of the person person1.displayDetails(); // Attempt to set an invalid age person1.setAge(-5); } } |
Code Description
- Private Attributes: our Person class has private attributes of name and age. These attributes cannot be accessed directly from outside of class.
- Constructor: constructor Person initializes the name and age attributes.
- Getter and Setter Methods: Public getter and setter methods are provided for accessing and modifying the private attributes. These methods ensures that internal state is accessed and modified in controlled manner.
- getName and getAge methods return the values of name and age.
- setName method updates the name attribute.
- setAge method updates age attribute, but only if provided age is positive. This adds a layer of validation.
- Displaying Details: displayDetails method prints the name and age of the person.
Key Points on Java Encapsulation
- Access Modifiers: Use private for hiding attributes from external access, and public for methods that need to be accessible.
- Data Protection: Encapsulation protects the state of an object by providing controlled access through getter and setter methods.
- Validation: Using encapsulation you can add validation logic in setter methods to ensure the integrity of the data.
-
Java Inheritance
Using inheritance one class inherits the properties and behaviors of another class. for the class that is being inherited we can call superclass or parent class, and the class that inherits from, it is called subclass or child class. inheritance allows us to reuse code and create hierarchy of classes with increasing levels of abstraction.
Basic Syntax for Inheritance
For implementing inheritance in Java, you use extends keyword.
1 2 3 4 5 6 7 |
class Superclass { // Superclass properties and methods } class Subclass extends Superclass { // Subclass additional properties and methods } |
Let’s create an example demonstrating inheritance in Java.
Superclass: Animal
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17 18 19 20 |
// Superclass class Animal { // Properties String name; // Constructor public Animal(String name) { this.name = name; } // Method to display animal details public void displayDetails() { System.out.println("Animal Name: " + name); } // Method to make sound public void makeSound() { System.out.println(name + " makes a sound."); } } |
Subclass: Dog
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17 18 19 20 21 22 23 |
// Subclass class Dog extends Animal { // Additional property String breed; // Constructor public Dog(String name, String breed) { super(name); // Call superclass constructor this.breed = breed; } // Override method to make sound @Override public void makeSound() { System.out.println(name + " barks."); } // Method to display dog details @Override public void displayDetails() { System.out.println("Dog Name: " + name + ", Breed: " + breed); } } |
Using Animal and Dog Classes
1 2 3 4 5 6 7 8 9 10 11 12 13 |
public class Main { public static void main(String[] args) { // Create an Animal object Animal animal = new Animal("Generic Animal"); animal.displayDetails(); animal.makeSound(); // Create a Dog object Dog dog = new Dog("Buddy", "Golden Retriever"); dog.displayDetails(); dog.makeSound(); } } |
Code Description
- Superclass (Animal):
- Animal class has a property name, a constructor to initialize this property, and two methods: displayDetails and makeSound.
- makeSound method in Animal provides a general behavior for making a sound.
- Subclass (Dog):
- Dog class extends the Animal class, and it inherits its properties and methods.
- It has an additional property breed and a constructor that calls the superclass constructor using super(name) to initialize the name property.
- makeSound method is overridden to provide a specific implementation for dogs.
- displayDetails method is also overridden to include the breed information.
- Main Class:
- In the Main class, an Animal object and a Dog object are created.
- displayDetails and makeSound methods are called on both objects to demonstrate inheritance and method overriding.
Key Points on Java Inheritance
- Inheritance Syntax: We need to use extends keyword for creating subclass.
- Superclass and Subclass: superclass contains general properties and methods, and subclass can add specific properties and methods or override existing ones.
- Code Reuse: Using java inheritance we can reuse our code, and that is because subclasses inherits and build upon the code in the superclass.
- Method Overriding: Subclasses can provide specific implementations of methods that are defined in the superclass, allowing for polymorphic behavior.
-
Java Polymorphism
Using polymorphism an object can take many forms. in Java we can create polymorphism using method overriding and method overloading. method overriding is used when subclass provides its implementation of a method that is already defined in its superclass. method overloading is used when a class has two or more methods with the same name but different parameters.
Java Method Overriding
Using method overriding, we have specific implementation for a method that is already defined in its superclass. This is useful for defining behavior that is specific to the subclass.
Example of Method Overriding
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17 18 19 20 21 22 23 24 25 26 27 28 |
// Superclass class Animal { // Method to make sound public void makeSound() { System.out.println("The animal makes a sound"); } } // Subclass class Dog extends Animal { // Overriding makeSound method @Override public void makeSound() { System.out.println("The dog barks"); } } public class Main { public static void main(String[] args) { // Creating objects of Animal and Dog Animal myAnimal = new Animal(); Animal myDog = new Dog(); // Polymorphism in action // Calling makeSound method myAnimal.makeSound(); // Outputs: The animal makes a sound myDog.makeSound(); // Outputs: The dog barks } } |
Run the code and this will be the result
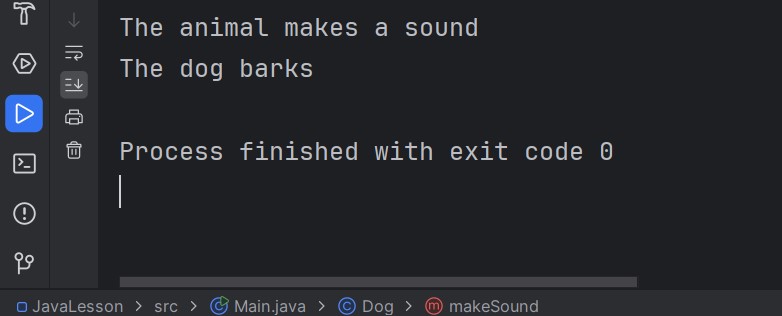
Code Description for Method Overriding
- Superclass (Animal): Animal class has a method makeSound that prints a generic message.
- Subclass (Dog): Dog class extends Animal and overrides the makeSound method to provide a specific implementation that prints a message for a dog.
- Main Class: In the Main class, we have created objects of Animal and Dog. When the makeSound method is called on the Dog object, the overridden method in the Dog class is executed, demonstrating polymorphism.
Java Method Overloading
Method overloading allows a class to have more than one method with the same name, but with different parameters. This is useful for providing multiple ways to perform a similar operation.
Example of Method Overloading
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17 18 19 20 21 22 23 24 25 26 27 |
class MathOperations { // Method to add two integers public int add(int a, int b) { return a + b; } // Overloaded method to add three integers public int add(int a, int b, int c) { return a + b + c; } // Overloaded method to add two double values public double add(double a, double b) { return a + b; } } public class Main { public static void main(String[] args) { MathOperations math = new MathOperations(); // Calling the overloaded add methods System.out.println("Sum of 2 and 3: " + math.add(2, 3)); // Outputs: Sum of 2 and 3: 5 System.out.println("Sum of 1, 2 and 3: " + math.add(1, 2, 3)); // Outputs: Sum of 1, 2 and 3: 6 System.out.println("Sum of 2.5 and 3.5: " + math.add(2.5, 3.5)); // Outputs: Sum of 2.5 and 3.5: 6.0 } } |
Method Overloading
- MathOperations Class: MathOperations class defines multiple add methods, each with different parameters.
- first add method takes two integers.
- second add method takes three integers.
- third add method takes two double values.
This will be the result
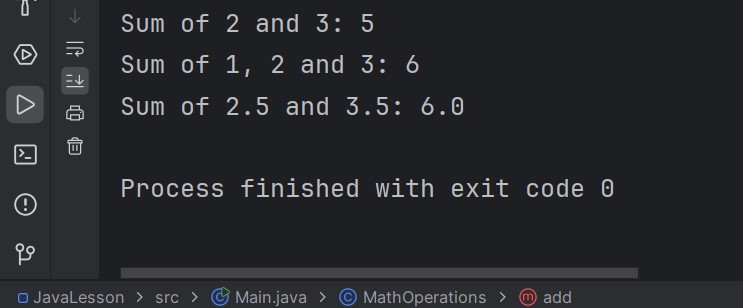
-
Java Abstraction
Abstraction is the process of identifying the essential features of an object while ignoring the non-essential details. Using that we can focus on what an object does rather than how it does it. In Java, abstraction can be achieved using abstract classes and interfaces.
- Abstract Class: An abstract class is a class that cannot be instantiated and can only be used as a superclass for its subclasses. It can have both abstract methods (without a body) and concrete methods (with a body).
- Interface: An interface is a collection of abstract methods and constants. A class that implements an interface must provide implementations for all the abstract methods defined in the interface.
Abstract Class Example
An abstract class can have abstract methods, and do not have a body, and concrete methods, which have a body. Subclasses of an abstract class must provide implementations for the abstract methods.
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17 18 19 20 21 22 23 24 25 26 27 28 |
// Abstract class abstract class Shape { // Abstract method abstract void draw(); // Concrete method public void display() { System.out.println("This is a shape."); } } // Subclass class Circle extends Shape { // Implementing the abstract method @Override void draw() { System.out.println("Drawing a circle."); } } public class Main { public static void main(String[] args) { // Shape shape = new Shape(); // This would give an error Shape circle = new Circle(); circle.display(); // Outputs: This is a shape. circle.draw(); // Outputs: Drawing a circle. } } |
This will be the result
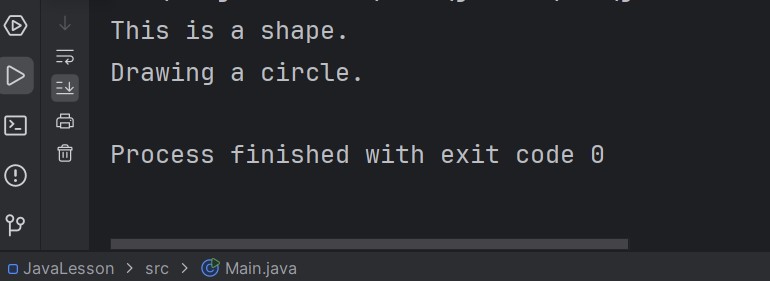
Java Interface
An interface defines a contract for what a class can do, without specifying how it does it. Classes that implement an interface must provide implementations for all its methods.
This is an example of Java Interface
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17 18 19 20 21 22 23 24 25 26 |
// Interface interface Animal { void makeSound(); void eat(); } // Class implementing the interface class Dog implements Animal { @Override public void makeSound() { System.out.println("The dog barks."); } @Override public void eat() { System.out.println("The dog eats."); } } public class Main { public static void main(String[] args) { Animal myDog = new Dog(); myDog.makeSound(); // Outputs: The dog barks. myDog.eat(); // Outputs: The dog eats. } } |
Subscribe and Get Free Video Courses & Articles in your Email