This is our third tutorial in Java GUI, in this tutorial we are going to learn bout JavaFX Shape
Drawing Line. so drawing different shapes, especially lines are so easy in JavaFX. When drawing
on the JavaFX scene graph, lines are rendered using the screen coordinate space (system).
In geometry, a line is defined as a segment connected by two points in space, although it lacks
a width (thickness) and color value.
Also you can check Python GUI Development Tutorials in the below link.
1: PyQt5 GUI Development Tutorials
2: TKinter GUI Development Tutorials
3: Pyside2 GUI Development Tutorials
4: Kivy GUI Development Tutorials
5: wxPython GUI Development Tutorials
You can draw line by creating the object of the Line class in the JavaFX. and you need to give
the start and end position for the x and y.
1 |
Line redLine = new Line(10, 10, 200, 10); |
Also you can set the properties like this.
1 2 3 4 |
line.setStartX(100); line.setStartY(10); line.setEndX(10); line.setEndY(100); |
So now this is the complete code for Java GUI – JavaFX Shape Drawing Line.
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17 18 19 20 21 22 23 24 25 26 27 28 29 30 31 32 33 34 35 36 37 38 39 40 41 42 43 44 45 46 |
import javafx.application.Application; import javafx.scene.Group; import javafx.scene.Scene; import javafx.scene.paint.Color; import javafx.scene.shape.Line; import javafx.scene.shape.StrokeLineCap; import javafx.stage.Stage; public class DrawingLines extends Application { public static void main(String[] args) { launch(args); } @Override public void start(Stage stage) { stage.setTitle("Drawing Lines In JavaFx"); Group root = new Group(); Scene scene = new Scene(root, 400, 400, Color.GREEN); // Common Line Line line = new Line(100,10,10,100); //line.setStartX(100); //line.setStartY(10); //line.setEndX(10); //line.setEndY(100); line.setStroke(Color.RED); line.setStrokeWidth(10); line.setStrokeLineCap(StrokeLineCap.ROUND); // DashedLine line.getStrokeDashArray().addAll(10d, 5d,15d,20d); line.setStrokeDashOffset(0); root.getChildren().add(line); stage.setScene(scene); stage.show(); } } |
Every JavaFX application should have a container, a container is like layout. as we
have created a Group container in the above code. but you can use different containers
from JavaFX.
1 |
Group root = new Group(); |
Also for every JavaFX application we need to create a Scene object. in the scene we need
to add our container with the width and height of the window, if you want to colorize your
window, you can do it in here. you can see that we have given 400 width and 400 height for the
window, also a green color for the window.
1 |
Scene scene = new Scene(root, 400, 400, Color.GREEN); |
This is for creating line , also we have used some properties for the line like given the stroke
color for line, the width of stroke and the style of the stroke.
1 2 3 4 5 6 |
Line line = new Line(100,10,10,100); line.setStroke(Color.RED); line.setStrokeWidth(10); line.setStrokeLineCap(StrokeLineCap.ROUND); |
Using this code you can create dashed line.
1 2 3 4 |
// DashedLine line.getStrokeDashArray().addAll(10d, 5d,15d,20d); line.setStrokeDashOffset(0); |
When you create a widget in JavaFX, for example button,label,combobox or some other
widgets, you need to add that to your container, as we have added our this button in the
Group container.
1 |
root.getChildren().add(line); |
At the end you need to set your scene object to the stage of the window, and show the window.
1 2 |
stage.setScene(scene); stage.show(); |
Run the complete code and this will be the result.
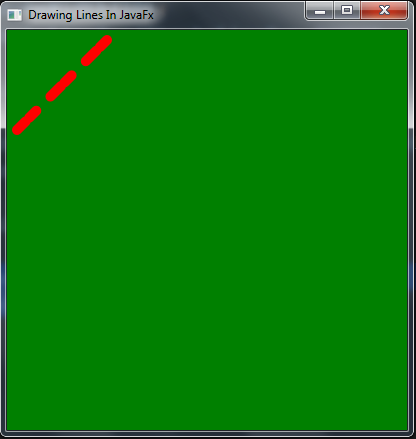
Subscribe and Get Free Video Courses & Articles in your Email
Comments are closed.