In this Python TKinter article we want to talk about How to Work with Images in Python Tkinter, so Python Tkinter is powerful and popular GUI library for creating graphical user interfaces (GUIs). when you are building GUI in TKinter, than some times you will need to work with images, in this article we want to explore different aspects of working with images in Python Tkinter.
Displaying Images in Python TKinter
For displaying an image in a Tkinter window, you need to follow some steps. first of all make sure you have installed PIL (Python Imaging Library) module, because it provides the necessary functionality for image handling. after that we need to load the image using the PIL Image.open() function. once you have the image object, you can use the Tkinter PhotoImage class to create an image widget. and lastly place the widget in your Tkinter window using a layout manager like pack() or grid(). this is an example:
1 2 3 4 5 6 7 8 9 10 11 12 |
from PIL import Image, ImageTk import tkinter as tk window = tk.Tk() image = Image.open("python.png") photo = ImageTk.PhotoImage(image) label = tk.Label(window, image=photo) label.pack() window.mainloop() |
Run your code and this will be the output.
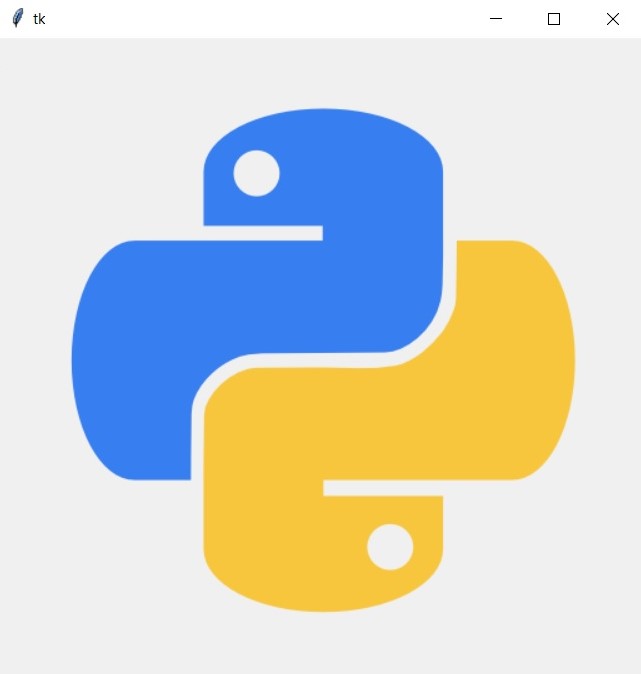
Resizing Images in Python TKinter
In some cases, you may need to resize images to fit them into specific regions of your GUI. Tkinter provides an easy way to resize images using the Image class from the PIL module. you can use the resize() method to adjust the dimensions while maintaining the aspect ratio.
1 2 3 4 5 6 7 8 9 10 11 12 13 |
from PIL import Image, ImageTk import tkinter as tk window = tk.Tk() image = Image.open("python.png") resized_image = image.resize((200, 200)) # Adjust dimensions as needed photo = ImageTk.PhotoImage(resized_image) label = tk.Label(window, image=photo) label.pack() window.mainloop() |
Adding Image Buttons
You can create interactive image buttons by combining the Button widget with an image. this process is similar to displaying images, but instead of using a Label, you use a Button widget. Tkinter allows you to bind a function to the button’s click event, enabling you to perform actions when the button is pressed.
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 |
from PIL import Image, ImageTk import tkinter as tk def button_click(): # Add your button click logic here pass window = tk.Tk() image = Image.open("python.png") photo = ImageTk.PhotoImage(image) button = tk.Button(window, image=photo, command=button_click) button.pack() window.mainloop() |
More on Python GUI Development
- Kivy GUI Development Tutorials
- TKinter GUI Development Tutorials
- Pyside2 GUI Development
- wxPython GUI Development Tutorials
- PyQt5 GUI Development Tutorials
Subscribe and Get Free Video Courses & Articles in your Email