In this article we are going to have Introduction to Java Object Oriented Programming, but first of all let’s talk about OOP in Java.
What is Java Object Oriented Programming?
So Object-oriented programming (OOP) is one of the bigger programming buzzwords of recent years, and you can spend years learning all about object-oriented programming methodologies and how they can make your life easier than The Old Way of programming. It all comes down to organizing your programs in ways that echo how things are put together in the real world.
Key Concept on Java Objected Oriented Programming
These are an overview of key concepts in Java OOP:
- Objects: Objects are instances of classes. They encapsulate data and behavior related to a specific entity. For example, a Person class can represent a person and contain attributes like name, age, and methods like eat(), sleep(), etc.
- Classes: Classes are blueprints for creating objects. They define the structure (attributes) and behavior (methods) of objects. In Java, classes are defined using the class keyword.
- Encapsulation: Encapsulation is the bundling of data (attributes) and methods that operate on the data inside a single unit (class). It helps in hiding the internal state of an object and only exposing necessary functionalities. Access modifiers like public, private, and protected are used to control access to the members of a class.
- Inheritance: Inheritance is the mechanism by which one class (subclass or derived class) can inherit properties and behavior from another class (superclass or base class). It promotes code reusability and establishes a hierarchical relationship between classes. In Java, inheritance is achieved using the extends keyword.
- Polymorphism: Polymorphism allows objects of different classes to be treated as objects of a common superclass. It enables method overriding and method overloading. Method overriding occurs when a subclass provides a specific implementation of a method that is already defined in its superclass. Method overloading occurs when a class has multiple methods with the same name but different parameter lists.
- Abstraction: Abstraction is the process of hiding the implementation details and showing only the essential features of an object. It helps in managing complexity by providing a simplified view of objects. Abstract classes and interfaces are used to achieve abstraction in Java.
Now let’s talk more about these concepts
Java Objects and Classes
Object-oriented programming models how objects in the real world are often composed of many smaller objects. However, the ability to combine objects is just one aspect of object-oriented programming. Object-oriented programming encompasses several other concepts and features aimed at simplifying the creation and usage of objects. Among these features, classes are the most important.
A class serves as a template for creating multiple objects with similar characteristics. It embodies all the attributes and behaviors shared by a specific group of objects. When programming in an object-oriented language, you define classes rather than actual objects. Classes define the blueprint for objects.
Java Behavior and Attributes
Every class you write in Java is generally made up of two components: attributes and behavior. In this section, you’ll learn about each one as it applies to a theoretical class called Motorcycle. To finish up this section, you’ll create the Java code to implement a representation of a motorcycle.
Java Attributes
Attributes are the individual things that differentiate one object from another and determine the appearance, state, or other qualities of that object. Let’s create a theoretical class called Motorcycle. The attributes of a motorcycle might include the following:
- Color: red, green, silver, brown
- Style: cruiser, sport bike, standard
- Make: Honda, BMW, Bultaco
Attributes of an object can also include information about its state; for example, you could have features for engine condition (off or on) or current gear selected.
Attributes are defined by variables; in fact, you can consider them analogous to global variables for the entire object. Because each instance of a class can have different values for its variables, each variable is instance variable.
Java Instance Variable
Instance variables define the attributes of an object. The class defines the kind of attribute, and each instance stores its own value for that attribute.
Java Behavior
A class’s behavior determines what instances of that class do when their internal state changes
or when that instance is asked to do something by another class or object. Behavior is the way
objects can do anything to themselves or have anything done to them. For example, to go back
to the theoretical Motorcycle class, here are some behaviors that the Motorcycle class might have:
- Start the engine
- Stop the engine
- Speed up
- Change gear
- Stall
How To Create Class In Java ?
Now let’s create our class in Java
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17 18 19 20 21 |
// Define the Motorcycle class public class Motorcycle { // Define attributes for the Motorcycle class String make; // Attribute to store the make of the motorcycle String color; // Attribute to store the color of the motorcycle boolean engineState; // Attribute to store the state of the engine (on/off) // Method to start the engine of the motorcycle void startEngine() { // Check if the engine is already on if (engineState == true) { System.out.println("The engine is already on."); } // If the engine is off, start it else { engineState = true; // Set the engine state to on System.out.println("The engine is now on."); } } } |
So in the above code we have created a Motorcycle class. this class has attributes and behaviors, let me complete the code and run the code for the class. Also i have add a new method in this class.
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17 18 19 20 21 22 23 24 25 26 27 28 29 30 31 32 33 34 35 36 37 38 39 40 41 42 43 44 45 |
// Define the Motorcycle class public class Motorcycle { // Define attributes for the Motorcycle class String make; // Attribute to store the make of the motorcycle String color; // Attribute to store the color of the motorcycle boolean engineState; // Attribute to store the state of the engine (on/off) // Method to start the engine of the motorcycle void startEngine() { // Check if the engine is already on if (engineState == true) { System.out.println("The engine is already on."); } // If the engine is off, start it else { engineState = true; // Set the engine state to on System.out.println("The engine is now on."); } } // Method to display the attributes of the motorcycle void showAtts() { System.out.println("This motorcycle is a " + color + " " + make); // Check the engine state and print whether it's on or off if (engineState == true) { System.out.println("The engine is on."); } else { System.out.println("The engine is off."); } } // Main method to create an instance of Motorcycle public static void main(String[] args) { // Create an instance of Motorcycle Motorcycle m = new Motorcycle(); // Set the attributes of the motorcycle m.make = "Yamaha"; m.color = "Yellow"; // Display the attributes of the motorcycle m.showAtts(); // Start the engine of the motorcycle m.startEngine(); } } |
Run the code and this will be the result
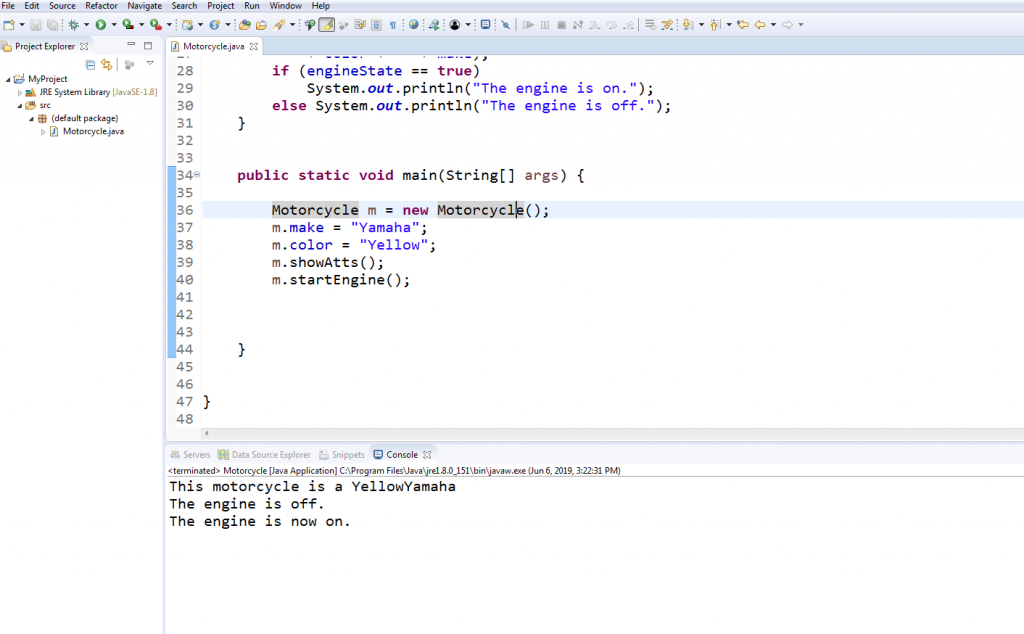
Subscribe and Get Free Video Courses & Articles in your Email