In this Numpy tutorial we want to learn about Numpy Array Indexing and Slicing, for Numpy we can say Numerical Python, so Numpy is a powerful library in Python, and it is used for scientific computing and data analysis tasks. the key feature that Numpy has is the ability of working with arrays. In this article we want to talk about Numpy array indexing and slicing. These are two essential techniques that allows us to access and manipulate data in the arrays.
Indexing Numpy Arrays
Indexing in Numpy refers to accessing individual elements inside an array. It allows us to fetch specific values or subsets of data. Numpy arrays are zero indexed, which means that the first element has an index of 0.
Now let’s check this example
1 2 3 4 5 6 7 8 |
import numpy as np # Create 1D array arr = np.array([10, 20, 30, 40, 50]) # Accessing individual elements print(arr[0]) print(arr[3]) |
In the above example, we have created 1D array and access elements by providing the desired index in the square brackets. Numpy also allows negative indexing, where -1 represents the last element, -2 represents the second last element, and so on.
This will be the output
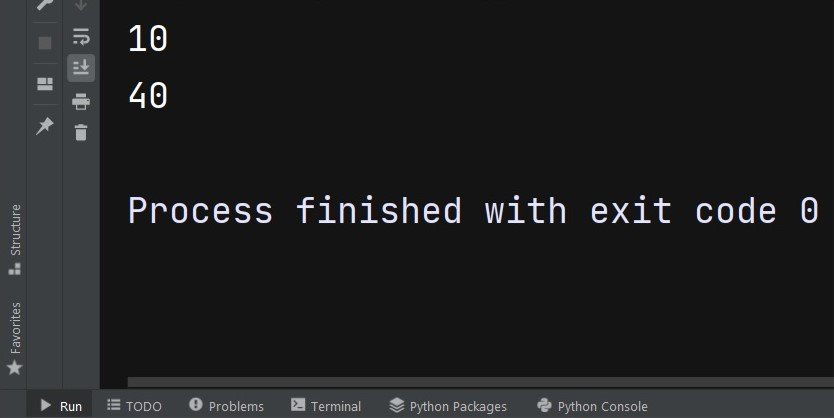
Slicing Numpy Arrays
Slicing goes beyond accessing individual elements and enables us to extract specific portions of an array. Slicing syntax involves specifying the start, stop and step parameters inside square brackets.
Let’s take a look at a practical example
1 2 3 4 5 6 7 8 9 10 |
import numpy as np # Create 1D array arr = np.array([10, 20, 30, 40, 50]) # Slice the array print(arr[1:4]) print(arr[2:]) print(arr[:3]) print(arr[::2]) |
In the above example, we have created 1D array and demonstrate different slicing techniques. The first slice [1:4] extracts elements starting from index 1 up to, but not including, index 4. The second slice [2:] extracts elements from index 2 until the end of the array. The third slice [:3] extracts elements from the beginning of the array up to, but not including, index 3. Lastly, the slice [::2] extracts elements at every second index.
This will be the output
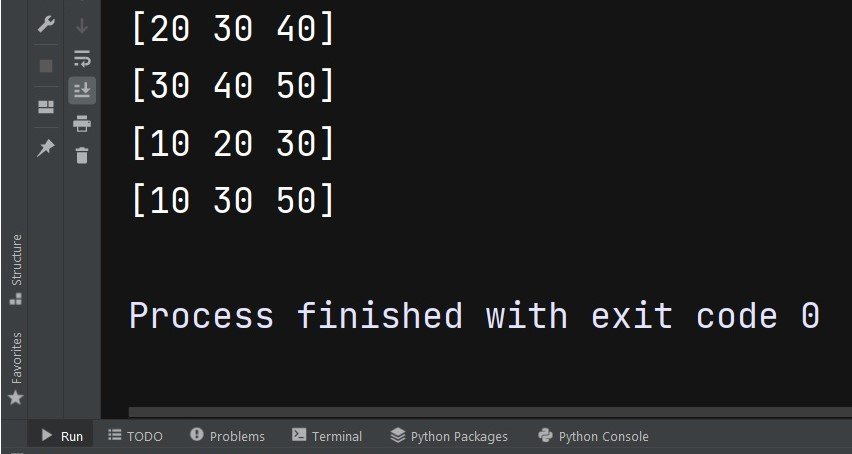
Numpy Indexing and Slicing Multidimensional Arrays
Numpy arrays can have multiple dimensions, and indexing and slicing become even more powerful when dealing with such arrays.
1 2 3 4 5 6 7 8 |
import numpy as np # Create 2D array arr = np.array([[1, 2, 3], [4, 5, 6], [7, 8, 9]]) # Accessing elements print(arr[0, 1]) print(arr[1:, :2]) |
In the above example, we create a 2D array and demonstrate how to access elements using row and column indices. The expression arr[0, 1] accesses the element at row index 0 and column index 1. The slice [1:, :2] selects the rows starting from index 1 and the columns up to, but not including, index 2.
Run the code and this will the output
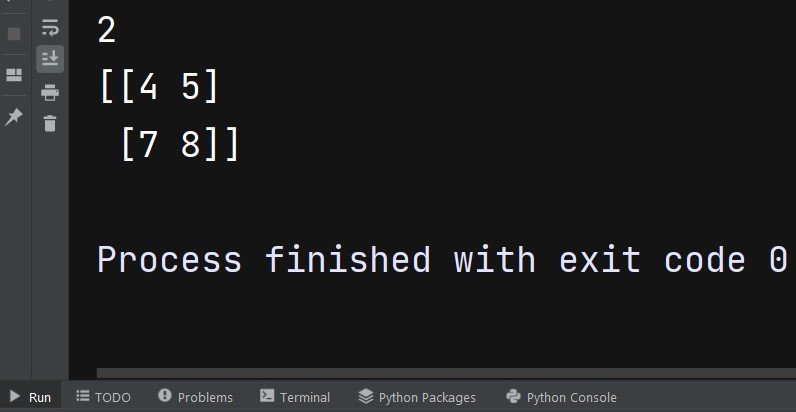
Learn More on Python Numpy
- Python Numpy for Machine Learning
- How to Install Numpy
- Working with Linear Algebra in Numpy
- Numpy vs Pandas
- Advance Python Numpy Techniques
Subscribe and Get Free Video Courses & Articles in your Email