In this PyQt5 article iam going to have Introduction To PyQt5 QLinearGradient Color
also we are going to make an example for the QLinearGradient Color in PyQt5, there are three different kinds of Gradient Color in PyQt5, we have QLinearGradient, QRadialGradient and QConicalGradient. so first of all what is QLinearGradient ?
What is PyQt5 QLinearGradient?
According to Qt Documentation QLinearGradient class is used in combination with QBrush to specify a linear gradient brush. Linear gradients interpolate colors between start and end points. Outside these points the gradient is either padded, reflected or repeated depending on the currently set spread method.
Frst of all you can check my some articles on QPainter
1: PyQt5 Drawing Rectangle With QPainter Class
2: PyQt5 Drawing Ellipse With QPainter Class
3: PyQt5 Drawing Polygon With QPainter Class
So now this is the complete source code for Introduction To PyQt5 QLinearGradient Color
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17 18 19 20 21 22 23 24 25 26 27 28 29 30 31 32 33 34 35 36 37 38 39 40 41 42 43 44 45 46 47 48 49 50 51 52 53 54 55 56 57 58 59 |
from PyQt5 import QtGui from PyQt5.QtWidgets import QApplication, QMainWindow import sys from PyQt5.QtGui import QPainter, QBrush, QPen, QLinearGradient from PyQt5.QtCore import Qt class Window(QMainWindow): def __init__(self): super().__init__() # Window properties self.title = "Codeloop - PyQt5 Window" self.top = 200 self.left = 500 self.width = 400 self.height = 300 # Initialize the window self.InitWindow() def InitWindow(self): # Set window icon self.setWindowIcon(QtGui.QIcon("codeloop.png")) # Set window title and geometry self.setWindowTitle(self.title) self.setGeometry(self.left, self.top, self.width, self.height) # Show the window self.show() def paintEvent(self, e): # Define a QPainter object for painting painter = QPainter(self) # Set pen properties painter.setPen(QPen(Qt.black, 4, Qt.SolidLine)) # Create a linear gradient for filling grad1 = QLinearGradient(25, 100, 150, 175) # Set gradient colors grad1.setColorAt(0.0, Qt.darkGray) grad1.setColorAt(0.5, Qt.green) grad1.setColorAt(1.0, Qt.yellow) # Set brush with the gradient painter.setBrush(QBrush(grad1)) # Draw a rectangle with the gradient brush painter.drawRect(10, 10, 200, 200) # Create the application instance App = QApplication(sys.argv) # Create the main window window = Window() # Execute the application sys.exit(App.exec()) |
So this is the important line of code in QPainter class, when you want to draw you need create a built in method of def paintEvent(), the purpose of this method is for drawing shapes, so first we have created the object of QPainter class and after that we have created the QLinearGradient object and we set the color position for the Linear Gradient at the end we have drawn our rectangle.
1 2 3 4 5 6 7 8 9 10 11 12 |
def paintEvent(self, e): painter = QPainter(self) painter.setPen(QPen(Qt.black, 4, Qt.SolidLine)) grad1 = QLinearGradient(25, 100, 150, 175) grad1.setColorAt(0.0, Qt.darkGray) grad1.setColorAt(0.5, Qt.green) grad1.setColorAt(1.0, Qt.yellow) painter.setBrush(QBrush(grad1)) painter.drawRect(10, 10, 200, 200) |
Run the complete code and this will be the result.
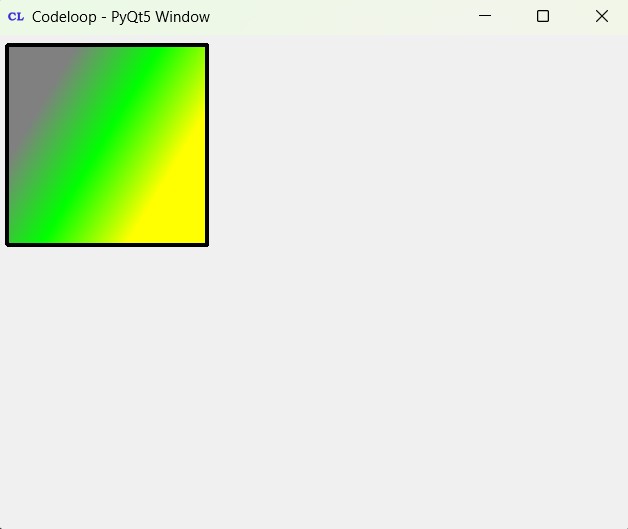
FAQs:
What is PyQt5 used for?
PyQt5 is Python binding for Qt framework. It is used to develop cross-platform desktop applications with rich graphical user interfaces (GUIs). PyQt5 allows you to create applications with features such as windows, buttons, menus, dialog boxes and many more, using Python programming language.
How to use Qt Designer in Python?
Qt Designer is a graphical user interface design tool provided by Qt for creating and designing GUIs. For using Qt Designer with PyQt5 in Python, follow these steps:
- Install PyQt5 and Qt Designer (PyQt5-Tools).
- Create your GUI design using Qt Designer and save the .ui file.
- Convert the .ui file to Python module using pyuic5 command-line tool.
- Import the generated Python module into your Python application and use it to create the GUI.
How to create a GUI with PyQt5?
For creating a GUI with PyQt5, follow these basic steps:
- Import necessary modules from PyQt5.
- Create a subclass of QMainWindow or QWidget to represent your main window or widget.
- Define layout and components of your GUI (buttons, labels, etc.) in the subclass’s constructor method.
- Set up event handlers or signals and slots to handle user interactions.
- Instantiate your subclass, set any additional properties, and show the GUI using the show() method.
Subscribe and Get Free Video Courses & Articles in your Email